Understanding Python Operators with Example Programs
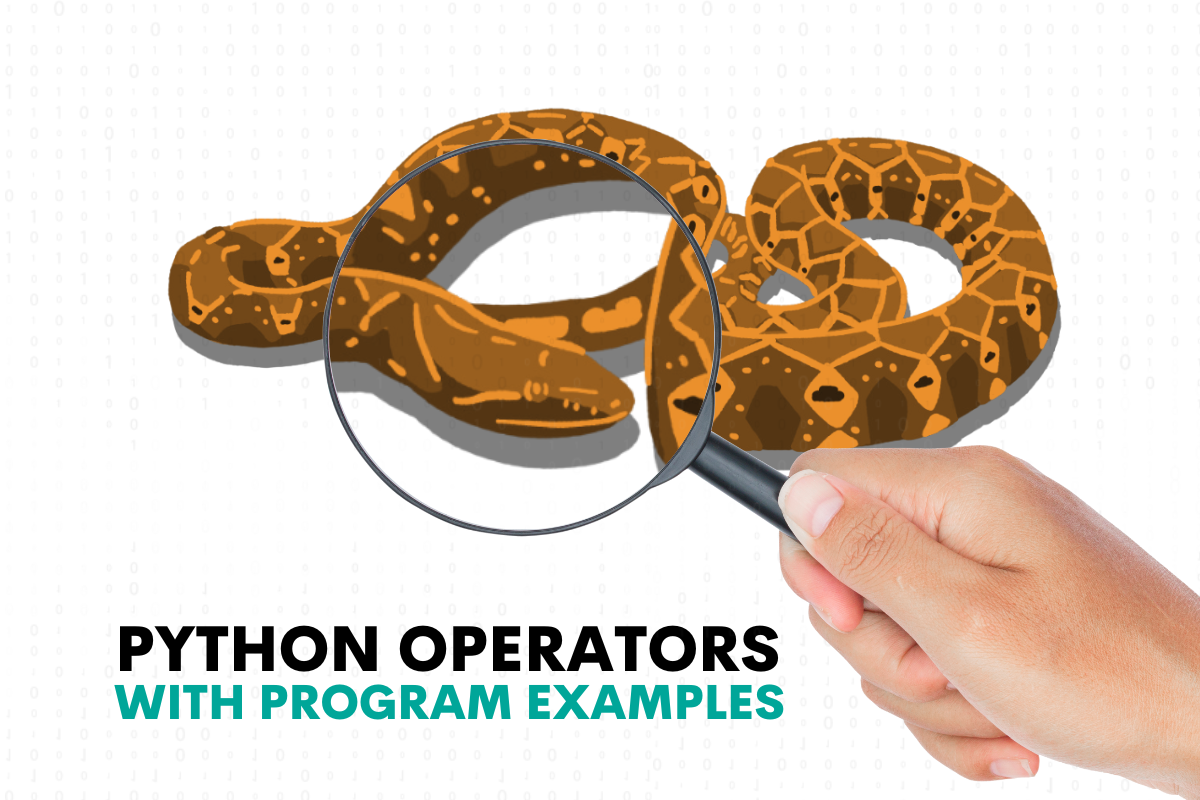
Categories:
- Written by:
Nathan Rosidi
The mastery of Python operators will serve as a strong foundation for solving complex challenges with confidence and ease.
There is no doubt that Python is one of the most popular programming languages when it comes to applying Data Science tasks.
And one of the key features that make Python an excellent choice for various tasks is its comprehensive set of operators.
What are Python Operators in a Nutshell?
Python operators are special symbols that perform certain operations on values or variables, like arithmetic operators(+), comparison operators(==), logical operators(and), membership operators(in), assignment operators(+=), bitwise operators(or), identity operators(is) and ternary operators.
How Python Operators Are Useful for Programming in Python?
Python operators allow data manipulation, conditional decision-making, and a number of other tasks needed for the development of great applications in Python.
In this article, we will cover the following Python operators:
- Arithmetic Operators
- Comparison Operators
- Logical Operators
- Membership Operators
- Assignment Operators
- Bitwise Operators
- Identity Operators
- Ternary Operators
By understanding the potential uses of these Python operators, you will be better equipped to write efficient and concise Python code.
If you are eager to read more Advanced Python Concepts, check out our post “A Comprehensive Guide to Advanced Python Concepts”.
Now let’s dig deeper into Python Operators.
Python Operators
Programming languages use symbols called operators to carry out specific manipulations on values or variables, as we said earlier too.
Data manipulation and condition-based decision-making are both can be done in Python by using these.
We will cover different examples of them in this article.
The Python operators that we will cover for this article are arithmetic operators, comparison operators, logical operators, membership operators, assignment operators, bitwise operators, identity operators, and ternary operators.
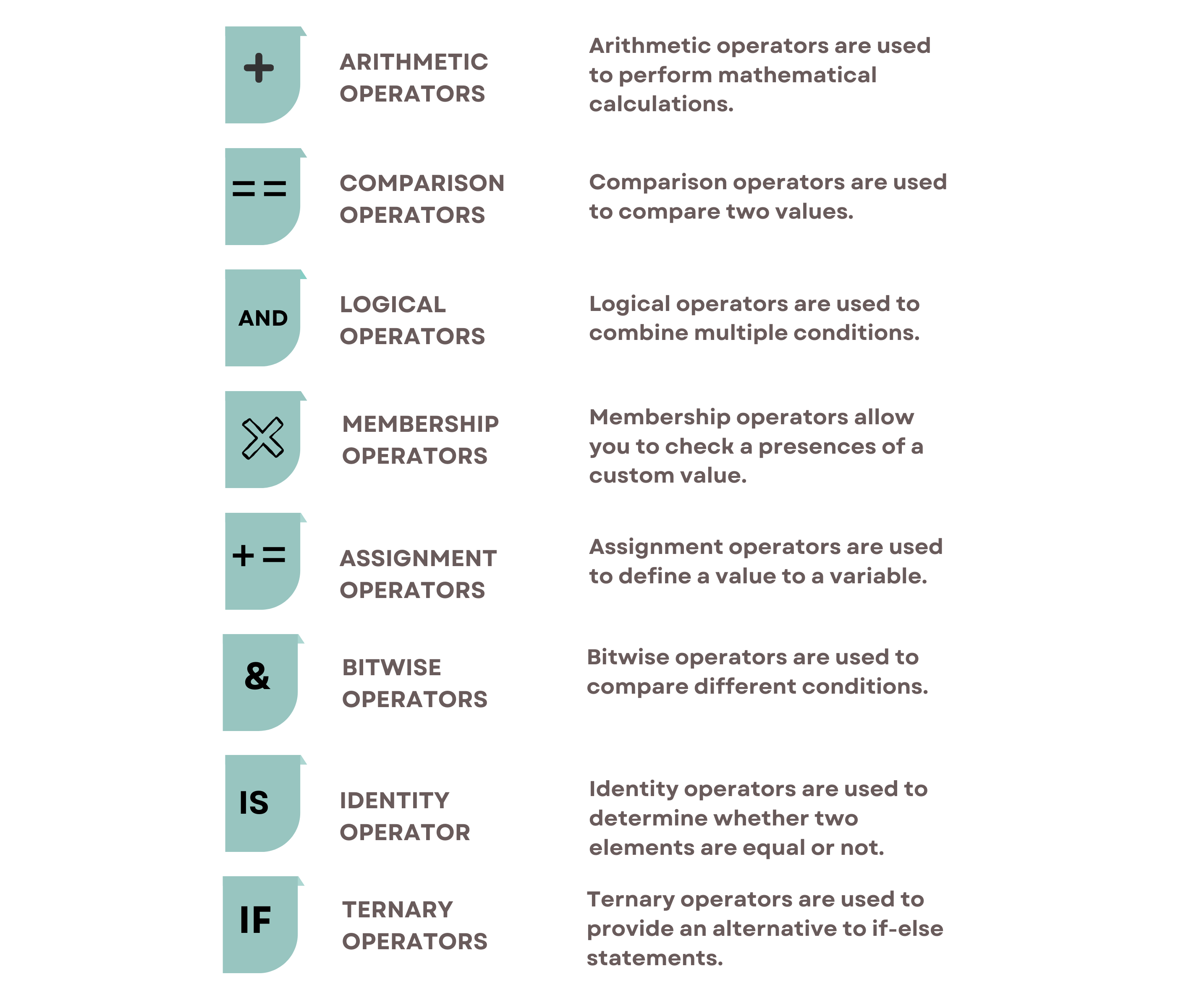
Let’s start with the Arithmetic operators.
Python Arithmetic Operators
Python offers arithmetic operators for executing mathematical operations on variables, numbers, or expressions.
They enable calculations such as addition, subtraction, multiplication, division, modulus, exponentiation, and floor division.
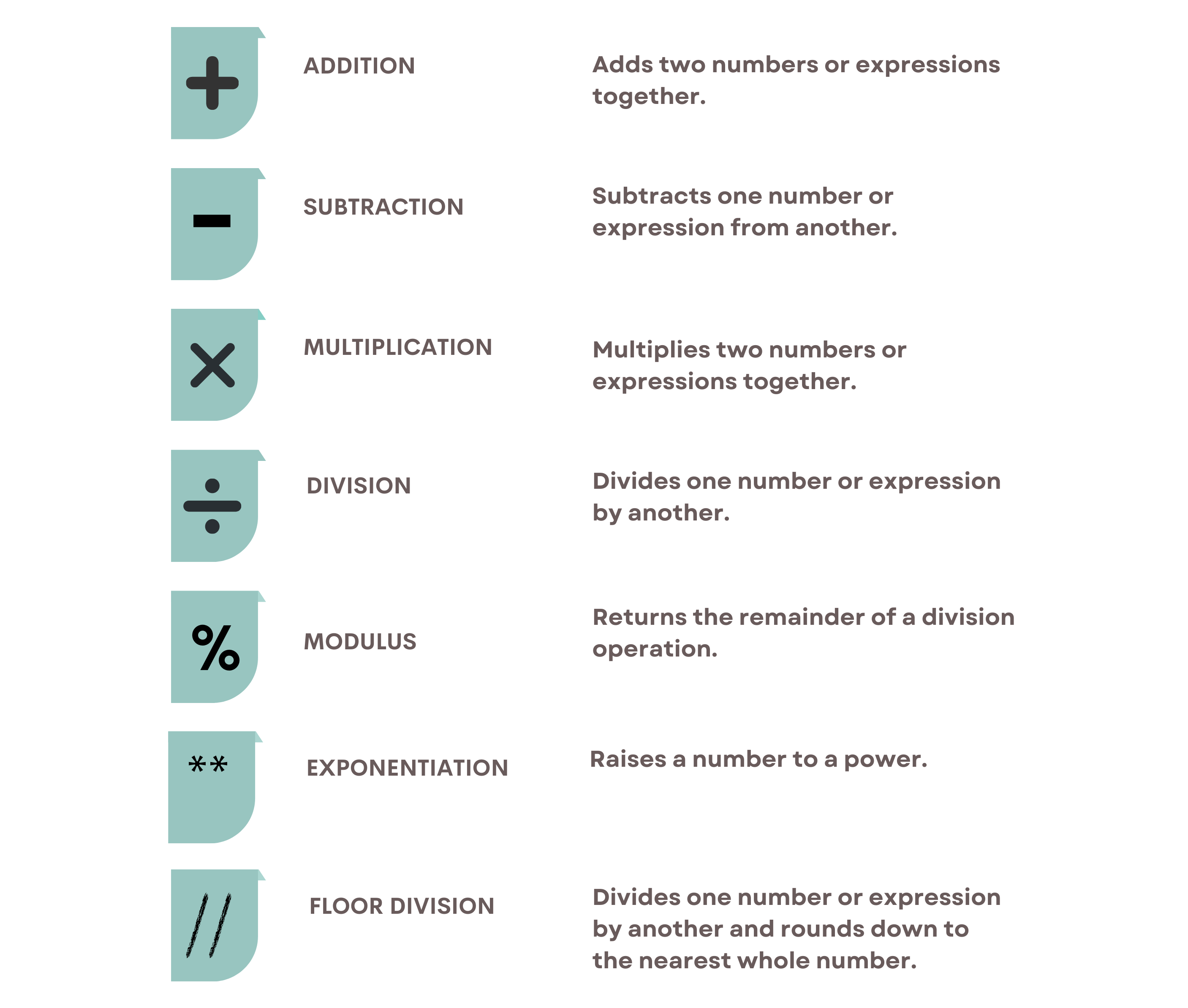
Now let’s see how to implement these in Python.
# Addition
x = 3 + 4 # x is 7
# Subtraction
y = 8 - 5 # y is 3
# Multiplication
z = 2 * 6 # z is 12
# Division
a = 10 / 2 # a is 5.0
# Modulo
b = 10 % 3 # b is 1
# Exponentiation
c = 2 ** 3 # c is 8
# Floor Division
d = 10 // 3 # d is 3
# Exponentiation
e = 2 ** 3 # d is 8
Python Comparison Operators
Comparison operators are used to compare the values of two variables and identify their relationship.
These python operators enable conditional decision-making in programs by returning a boolean value (True or False) based on the comparison.
They can do tests, for equality, inequality, greater than, less than, greater than or equal to, and less than or equal to.
There are several comparison operators available in Python.
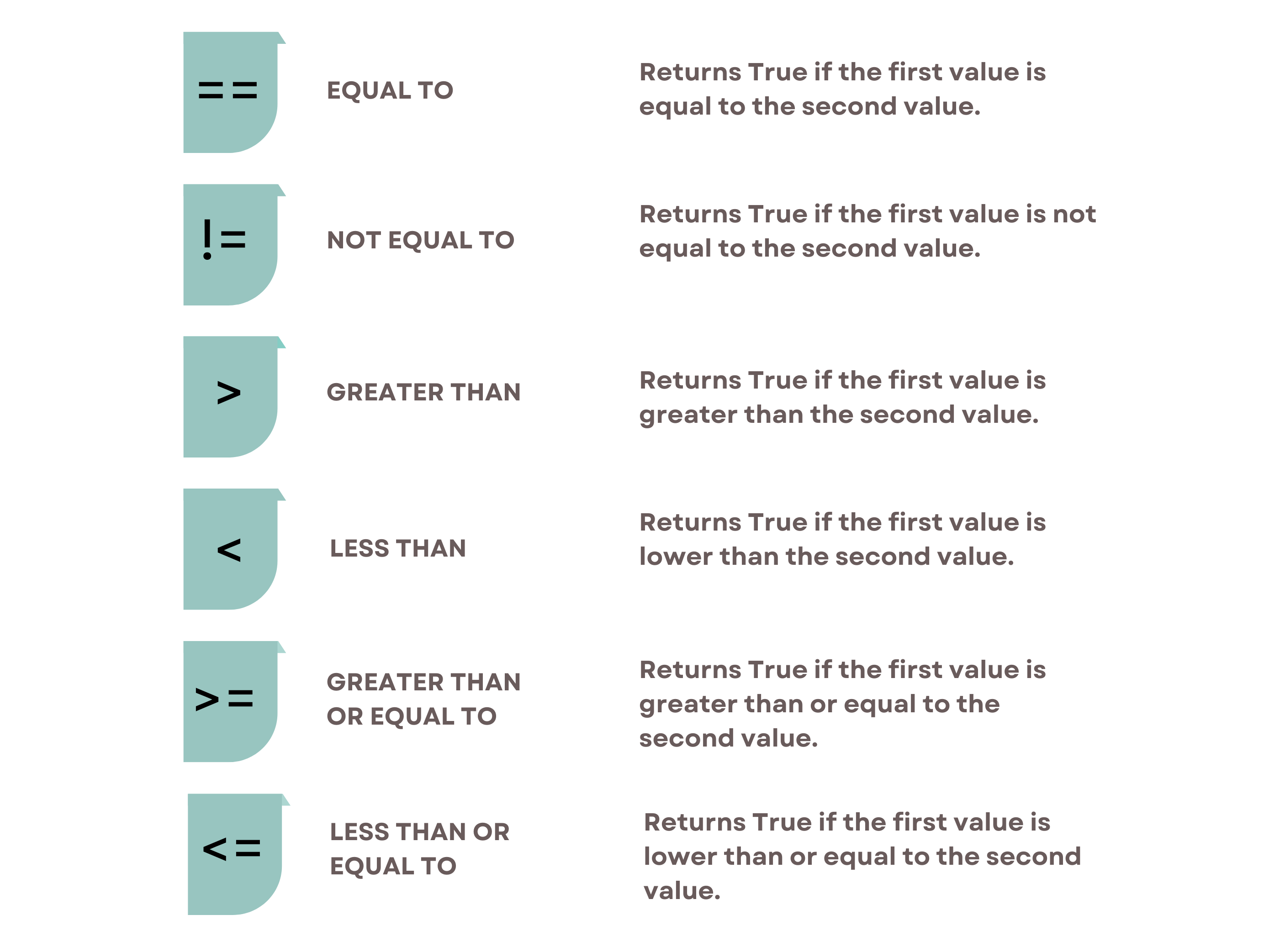
These comparison operators can be used to compare variables, constants, or even results of expressions, and the result of the comparison will always be a boolean value, either True or False.
Let’s see the codes.
# Equal to
x = 3 == 3 # x is True
# Not equal to
y = 4 != 2 # y is True
# Greater than
z = 5 > 2 # z is True
# Less than
a = 7 < 9 # a is True
# Greater than or equal to
b = 6 >= 6 # b is True
# Less than or equal to
c = 2 <= 3 # c is True
Python Logical Operator
In conditional statements, Python logical operators are used to combine multiple conditions and make conclusions depending on the truth value of the conditions.
They are required for integrating numerous conditions in control structures such as if statements and while loops. AND, OR, and NOT are the three fundamental logical operators.
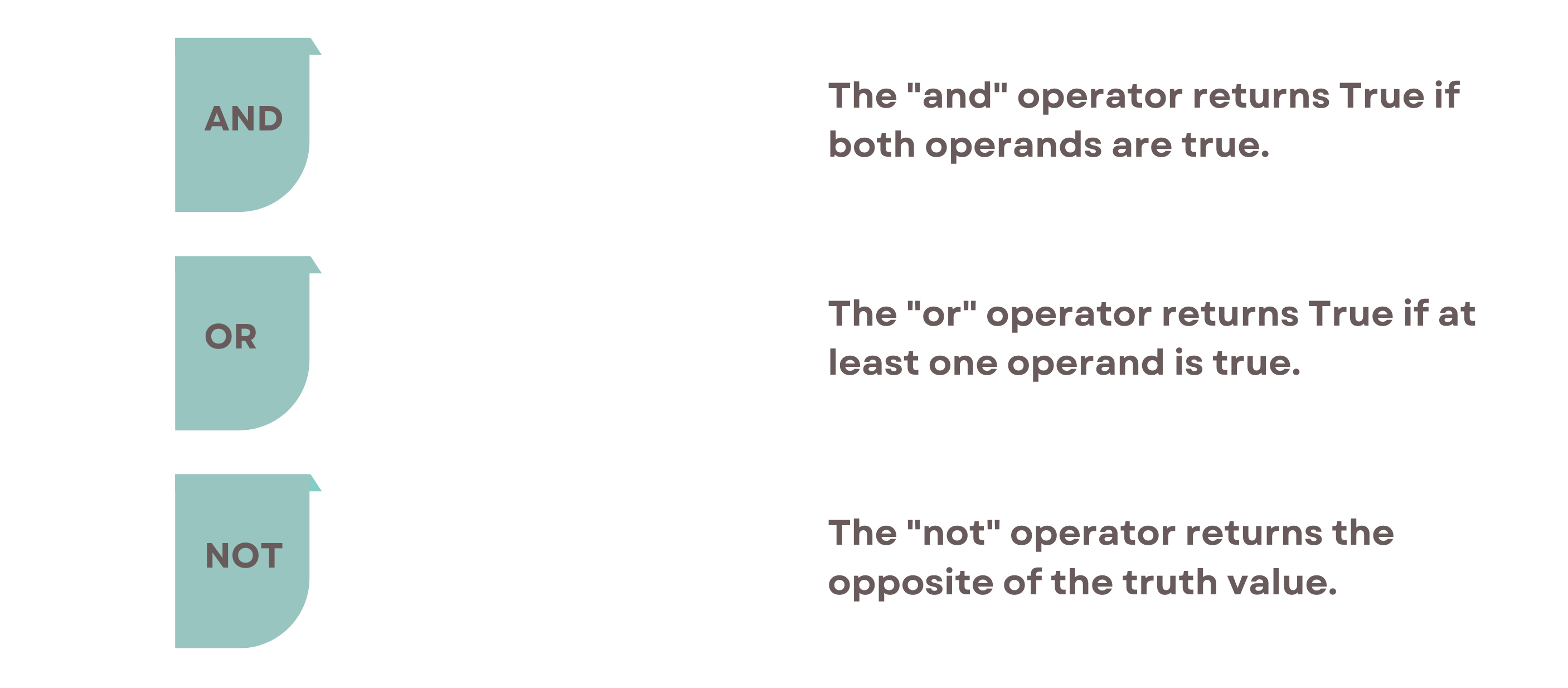
The "and" operator returns True if and only if both of its operands are True. In the example, (3 > 2) is True and (4 < 5) is also True, so the expression (3 > 2) and (4 < 5) evaluates to True and x is assigned the value True.
The "or" operator returns True if either one of its operands is True. In the example, (2 == 2) is True and (3 != 3) is False, so the expression (2 == 2) or (3 != 3) evaluates to True and y is assigned the value True.
The "not" operator negates a boolean expression. It returns the opposite of the boolean value of its operand. In the example, (2 < 1) is False, so not (2 < 1) returns True and z is assigned the value True.
Now, let’s see the code.
# and
x = (3 > 2) and (4 < 5) # x is True
# or
y = (2 == 2) or (3 != 3) # y is True
# not
z = not (2 < 1) # z is True
In summary, logical operators are used to make decisions based on the truth value of multiple conditions and are an essential part of programming in Python.
Python Membership Operator
Membership operators identify whether a variable is a member of a sequence, such as strings, lists, or tuples. or not.
They are helpful to find out if a variable in a data structure exists or not. If the value provided is found in the series, the 'in' operator returns True, and if it is not, the 'not in' operator returns True.
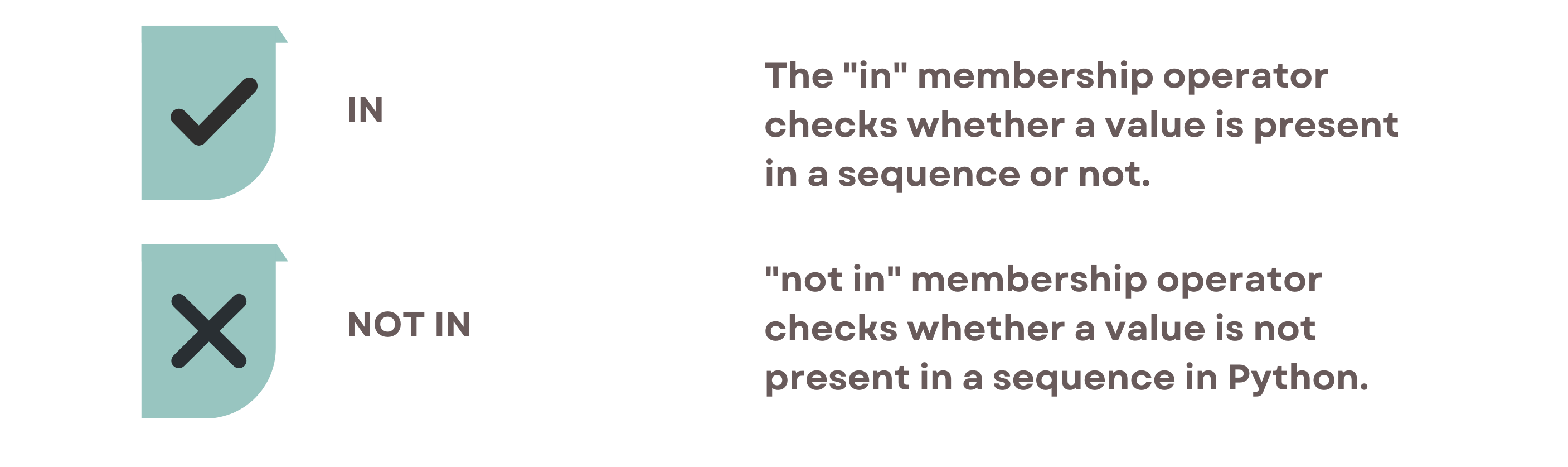
The in operator returns True if the specified value or object is found in the sequence and False otherwise. For example, in the code x = 3 in [1, 2, 3, 4], the value of x would be True because 3 is present in the list [1, 2, 3, 4].
The not in operator returns True if the specified value or object is not found in the sequence and False otherwise. For example, in the code y = 5 not in [1, 2, 3, 4], the value of y would be True because 5 is not present in the list [1, 2, 3, 4].
Now let’s see the code.
# in
x = 3 in [1, 2, 3, 4] # x is True
# not in
y = 5 not in [1, 2, 3, 4] # y is True
Python Assignment Operators
Variables were given values using assignment operators.
The equal sign, which assigns a value to a variable, is the most fundamental assignment operator.
Aside from the fundamental assignment operator, there currently are many compound assignment operators that execute an arithmetic operation and an assignment in a single step. These Python operators combine addition, subtraction, multiplication, division, and modulo with assignments to simplify coding.
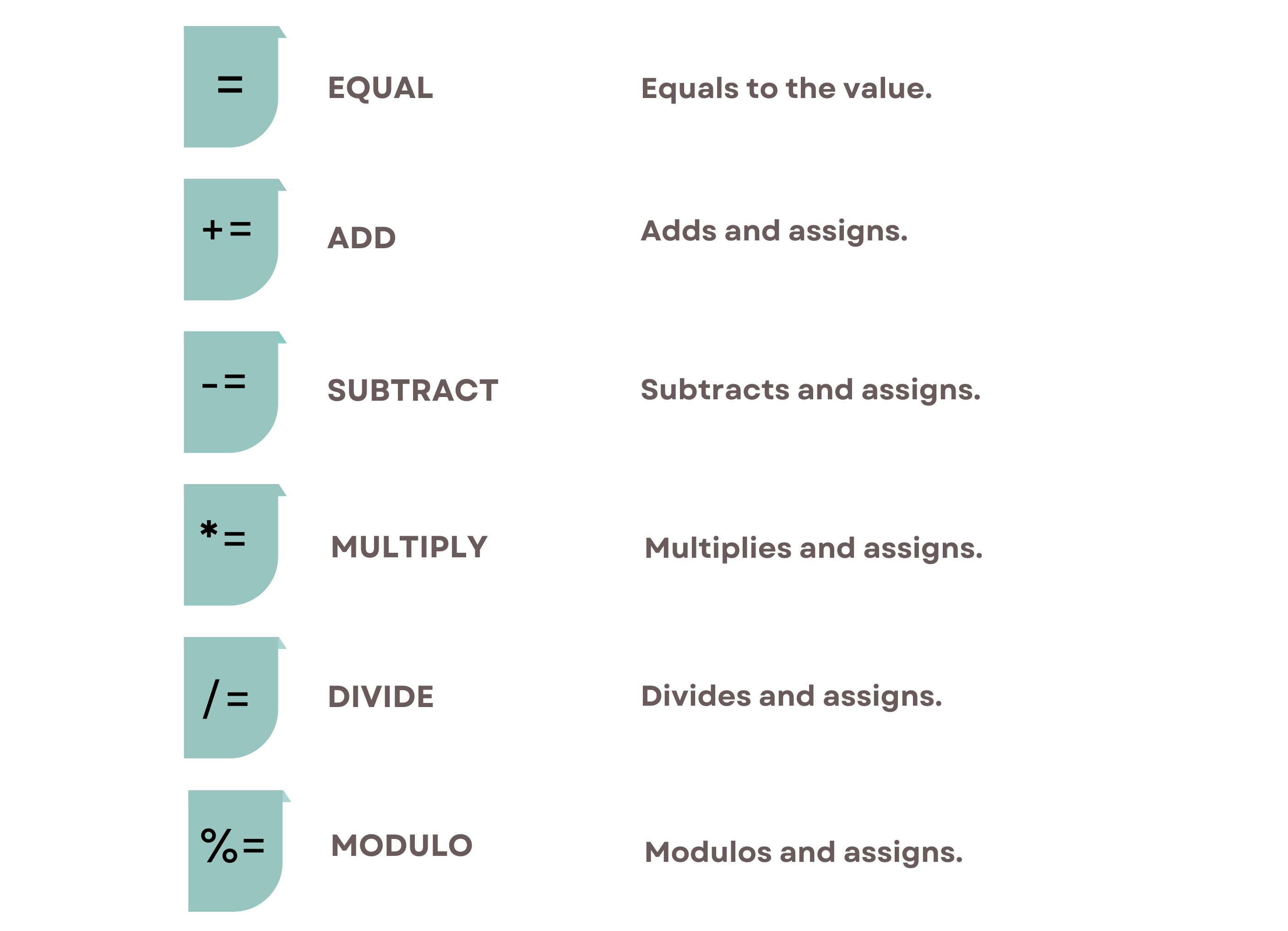
Compound assignment operators are a shorthand way to perform an operation and then assign the result back to the same variable.
For example, the "+=" operator adds the value on the right side to the variable on the left side and then assigns the result back to the same variable.
The "-=", "*=", "/=" and "%=" operators work in a similar way, but perform subtraction, multiplication, division, and modulo operations respectively.
For example,
- "a = 5" assigns the value 5 to the variable "a".
- "a += 5" adds 5 to the current value of "a" and assigns the result back to "a". So now "a" is equal to 10.
- "a -= 5" subtracts 5 from the current value of "a" and assigns the result back to "a". So now "a" is equal to 5.
- "a *= 5" multiplies the current value of "a" by 5 and assigns the result back to "a". So now "a" is equal to 25.
- "a /= 5" divides the current value of "a" by 5 and assigns the result back to "a". So now "a" is equal to 5.
- "a %= 5" calculates the modulo of the current value of "a" with 5 and assigns the result back to "a". So now "a" is equal to 0.
Now, let’s see the code.
# Assignment operator (=)
a = 5
# Compound assignment operator (+=)
a += 5 # a = a + 5 = 10
# Compound assignment operator (-=)
a -= 5 # a = a - 5 = 5
# Compound assignment operator (*=)
a *= 5 # a = a * 5 = 25
# Compound assignment operator (/=)
a /= 5 # a = a / 5 = 5
# Compound assignment operator (%=)
a %= 5 # a = a % 5 = 0
Python Bitwise Operators
Bitwise operators perform operations on the binary representations of integer values.
They are used for low-level manipulation of data.
AND, OR, XOR, and NOT are bitwise operators that carry out operations on individual bits in a number's binary representation.
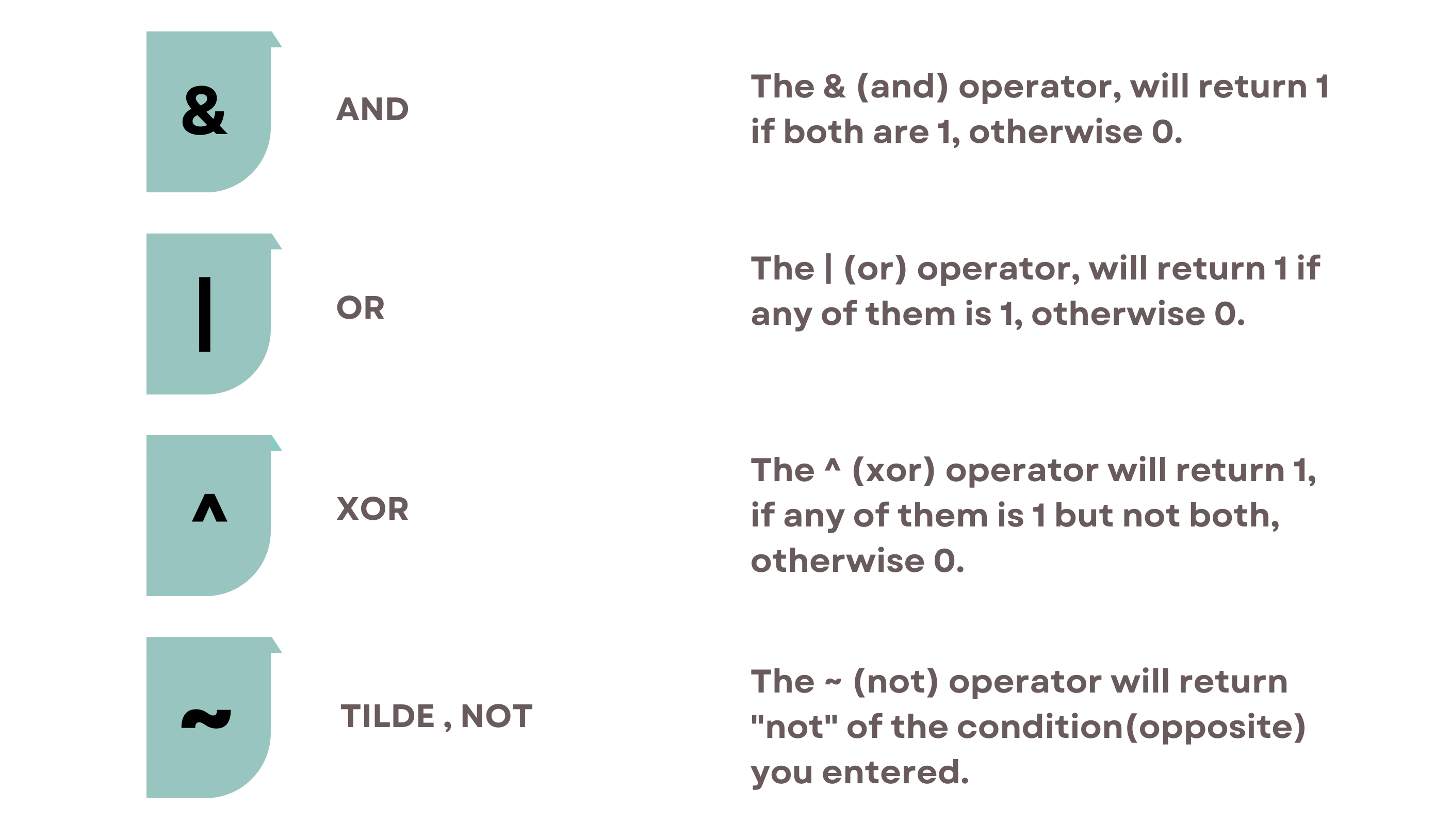
Now let’s examine bitwise operators by manipulating the built-in titanic data set. Here we will examine the bitwise operators used in Data Science and analytics.
Let’s load our Python libraries, and data frame and assign it as df.
import pandas as pd
import seaborn as sns
# Load the Titanic dataset
titanic_dataset = sns.load_dataset("titanic")
df = titanic_dataset
# Show the first 5 rows of the dataset
titanic_dataset.head()
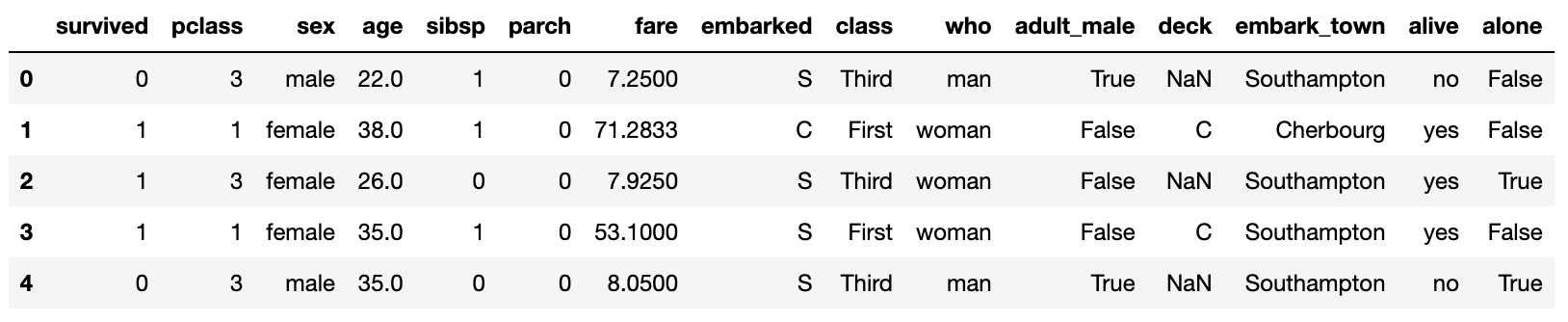
and
The "&" bitwise operator is used in the given code to perform a logical AND operation between two conditions.
The "&" operator compares the binary representation of each bit of the operands and returns 1 only if both bits are 1.
In the following code, the two conditions being compared are
"df["survived"] == 1" and "df["sex"] == "female".
The code is finding the number of rows in the "df" data frame where both of these conditions are true and dividing it by the number of rows where "df["survived"] == 1".
The result of this operation is the survival rate of women in the Titanic dataset.
The first part of the expression, "len(df[(df["survived"] == 1) & (df["sex"] == "female")])", returns the number of rows where both conditions are true, i.e. the number of women who survived.
The second part of the expression, "len(df[(df["survived"] == 1)])", returns the total number of people who survived.
The final result is the ratio of the number of women who survived to the total number of people who survived.
Now let’s see the code.
#Here, we use and operator to find the rate of survival rate of the woman in the Titanic dataset.
len(df[(df["survived"] == 1) & (df["sex"] == "female")]) / len(df[(df["survived"] == 1)])
Here is the output.
It looks like %68 of survived are women.

Great, now let’s try it by yourself.
The best way to learn is to practice.
Find all the songs that were top-ranked (at first position) at least once in the past 20 years
or
The "|" operator in Python is the bitwise "OR" operator. In the following code, it is used in combination with the comparison operator "==" to select rows from a pandas data frame "df" where the value in the "class" column is either "First" or "Third".
The expression df["class"] == "First" returns a Boolean array where each element is True if the corresponding row in the "class" column is equal to "First", and False otherwise.
The expression df["class"] == "Third" returns a similar Boolean array.
When the "|" operator is applied between these two arrays, it performs an element-wise logical OR operation, returning a Boolean array where each element is True if either of the two input arrays is True.
Finally, this Boolean array is passed as an index to the data frame, df[(df["class"] == "First") | (df["class"] == "Third")], to select only the rows where the value in the "class" column is either "First" or "Third".
Now let’s see the code.
df[(df["class"] == "First") | (df["class"] == "Third")]
Here is the output.
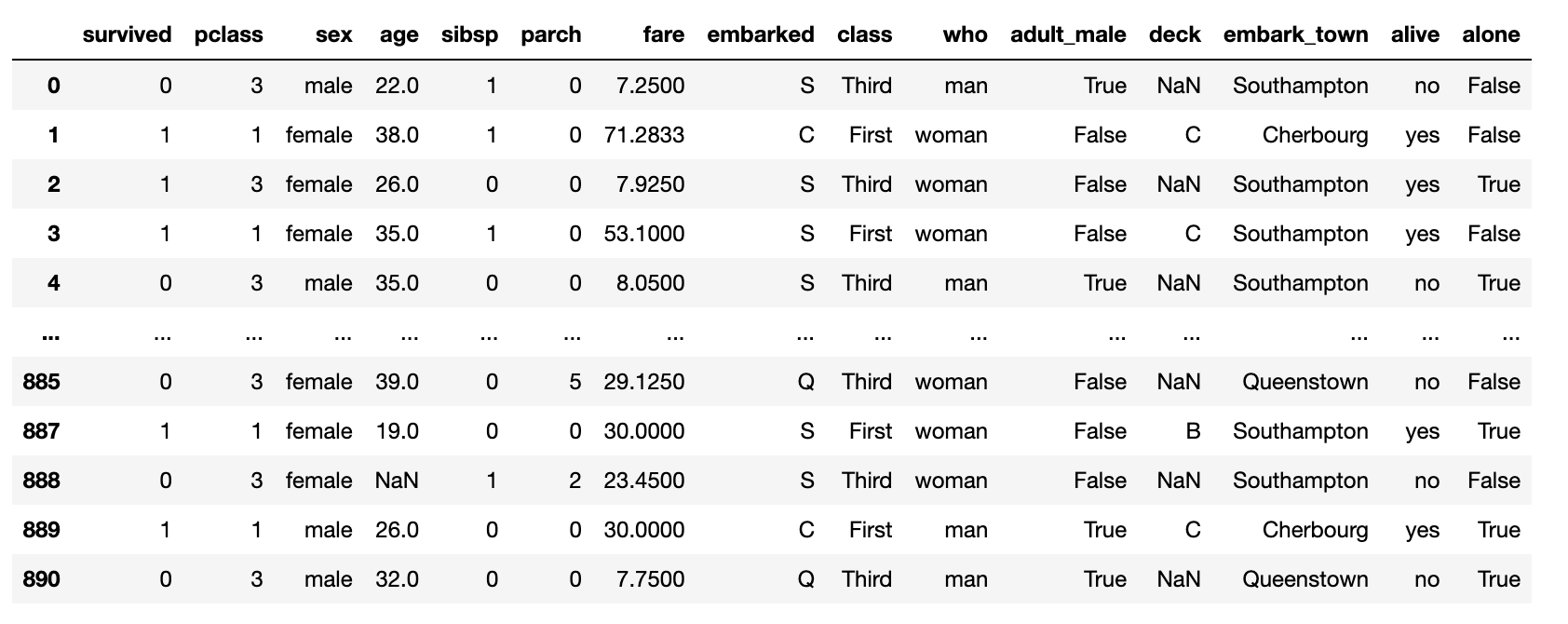
Now, let’s try it by yourself.
Here is the question from our platform.
You have been asked to find the employees with the highest and lowest salary across whole dataset.
Your output should include the employee's ID, salary, and employee's department, as well as a column salary_type
that categorizes the output by:
- 'Highest Salary' represents the highest salary
- 'Lowest Salary' represents the lowest salary
xor
The XOR (Exclusive OR) operator is a binary operator in programming that compares two values and returns True if exactly one of the values is True and False otherwise.
The code returns the rows of the data frame df, where the values in the "class" column are "First" or the values in the "alone" column are "False", but not both.
The "^" operator in this code is the XOR (Exclusive OR) operator.
The XOR operator returns True if exactly one of the operands is True, and False otherwise.
In this case, the XOR operator is used to combine the conditions in parentheses.
If the value in the "class" column is "First" and the value in the "alone" column is "False", both conditions would be True, and the XOR operator would return False.
But if either the "class" column is "First" or the "alone" column is "False", then one of the conditions would be True and the other would be False, and the XOR operator would return True.
Therefore, the code returns the rows where either the "class" column is "First" or the "alone" column is "False", but not both.
Now, let’s see the code.
df[(df["class"] == "First") ^ (df["alone"] == "False")]
Here is the output.
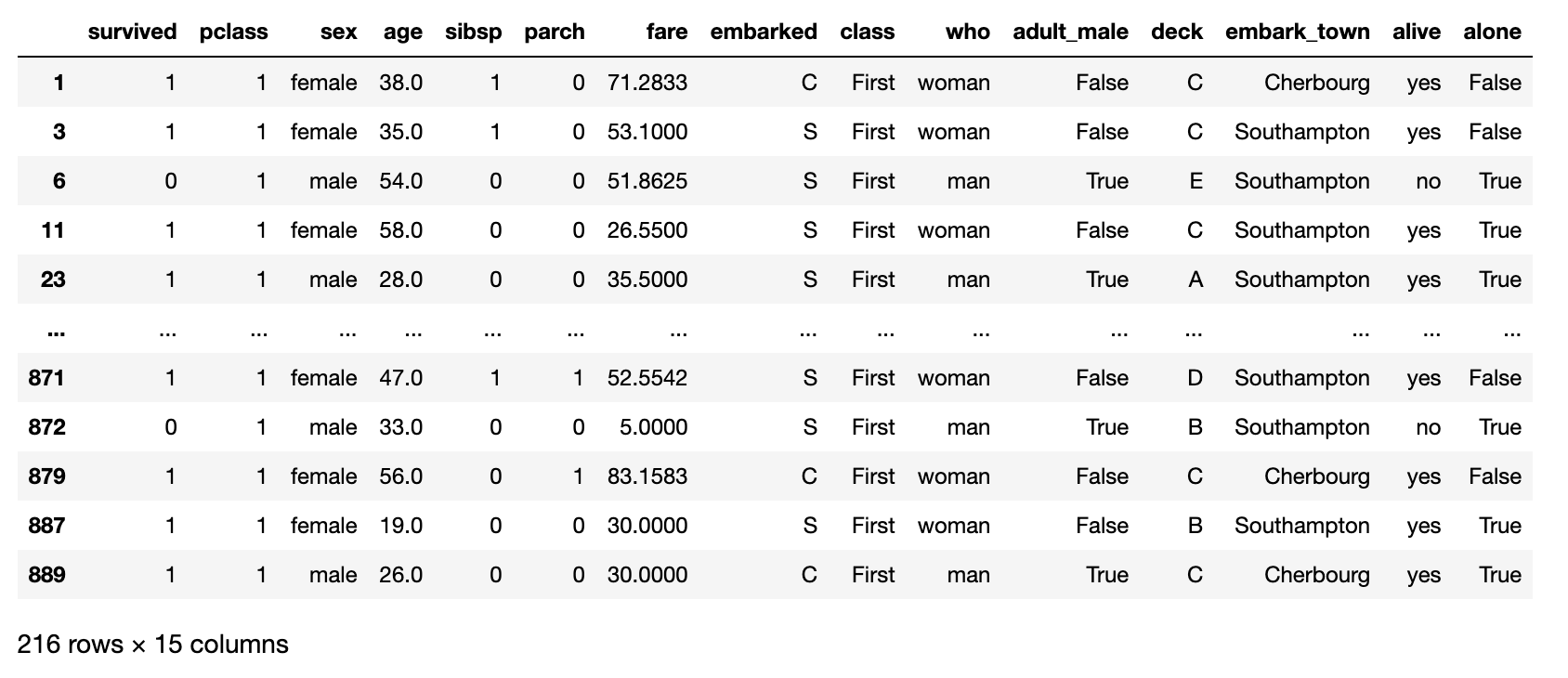
not
The tilde (~) operator is a bitwise NOT operator in Python. It inverts the bits of its operand, such that each 1 becomes 0 and each 0 becomes 1. In the context of a data frame, the ~ operator can be used to select all columns except a specific column.
In the following code, df.columns.isin(["survived"]) returns a Boolean mask indicating whether each column in df is equal to "survived".
The tilde operator inverts this mask, such that ~df.columns.isin(["survived"]) is True for all columns except "survived".
The .loc method is then used to select all rows of the data frame, but only the columns where the mask is True.
The result is assigned to a new data frame, df_without_survived.
Now let’s see the code.
# Select all columns except the "survived" column
df_without_survived = df.loc[:, ~df.columns.isin(["survived"])]
df_without_survived
Here is the output.
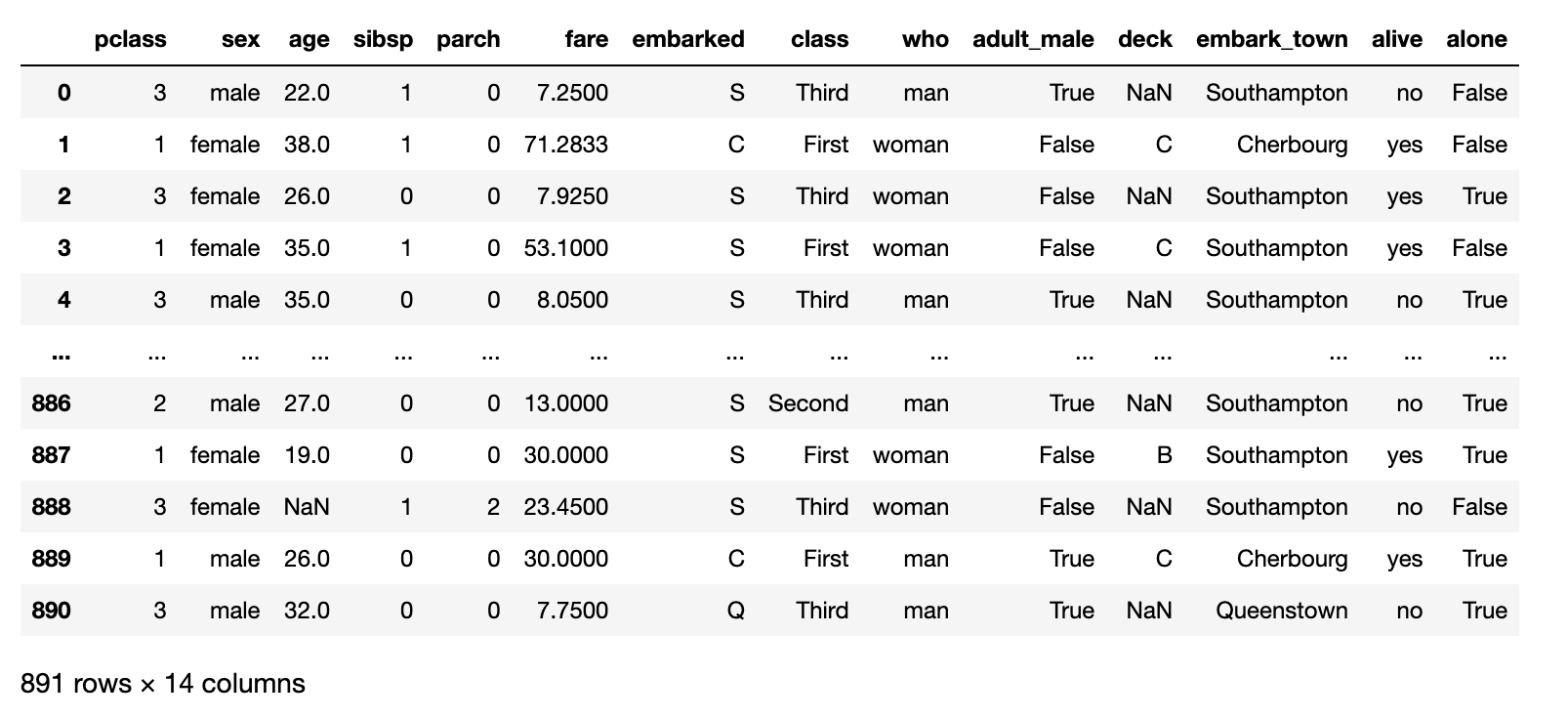
Great, here is another example from the platform. Let’s try solving it.
Last Updated: March 2020
Find the highest price in US country for each variety produced in English speaking regions, but not in Spanish speaking regions, with taking into consideration varieties that have earned a minimum of 90 points for every country they're produced in. Output both the variety and the corresponding highest price.
Let's assume the US is the only English speaking region in the dataset, and Spain, Argentina are the only Spanish speaking regions in the dataset. Let's also assume that the same variety might be listed under several countries so you'll need to remove varieties that show up in both the US and in Spanish speaking countries.
Python Identity Operators
Identity operators in Python are used to determine whether or not two objects have the same memory addresses. This differs from comparison operators, which compare object values rather than memory locations.
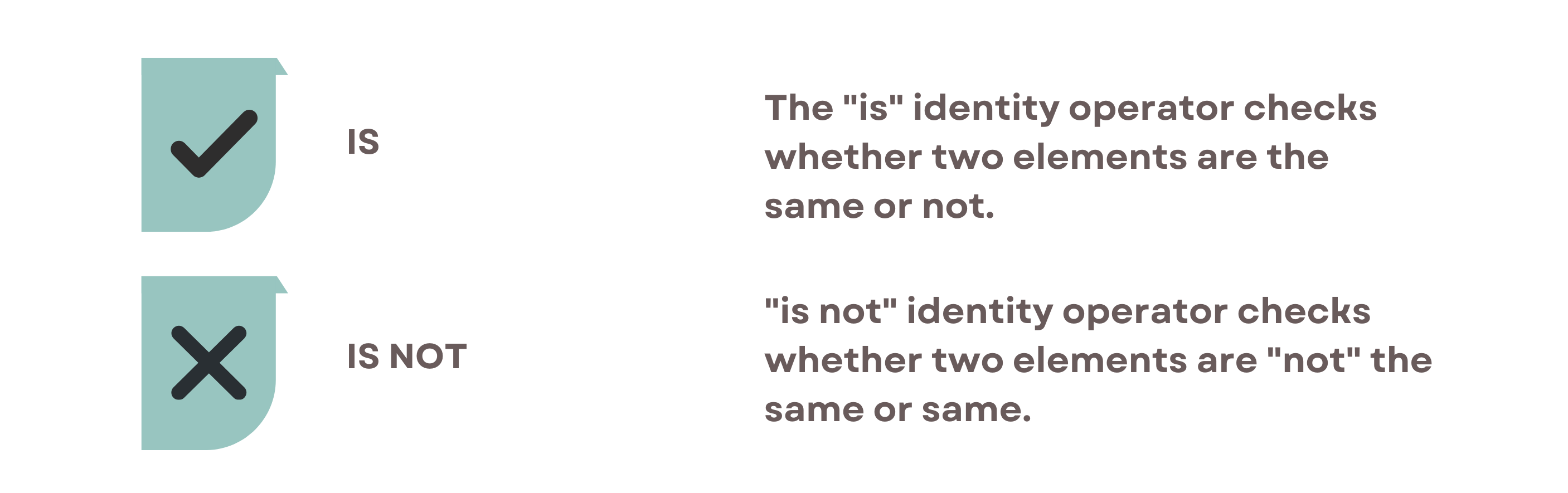
The 'is' operator outputs True when two variables relate to the same object in memory.
Yet, the 'is not' operator outputs True when the variables do not relate to the same object.
x = 5
y = 5
z = 10
print(x is y) # Output: True
print(x is z) # Output: False
print(x is not z) # Output: True
In this example, x and y have the same value and, due to Python's integer caching for small numbers, they also share the same memory address, so x is y returns True. The value of z is different from x, so x is z returns False.
Python Ternary Operators
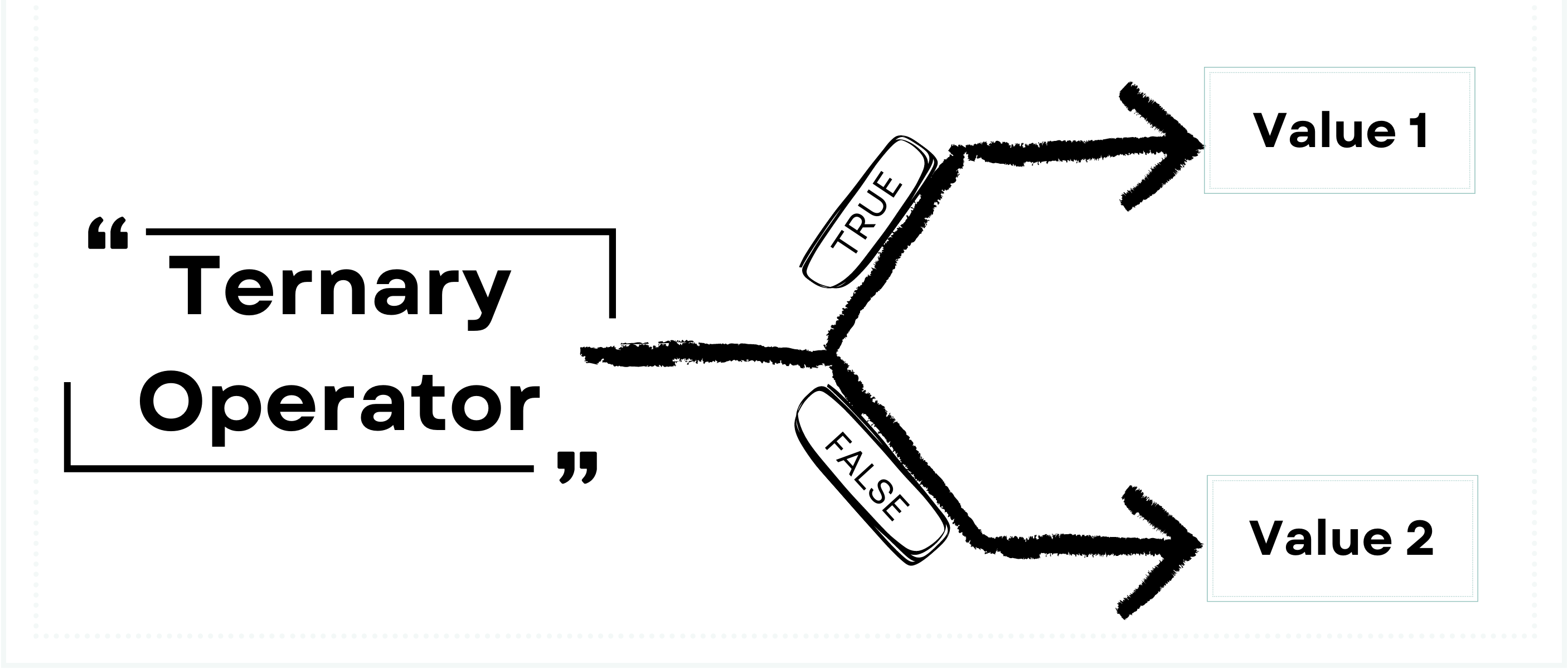
Ternary operators in Python provide a shorthand way of writing an if-else statement. The basic syntax of a ternary operator is as follows:
value_if_true if condition else value_if_false
In ternary operators syntax, first the condition is asses, and if it’s True then the first value is returned, if not the second is returned.
Here's an example of a custom function, which by using it, you can check whether the number is odd or even.
def odd_or_even(num):
result = "{} is odd number.".format(num) if num % 2 != 0 else "{} is an even number.".format(num)
print(result) # Output: odd
Now, let’s test our function.
odd_or_even(6)
Here is the output.

In the following example, the ternary operator is used to determine whether a passenger survived or not.
The function survived_or_not() takes an input of the passenger index and accesses the corresponding survived value in the data frame df.
The ternary operator then checks if the survived value is equal to 1, and if it is, returns the string "Passenger is survived.".
If the survived value is not equal to 1, the operator returns the string "Unfortunately, the passenger is not survived."
The result of the ternary operator is then printed.
Now, let’s see the code.
def survived_or_not():
passenger_number = int(input("Please give me the index of the passenger: "))
passenger = df.iloc[passenger_number]["survived"]
result = "Passenger has survived." if passenger == 1 else "Unfortunately, the passenger has not survived."
print(result)
Now, let’s test our code.
After running this code, it asks us to enter the number of passengers to run this code.
Here, I will enter 890 as an index. This is the last column of our dataset, let’s see.
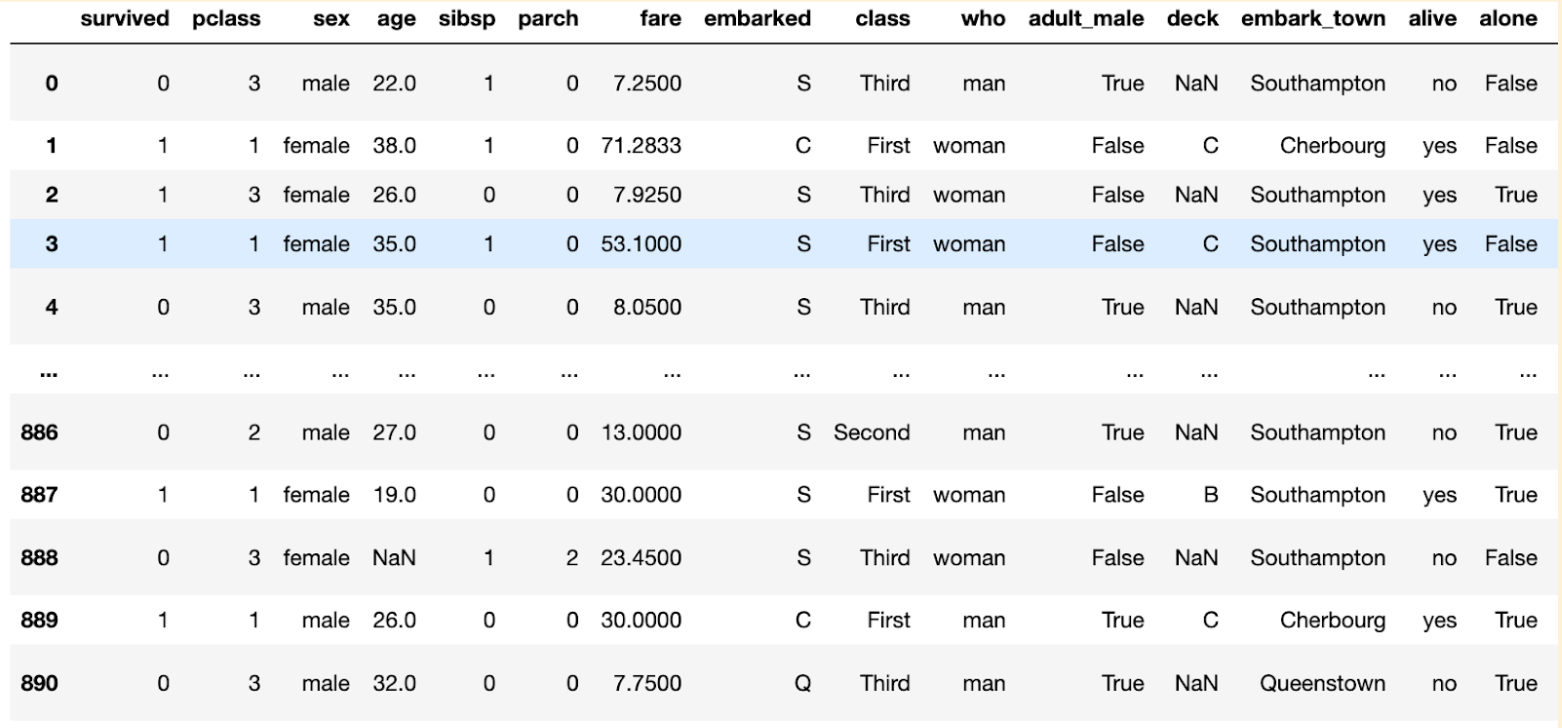
Here you can see, 890th passengers could not survive.
Here is the code.
survived_or_not()
Now let’s see the output of our custom function, which includes ternary operators.
Here is the output.

If you want to know more about Python, here is the Python Cheat Sheet.
Conclusion
In summary, Python operators are indispensable tools for developers working with the Python programming language.
By exploring the wide range of operators available in Python, including arithmetic, comparison, logical, membership, assignment, bitwise, identity, and ternary operators, programmers can tackle a variety of problems, Like writing effective, maintainable, and readable Python code.
They also enable concise and efficient manipulation of data and decision-making based on conditions.
As you continue to hone your Python programming skills, the mastery of these Python operators will serve as a strong foundation for solving complex challenges with confidence and ease.
If you want to further hone your Python skills and delve deeper into the world of Python programming and Data Science, don't hesitate to visit our platform.
For more, if you are preparing an interview, here are Python Interview Questions, you can study.
By expanding your knowledge and practicing your coding skills, you'll be better equipped to tackle any challenge that comes your way.
Share