Python Loops Explained: Here is How to Master Them
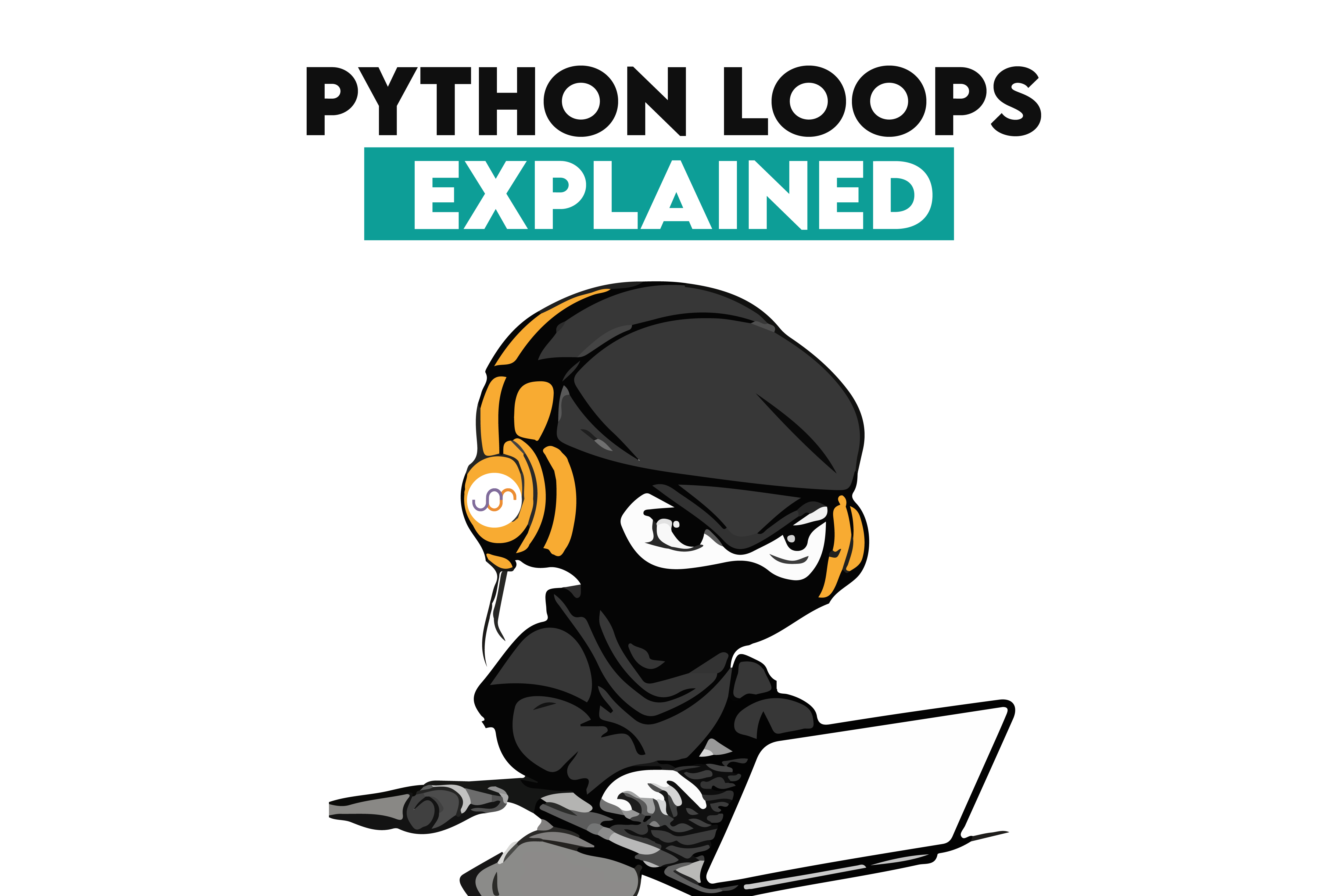
Categories
Discover the power and flexibility of Python loops to automate repetitive tasks and streamline your coding projects efficiently.
How can computers do the same things indefinitely, at precisely the same time, and with no mistakes?
Loops are the first element introduced when we start Python lessons. They are a simple concept but a potent tool for enabling computers to think and act independently.
In this article, I will demonstrate how to use for- and while-loops in Python and why you should use them to make your code robust.
What are Python Loops?
Loops in Python allow you to run a code block more than once. It is useful when you must do repetitive tasks and don’t want to write this code multiple times.
For example, Python loops can automate the process of analyzing large datasets.
Types of Python Loops
Python has two main loops: for and while. Their programming uses are unique from each other.
- For loop: It works best when looping types of sequences like lists, tuples, dictionaries, Python sets, and strings.
- While loop: It acts as long as the condition remains true.
Let’s see them in action.
for loop
The for loop in this example loops through the list of fruits, and the receiving value of the item assigned to the fruit variable is iterated in each bracket. Let’s see the code.
fruits = ["apple", "banana", "cherry"]
for fruit in fruits:
print(fruit)
Here is the output.

while loop
Here, the while loop continues to the extent that count < 5 exists. It then executes those parts of the code in the loop by printing the value of count, which is then incremented by one.
The execution of the code stops at the count of 5 once that condition becomes false. Here is the comment.
count = 0
while count < 5:
print(count)
count += 1
Here is the output.
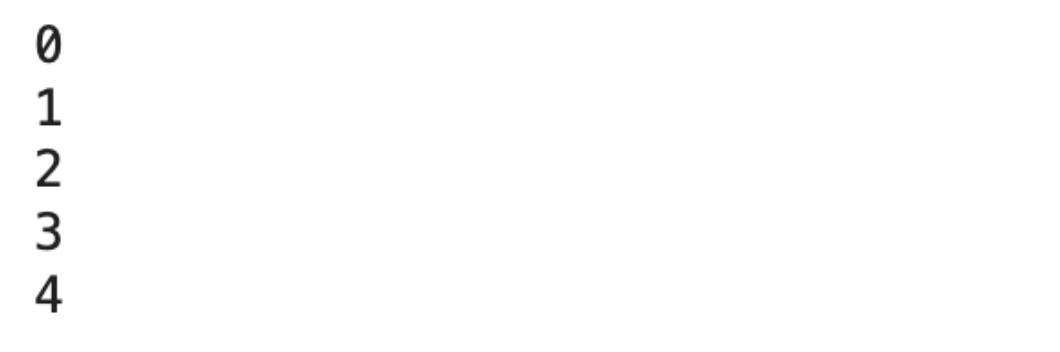
The for Loop
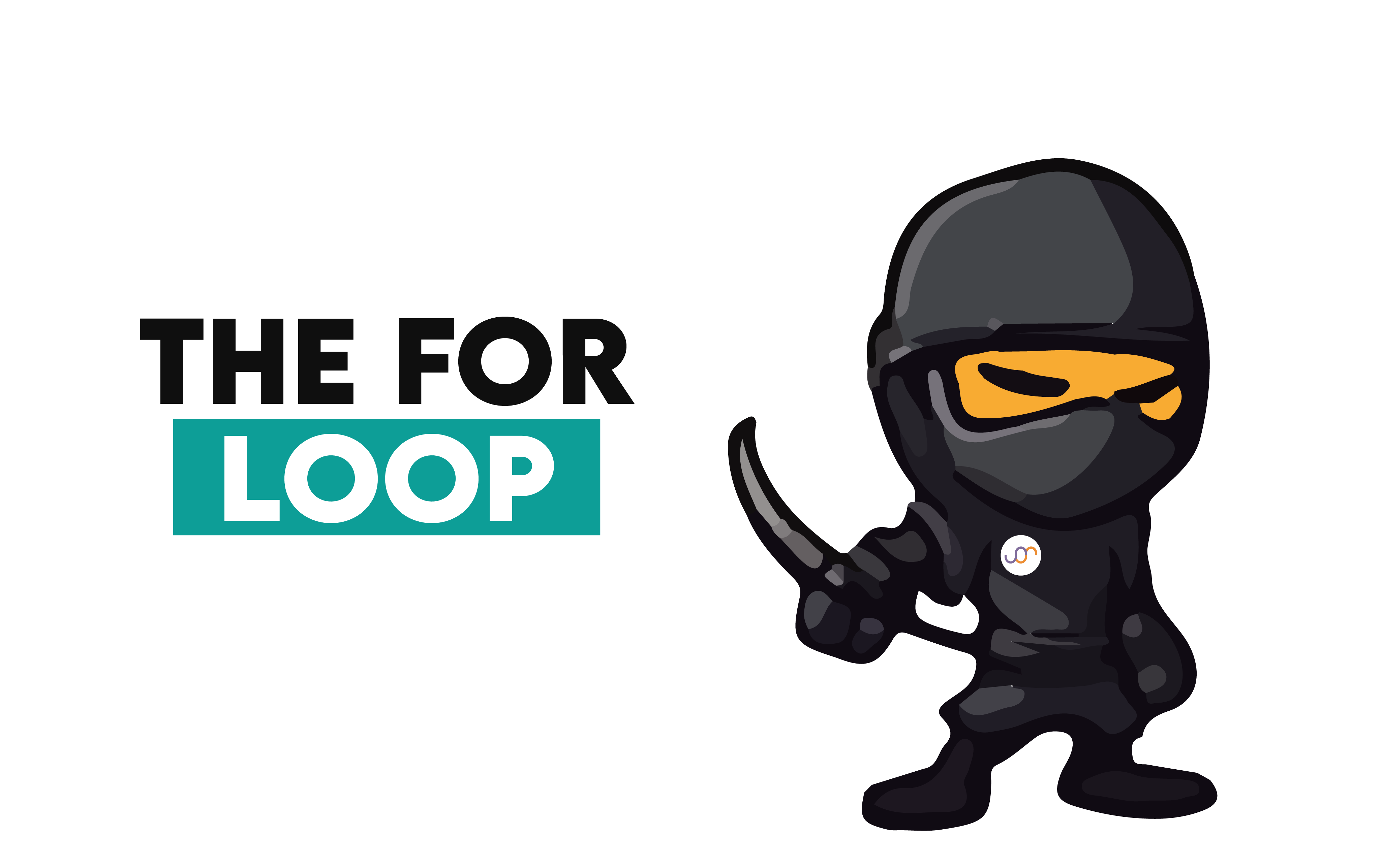
First, let’s see the syntax of it.
for loop syntax
Python for loop syntax is simple:
for variable in sequence:
# Perform actions using variable
How to iterate over sequences (lists, tuples, dictionaries, sets, strings)
One can iterate over any sequence type with a for loop in Python. For example, the most common sequence type in data science is a list of data points.
Example: imagine that we have a list of temperatures in Celsius and want to convert them into Fahrenheit. We can do that with the following code.
In the following code, we begin with the list of temperatures, loop through each temperature, transform it into Fahrenheit, and add it to the new list of temperatures_fahrenheit. Let’s see the code.
temperatures_celsius = [22, 25, 17, 15, 18]
temperatures_fahrenheit = []
for temp in temperatures_celsius:
temperatures_fahrenheit.append((temp * 9/5) + 32)
print(temperatures_fahrenheit)
Here is the output:

Use of the range() function
The range() function is helpful when producing a series of numbers.
Example: To illustrate, considering the data context, we may want numbers from 1 to 7 to prepare a list of them to represent days in a week for a statistical study.
Therefore, range(7) in the subsequent example yields numbers between 0 and 6, which aligns with the days of the week we studied.
for day in range(7): # Generates numbers 0 to 6
print(f"Study day: {day}")
Here is the output.
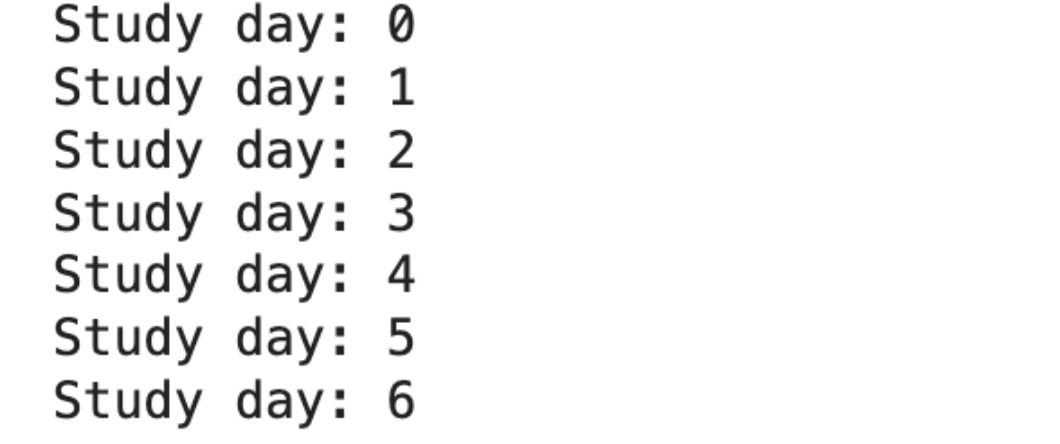
Nested for loops
A nested for loop is a loop in which the for loop can be employed to loop within a loop. This concept is generally used when dealing with multi-dimensional data.
Example: Let’s say you will analyze data by collecting data from multiple sensors across different days.
The following is some example code:
sensors = ["Temperature", "Humidity"]
days = ["Monday", "Tuesday", "Wednesday"]
for sensor in sensors:
for day in days:
print(f"Analyzing {sensor} data for {day}")
Here is the output.

Practical examples of Python for loop
We can take our example of temperature conversion and classify them as either “Cold,” “Warm,” or “Hot” according to their Fahrenheit value.
The code block below takes each temperature from the list in Fahrenheit, classifies it, and prints the appropriate message.
for temp in temperatures_fahrenheit:
if temp < 59:
print(f"{temp}F is Cold")
elif temp <= 77:
print(f"{temp}F is Warm")
else:
print(f"{temp}F is Hot")
Here is the output.
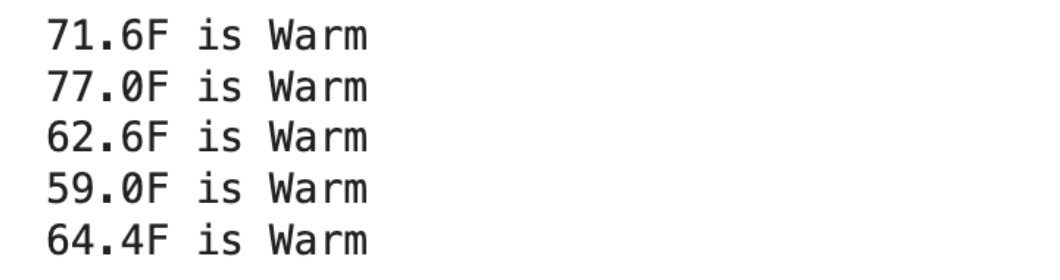
The while Loop
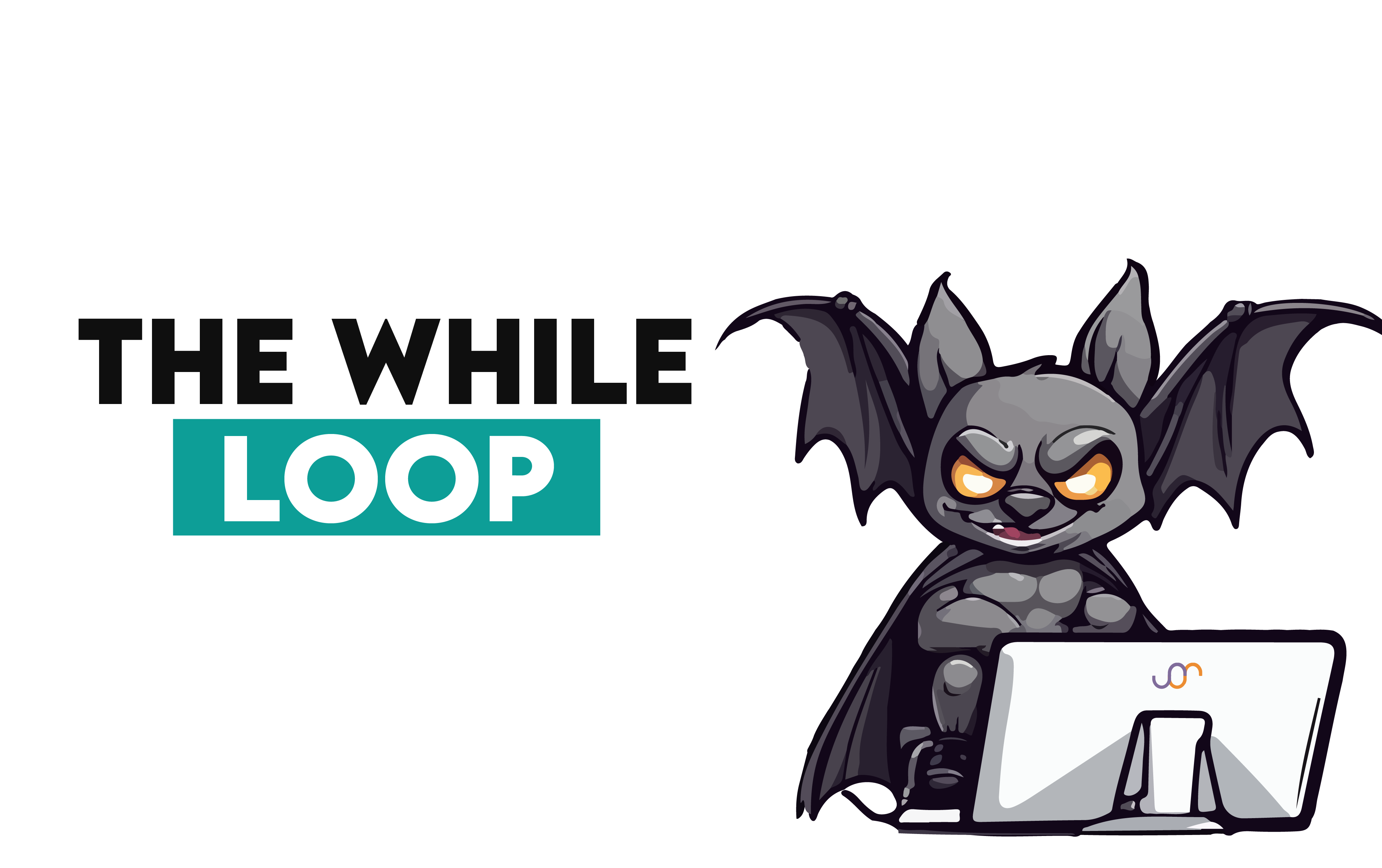
The while loop repeats as long as a condition is True.
while loop syntax
Here is the syntax;
while condition:
# code to execute
Condition-based nature of while loops
When unsure how often a block of code should execute, consider using a while loop.
In some cases in data science, a while loop may need to run until you reach a threshold, such as the accuracy of a model.
Creating infinite loops with a while
An infinite loop runs forever unless explicitly broken. It is usually not recommended but valuable when one wants to keep a server running to handle requests or other specific reasons.
Example: Considering our previous example of temperatures, let’s imagine a data stream of temperatures where we continuously keep reading temperatures until a command ‘Stop’ is encountered. Let’s see the code.
temperature_stream = iter(["22", "25", "Stop", "17"]) # Simulating a stream
temperature = next(temperature_stream)
while temperature != "Stop":
print(f"Received temperature: {temperature}")
temperature = next(temperature_stream)
Here is the output.

Nested while loops
You can use a nested while loop if you have more than one condition inside each other. It might look complicated. However, the application of it is straightforward, which you can understand from the following example.
Let’s see the code.
data_stream = iter(["Batch1-Data1", "Batch1-Data2", "End", "Batch2-Data1", "Stop"])
try:
batch = next(data_stream)
while batch != "Stop":
print(f"Processing {batch}")
data_point = next(data_stream)
while data_point != "End":
print(f" Data point: {data_point}")
data_point = next(data_stream)
batch = next(data_stream)
except StopIteration:
print("Finished processing all batches.")
Here is the output.
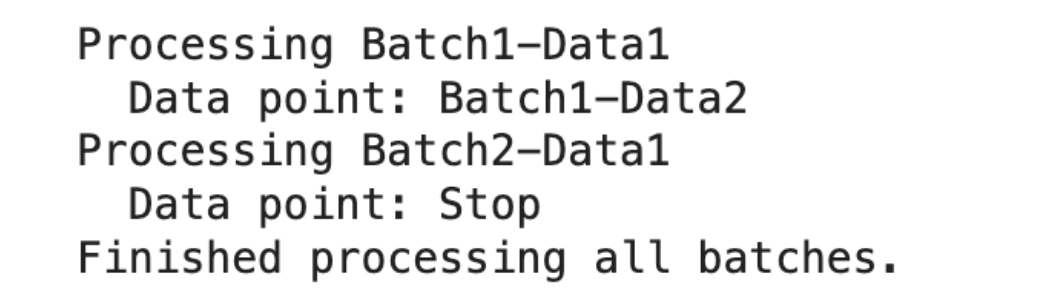
This approach emulates data processing in batches, each having multiple data points, terminating upon the arrival of the “Stop” batch. Here, the “End” signified the conduction of each such batch.
Practical examples of Python while loop
Having illustrated how temperature processing was done before, we can now introduce a condition to stop the loop if the temperature is above some threshold, which means a sensor error. Below is our code:
# Simulating a stream with a high temperature
temperature_stream = iter(["22", "25", "33", "Stop", "17"])
temperature = next(temperature_stream)
while temperature != "Stop":
if temperature == "33": # Assuming 33 indicates a sensor error
print("Sensor error detected, stopping.")
break
print(f"Received temperature: {temperature}")
temperature = next(temperature_stream)
Here is the output.

Loop Control Statements
Loop control statements change the execution of a loop from its typical sequence. They are crucial for managing the flow of loops efficiently, especially in complex logic scenarios.
break, continue, and pass
- break: Stop the loop without iterating.
- continue: Continue with the next iteration after skipping the current loop code.
- pass: Do nothing; it's a placeholder for future code.
Examples of these statements
Now we learn “break,” “continue” and “pass” statements. But, to solidify our understanding, let’s use them in one example to see how they can handle different situations.
In this example, we will see a simulated data stream where we will ignore temperature below 20, and if there is a sensor error, we’ll break the loop with an error. Otherwise, we’ll continue. Let’s see the code.
# Simulated data stream
temperature_stream = iter(["19", "22", "25", "Sensor Error", "17"])
for temperature in temperature_stream:
if temperature == "Sensor Error":
print("Sensor error detected, stopping.")
break # Exiting the loop on error
elif int(temperature) < 20:
continue # Skipping this iteration
# Placeholder for future logic
print(f"Logged temperature: {temperature}")
Here is the output.

Advanced Topics of Python Loops
There are many advanced things you can learn about loops in Python. These can help you handle challenges better. In the following sections, generators and list comprehensions are discussed. Let’s start with Generators.
Generators
Generators and iterators are two ways to improve loops. One way is to make things one at a time as they go instead of saving the whole chain, which could be very long in memory.
People who work in data science often have to deal with huge files or streams of data. In the following example, we’ll continue using temperature data; by this time, we will add a generator. Let’s see the code.
def temperature_stream():
for temperature in ["22", "29", "Sensor Error", "17"]:
yield temperature
for temperature in temperature_stream():
if temperature == "Sensor Error":
print("Sensor error detected, stopping.")
break
print(f"Logged temperature: {temperature}")
List Comprehensions with Conditional Logic
List comprehension is the easiest and the most efficient way to create lists, especially when it will be used for loops. You can also add conditions after the for loop.
In the following example, we can turn our list of Celcius temperatures into Fahrenheit.
temperatures_celsius = [22, 25, 17, 15, 18]
temperatures_fahrenheit = [(temp * 9/5) + 32 for temp in temperatures_celsius if temp >= 20]
print(temperatures_fahrenheit)
Here is the output

Best Practices and Common Mistakes
Before finishing, let’s look at the best practices and common mistakes.
Best Practices
Let’s look at best practices while writing Python loops.
- Use meaningful loop variable names: Don't use random letters like “i” or “x” as loop variable names; use the names that will make sense for your project.
- Leverage list comprehensions: List comprehensions are the classy way of creating variables into a list, and not only is it elegant, it is a neater way to make lists, too.
- Use range() wisely: When working with data, range () is handy because it allows you to examine the elements of a list or collection individually.
- Be cautious with nested loops: Nested loops can slow down the system, so don’t use them if you have to.
Common Mistakes
Yes, you need to follow best practices. But do you know what mistakes to avoid? Let’s discover them too.
- Creating Infinite Loops: It's common to make them accidentally, especially with while loops.
- Forgetting to update the condition variable: The variable that sets the condition for the while loop must be changed during the loop. Otherwise, it will lead to an endless loop.
- Misusing break and continue: They are best applied when neither the loop nor the store word makes the most sense in a specific situation.
- Ignoring enumerate(): You should use enumerate if you need both the thing and its index.
If you want to learn Python by solving Interview questions, read these Python interview questions.
Conclusion
In this article, we've examined the basics of Python loops for both while and for. We learned their importance in data science through real-world examples and tips, such as automating tasks and making programming processes run more smoothly.
To continue enhancing your Python skills by solving interview questions and doing data projects, visit our StrataScratch platform.
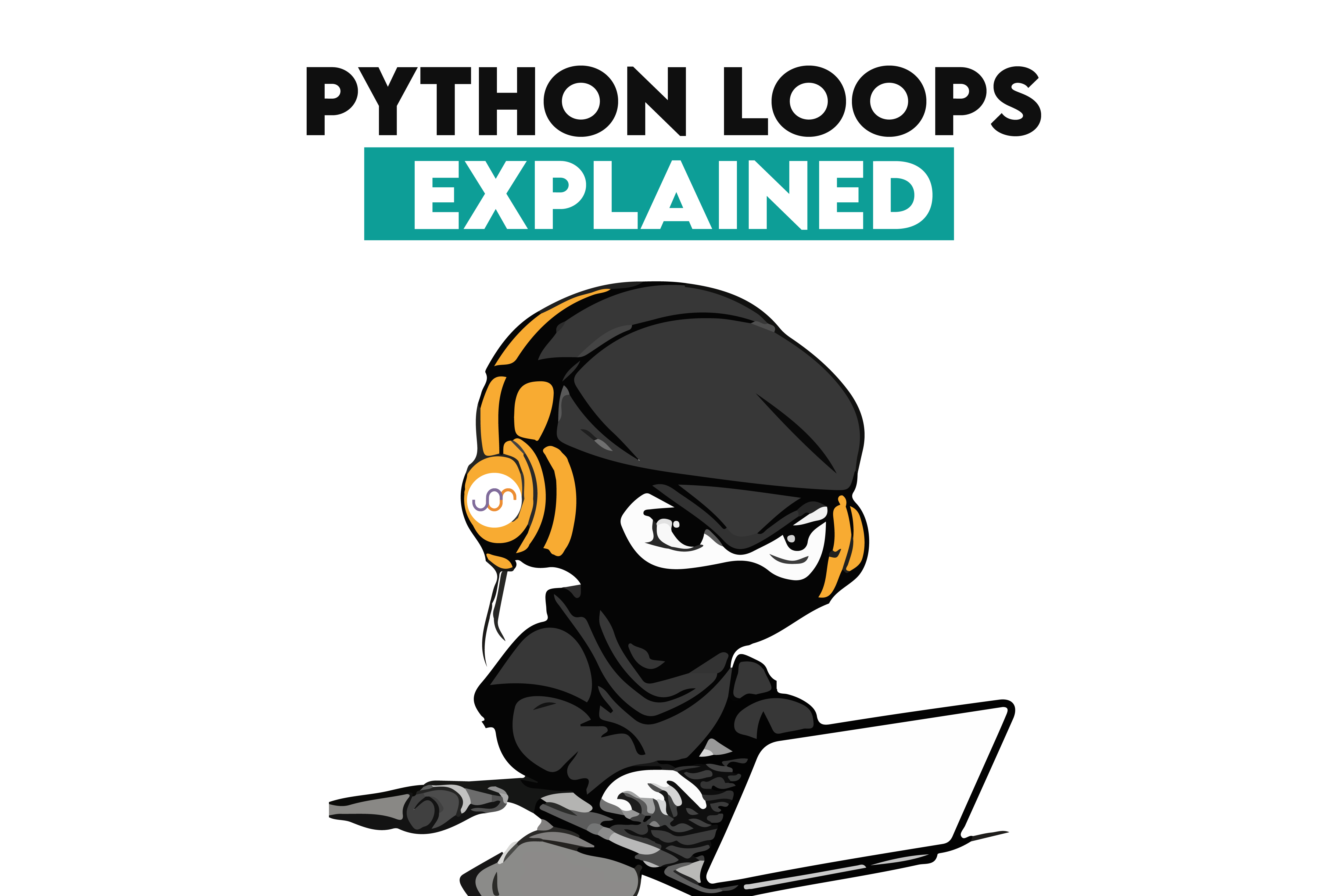