A Comprehensive Guide to Advanced Python Concepts

- Written by:
Nathan Rosidi
Advanced Python explained in a way that even beginners can get it. We’ll give you an overview of the concepts and then dedicate a separate article to each.
Thanks to its simplicity and versatility, Python is a popular programming language.
As you progress in your Python journey and data science career, you might encounter advanced Python concepts that may seem intimidating at first. Don't worry!
In this article, we will explore some of the essential advanced python concepts and provide easy-to-follow examples to help you understand them.
Whether you are preparing for a data science project, an interview, or expanding your knowledge, this article will help you to get up to speed on the advanced topics in Python.
We will explore topics in Python ranging from the basics, such as strings and numbers, to more advanced concepts like operators, Booleans, lists, dictionaries, deep and shallow copying of data frames, regular expressions, and decorators.
We’ll start with the more straightforward topics and move towards more complex ones.
Get ready to take your Python skills to the next level with the help of this comprehensive guide on advanced Python concepts.
Advanced Python Concepts

Data Types
Python provides a range of built-in data types, each designed for a specific purpose. In this section, we will give a brief introduction to some of these data types, which are string, numeric, sequence, set, Boolean, and binary data types.
Note: We didn’t mention dictionaries which are a mapping data type. Due to their importance and extensiveness, we’ll talk about dictionaries in a separate section and dedicate a whole article to this topic.
Now let’s get started with strings.
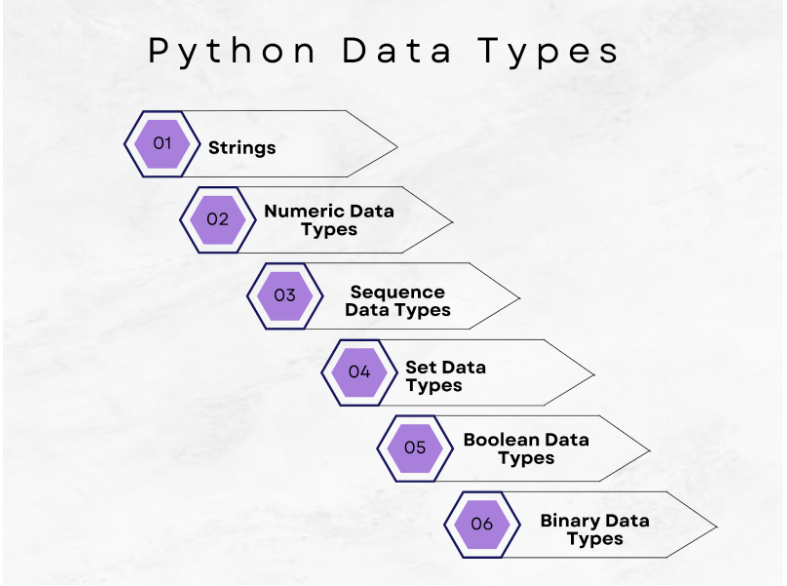
Strings
In Python, strings are a data type used to represent text. They can be created using single or double quotes and can contain a range of characters, including letters, numbers, symbols, and spaces. Strings can be manipulated in various ways, including concatenation, slicing, and formatting.
Numeric Data Types
Numeric data types in Python refer to a set of data types that are used to represent numerical values. The most common are integers, floating-point numbers, and complex numbers.
These data types are utilized to represent mathematical values, such as calculations and numbers.
Integers
Integers, which are whole numbers without decimal places, can have positive, negative, or zero values. They are primarily used to represent counting numbers, scores, or ratings.
For example, 5, -3, and 0 are all integers.
Float
Floating-point numbers, or floats, are decimal numbers that can also possess positive, negative, or zero values. These types of numbers are used to represent values that have decimal places, such as weight, height, or temperature.
For example, 3.14, -2.5, and 0.0 are all floats.
Complex Data Type
The complex data type consists of numbers that have both real and imaginary parts. The "j" operator is used to denote the imaginary component. They are commonly used in fields such as engineering and physics.
For example, 2 + 3j, -1j, and 4 - 2.5j are all complex numbers.
Sequence Data Types
Sequence types in Python are data types that represent sequences of values. The two common sequence types we’ll talk about are tuples and ranges.
Note: List is also a sequence data type. Due to its importance and extensiveness, we’ll talk about it in a separate section and dedicate a whole article to this topic.
Tuple
Tuples are collections of immutable ordered elements, meaning their values cannot be changed once created. Tuples can hold elements of different data types and are frequently utilized to group related data.
Range
In Python, the built-in function range() creates a sequence of integers. It can take up to three arguments: the starting number, the ending number (not included in the sequence), and the step size. range() objects are commonly employed in loops to perform a specific number of iterations.
Set Data Types
The set data types in Python are used to store a collection of unique elements. There are two main types of set data types in Python: set and frozenset.
Set
Sets are collections of elements that are unordered and contain no duplicates. They can be created using curly braces or the built-in set() function. Sets are commonly used to perform set operations like union, intersection, and difference.
Frozenset
Frozensets are sets that are immutable, meaning their elements cannot be modified after creation. They are commonly used as keys in dictionaries or as elements in other sets. Once created, their values remain constant.
Boolean Data Type
The Boolean data are a type of data in Python that can represent binary values, which are either True or False. These values are used in programming to make logical decisions and control program flow.
Python uses the keywords "True" and "False" to represent Boolean values. The Boolean data type is crucial in programming and extensively used in conditional statements, loops, and logical operations.
In summary, Booleans are a fundamental data type in Python programming, and their ability to represent binary values is essential to many programming concepts and operations.
Binary Data Types
Binary Types are essential to handling and manipulating binary data in Python. Python's three most important binary types are bytes, bytearray, and memoryview.
Bytes
Bytes represent immutable sequences of bytes that represent binary data, such as images, audio, or video files. You can create bytes using the b prefix or the bytes() constructor.
Bytearray
Bytearrays are similar to bytes but are mutable, meaning that their values can be modified after creation.
They can be created using the bytearray() constructor.
Memoryview
Memoryview is a memory-efficient way to access the data in a bytes object without copying it to a new object.
You can view the data as a sequence of bytes or other data types using memoryview, which is particularly useful when working with large amounts of binary data.
To learn more about Python, check out this Python Cheat Sheet for a quick reference. You can use it for common tasks related to data structures, functions, and data wrangling, which makes it a valuable resource for learning and mastering these python concepts.
Operators
In Python, different types of operators are used to manipulate data and perform various operations. These operators are arithmetic, comparison, logical, membership, assignment, bitwise, identity, and ternary operators.

Arithmetic operators are used to perform basic mathematical operations such as addition, subtraction, multiplication, and division on numerical data types like integers, floats, and complex numbers.
Comparison operators compare two values and return a Boolean value of either True or False.
Logical operators allow combining multiple conditions into a single condition using keywords such as "and," "or," and "not." Logical operations return a Boolean value.
Membership operators check whether an object is a member of a sequence like a list or string.
Assignment operators are used to assign a value to a variable.
Bitwise operators perform bit-level operations on integers, including binary numbers.
Identity operators compare the identity of two objects to check if they refer to the same memory location.
Ternary operators allow writing if-else statements concisely in a single line of code.
Arithmetic Operators
Arithmetic operators in Python are used to perform mathematical operations on variables, numbers, or expressions.
There are several arithmetic operators in Python.
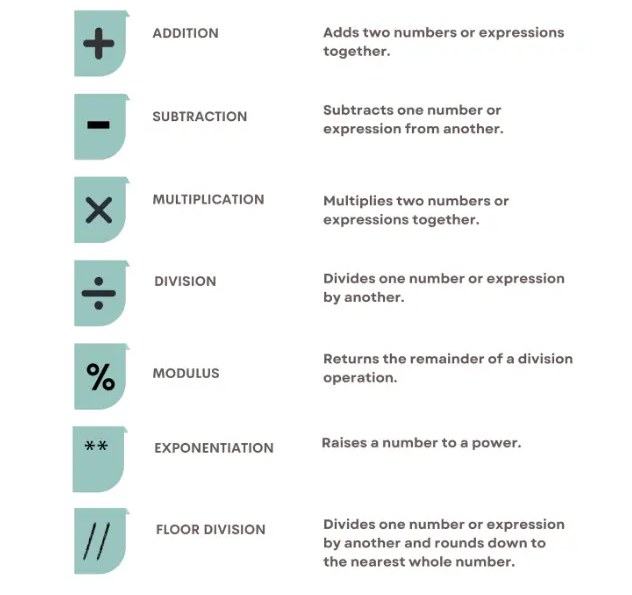
Comparison Operators
Comparison operators are used in Python to compare two values and return a Boolean value indicating whether the comparison is True or False.
There are several comparison operators available in Python.

Logical Operators
Logical operators are used in conditional statements to combine multiple conditions and make decisions based on the truth value of the conditions.
The three logical operators in Python are "and", "or", and "not".
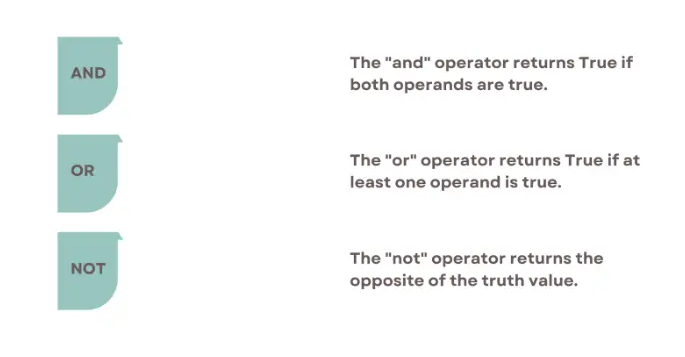
Membership Operators
The membership operators in Python allow you to check whether a value or an object is present in a sequence.
Python has two membership operators: "in" and "not in".
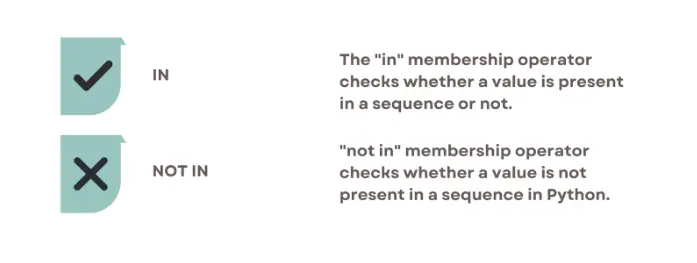
Assignment Operators
Assignment operators are used to assign a value to a variable in Python. The most basic assignment operator is the single equal sign "=", which assigns the value on the right side to the variable on the left side.

Bitwise Operators
Bitwise operators are operators in programming that perform operations on binary representations of numbers.
They work on the individual bits of the numbers rather than on the numbers as a whole. In Python, the bitwise operators are "&" (and), "|" (or), "^" (xor), and "~" (tilde or not).
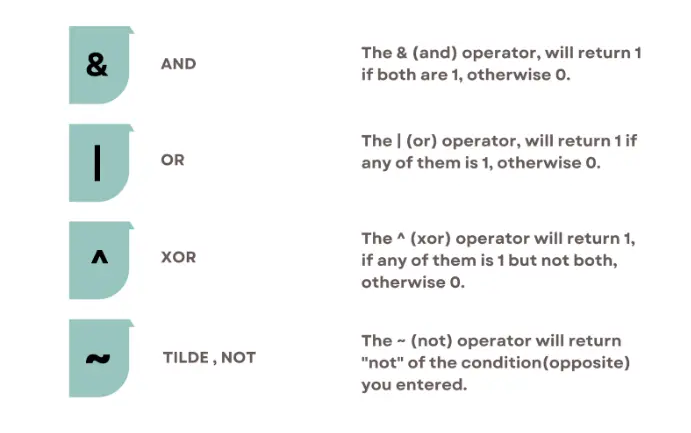
Identity Operators
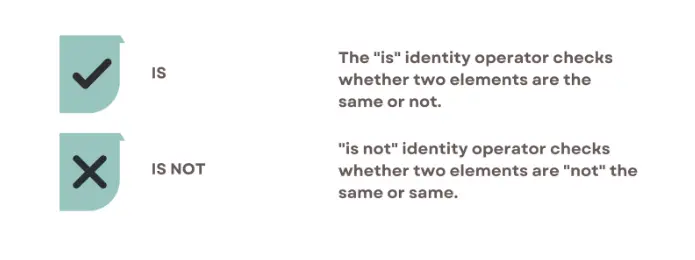
In Python, the identity operators compare the memory locations of two objects instead of their values. "is" and "is not" are the two identity operators available. "is" operator returns True if two variables point to the same object in memory, and False if they do not.
On the other hand, the "is not" operator returns True if two variables point to different objects in memory and False if they point to the same object.
Ternary Operators
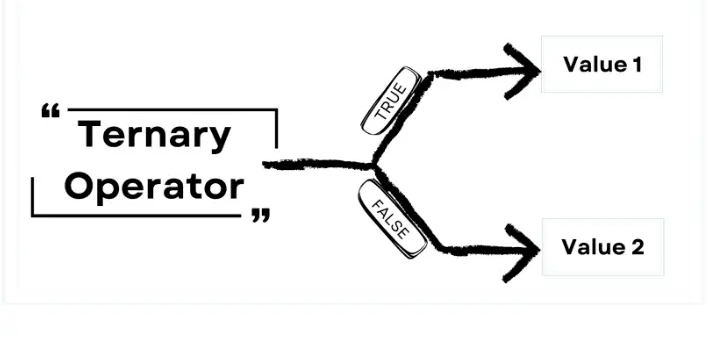
Ternary operators offer a shorter way of writing if-else statements and are also called conditional expressions.
The ternary operator consists of a condition, a value to return if the condition is True, and another value to return if the condition is False.
The condition is evaluated first, and if True, the first value is returned, and if False, the second value is returned.
Lists in Python
In Python, lists belong to the group of data types called sequence data types, which are utilized to represent ordered collections of values.
Python lists are ordered, mutable, and can hold any data type, including other lists.
Each element in the list is identified by an index, which is a non-negative integer representing its position in the list.
Creating a list is straightforward, and you can use square brackets "[item1, item2 ]" with items separated by commas or the list() constructor.
Python lists support a variety of operations, such as adding or removing items, slicing, concatenating with other lists, sorting, reversing, and iterating over items in the list using loops.
Since lists are mutable, you can modify their contents by adding, deleting, or updating items at specific positions.
Overall, Python lists are a flexible and essential data structure that can be utilized in various programs and applications.
Dictionaries
A dictionary in Python is a mapping data type, which is a collection of key-value pairs that are not ordered.
The keys in a dictionary are unique, and their corresponding values can be of any data type, including other dictionaries.
You can use integers, strings, or tuples as a key.
Python dictionaries can be created using curly braces, { ‘a’: ‘b’ }, with each key-value pair separated by a colon or by using the dict() constructor.
Dictionaries support a variety of operations, including adding, updating, deleting, or accessing key-value pairs.
You can also use loops to iterate over the keys or values in a dictionary.
Dictionaries are mutable, which means that you can modify their contents by adding, removing, or changing the value of a key, or by updating the entire dictionary.
Overall, dictionaries are a powerful Python data structure that enables efficient lookups and structured data storage.
Since dictionaries belong to the mapping data type, they are ideal for organizing and storing structured data, especially when you need to look up specific values quickly using unique keys.
Other Advanced Python Topics
In addition to the commonly discussed advanced Python topics, several other important features can enhance your data manipulation abilities. These advanced Python concepts may receive less coverage but are still highly relevant and can significantly benefit your Python programming skills.
One of these topics is shallow vs deep copying of Python DataFrames. When copying data in Python, you can choose to create a new object that either references the original data or contains new data.
Another important advanced Python concept is regular expressions. Regular expressions are a valuable and powerful feature in Python for searching and manipulating text data.
Finally, decorators are a key feature in Python that enable you to modify the behavior of a function or class without changing its source code.
Now let’s look at these advanced Python concepts by starting with shallow & deep copying.
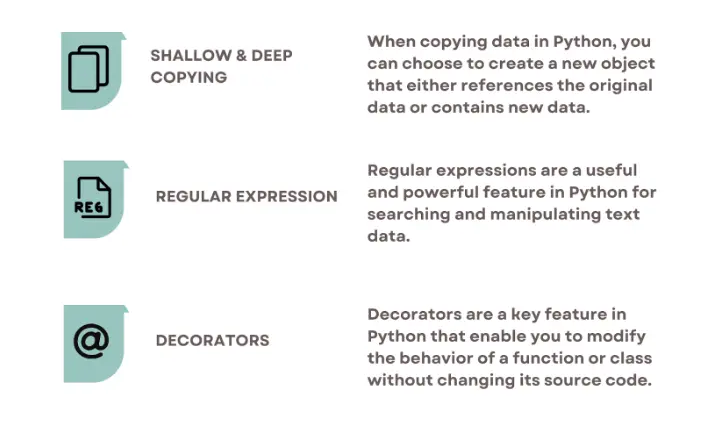
Shallow vs Deep Copying of Python DataFrames
Shallow vs deep copying of Python DataFrames refers to the two methods of creating a new DataFrame from an existing one.
In shallow copying, the new DataFrame shares the same memory location as the original DataFrame, so any changes made to the new DataFrame will affect the original DataFrame.
On the other hand, deep copying creates a new, independent copy of the original DataFrame, so any changes made to the new DataFrame will not affect the original DataFrame.
If you want to know more about common Python libraries, discover the top 18 Python libraries that can maximize your potential as a data scientist in 2023.
Regular Expressions
A search pattern can be defined in Python using a regular expression (regex or regexp), which consists of a sequence of characters.
Regular expressions are commonly used in programming languages like Python to search and manipulate text. A regular expression comprises a combination of special characters and text. These special characters have a special meaning and can be used to match specific patterns in the text.
For example, the "." character is used to match any single character, while the "*" character is used to match zero or more occurrences of the preceding character.
Suppose you have a large dataset of email addresses and want to extract the domain names from these email addresses. You could use regular expressions by searching for the pattern that matches the domain name.
Or regular expressions can be used to combine two data groups by specifying a pattern to match and merge the data that matches that pattern. For example, you can use regular expressions to extract and merge all the phone numbers in a list of customer data or to extract and merge all the email addresses in a text document.
Of course, there are other ways you can combine text or even the two different data groups by using pandas joins.
Here are the Types of Pandas Joins in Python.
Decorators
A decorator is a function that takes another function as input and returns another function as output.
The idea behind decorators is to modify the behavior of the original function without changing its code.
Mastering Python can significantly improve your chances of landing a job, particularly in data science. To prepare for interviews with top companies such as Amazon, Microsoft, and Google, you can practice solving Python Interview Questions commonly asked in data science interviews.
Conclusion
Advanced Python concepts are a must-know for every data scientist who wants to take their skills to the next level. This article explored data types, operators, lists, dictionaries, and other advanced Python concepts.
In the separate blog article, we will explain each of these concepts even in more detail (and with code!).
Understanding these concepts will take your Python skills to the next level and help you tackle complex projects with ease.
If you want to take your Python skills even further, consider checking out Data Projects, interview questions, and non-coding questions on StrataScratch platform.
These resources will provide you with hands-on experience and help you master advanced Python concepts in no time.
See you there!
Share