Understanding Data Types in Python with Examples

Categories
The extensive guide to the most important Python data types. We also included code examples showing you what to do with these data types.
Python is a widely-used programming language. It is often used in data science, data analysis, and machine learning.
One of Python's most significant advantages is its extensive library of pre-built data types, which offers a straightforward and efficient way to manage various types of data in Python.
This article will provide an in-depth exploration of multiple Python data types, examining their novel features, behaviors, and manipulation methods.
Whether you are a novice beginning your journey with Python or a senior developer looking to enhance your understanding of the language, this article offers a comprehensive guide to Python's fundamental building blocks. We gave you an overview of all these building blocks in the advanced Python concepts article.
A lot of those concepts can be practiced in Python interview questions.
What Are Python Data Types?
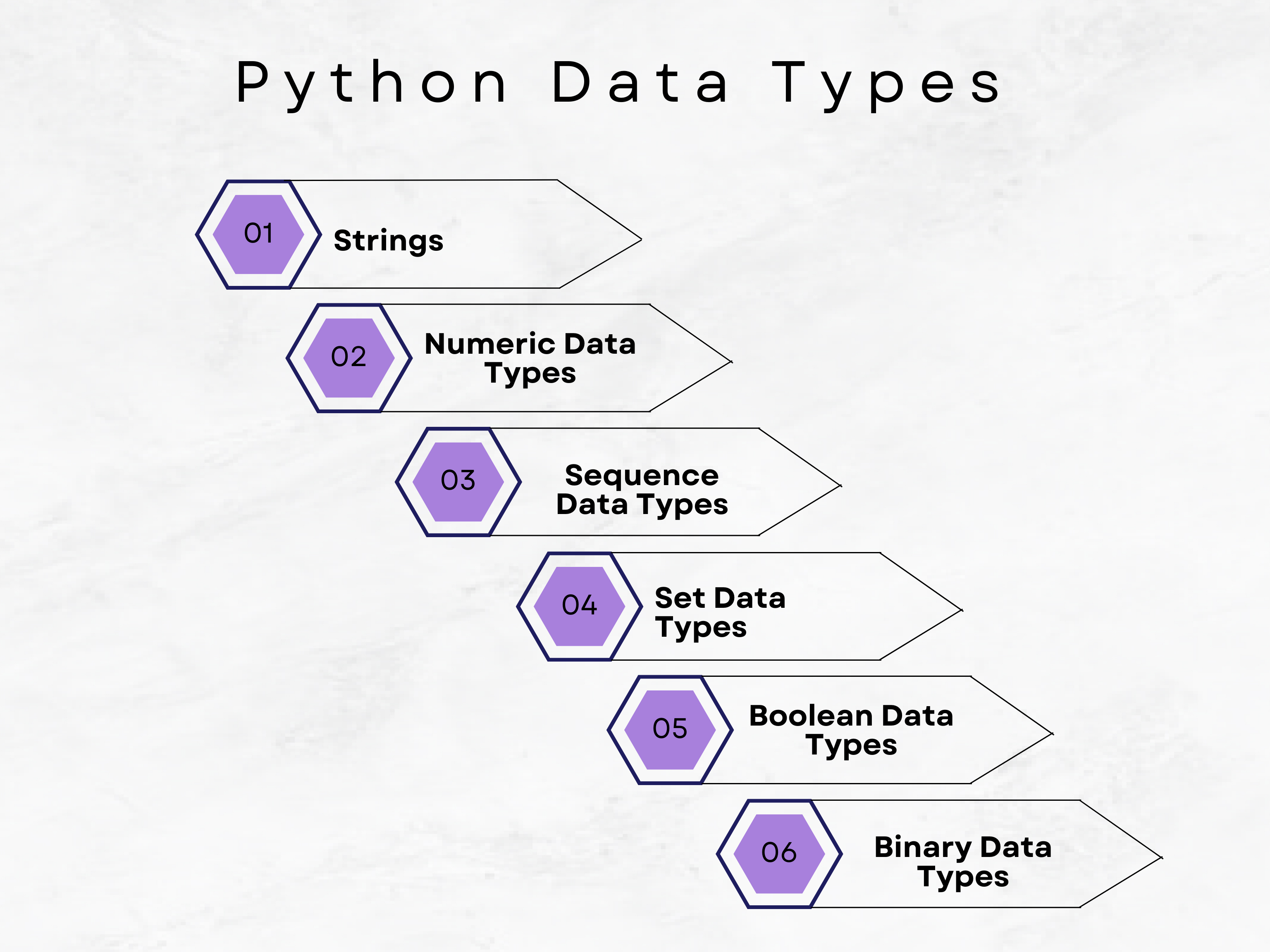
In programming, a data type is a classification of data based on the kind of value it represents.
Python has a variety of built-in data types that you can use to represent different kinds of values.
Some of the most commonly used Python data types, include
- Strings are used to represent text data.
- Here is the documentation.
- Numeric Data Types are used to represent the numbers.
- Here is the documentation.
- Sequence Data Types are used to store collections of items, like list tuples and ranges.
- Here is the documentation.
- Set Data Types are used to store collections of unique values.
- Here is the documentation.
- Boolean Data Types are used to store two possible values, TRUE or FALSE.
- Here is the documentation.
- Binary Data Types are used to represent binary data in Python
- Here is the documentation.
Each data type has its own set of methods and properties that you can use to manipulate and work with the data. Understanding the characteristics of each data type is essential for efficient and effective programming in Python.
String Data Types in Python
Strings are sequences of characters in Python and can be declared using single quotes ('example') or double quotes ("example"). In this section, we will examine the various string operations that can be performed in Python by giving coding examples of each.
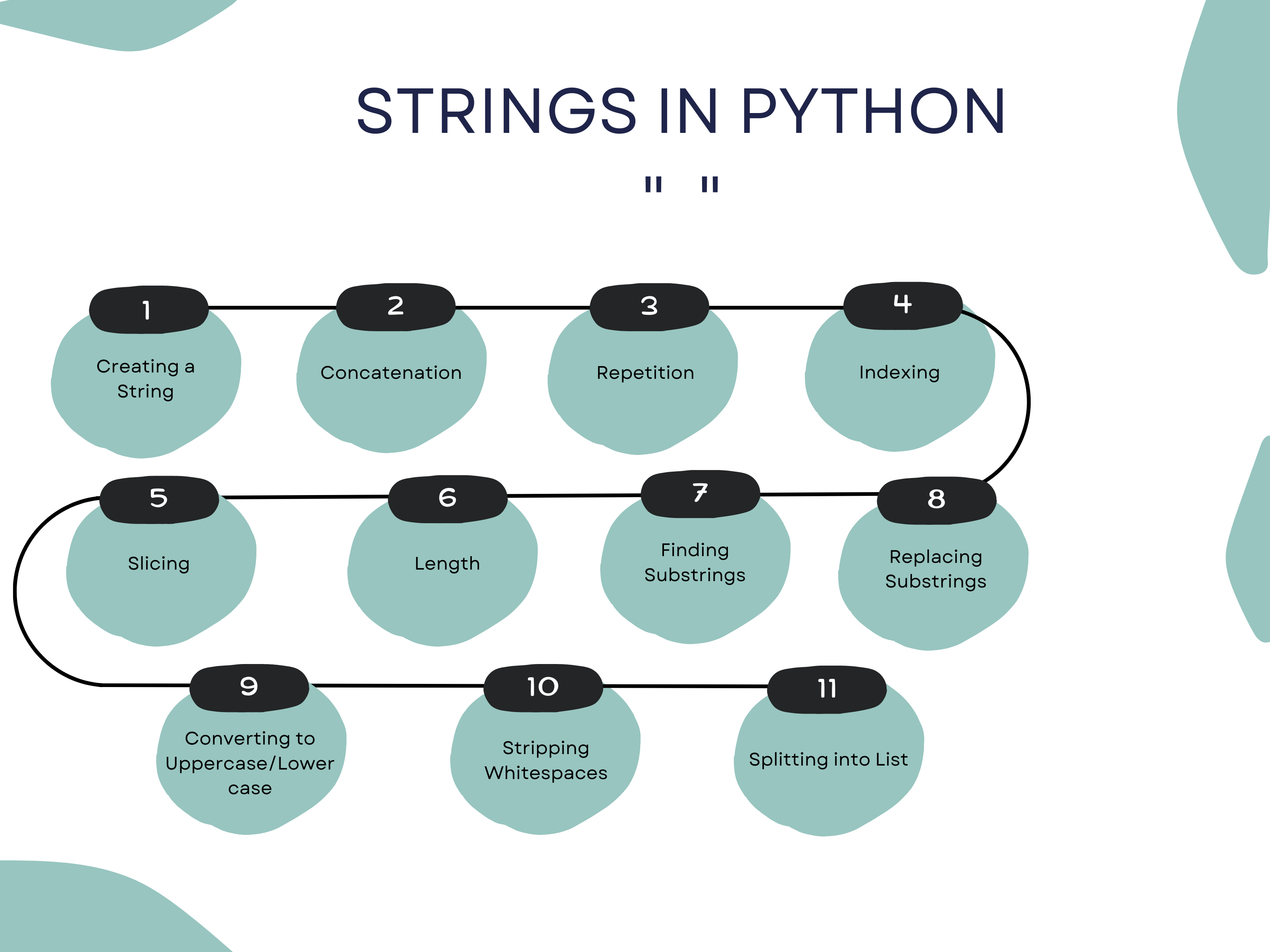
Creating a String
In Python, a string is created by enclosing characters within quotes, either single quotes ('...') or double quotes ("..."). The following code creates a string variable with the value "Hello, World!".
string1 = 'Hello, World!'
print(string1)
Here is the output.

Concatenation
The concatenation of two strings can be performed using the "+" operator.
In this code, two string variables are created with the values "Hello" and "World". The third variable is created by concatenating the two strings.
str1 = "Hello"
str2 = "World"
str3 = str1 + str2
print(str3)
Here is the output.

Repetition
The repetition of a string can be performed by multiplying it by an integer.
This code creates a string variable with the value "Hello" and then multiplies the string to create a new string variable with the value "HelloHelloHello".
str1 = "Hello"
str2 = str1 * 3
print(str2)
Here is the output.

Indexing
In Python, strings are sequences of characters and can be accessed using square brackets "[]" to index individual characters or slices of the string.
In the following code, we create a string variable str1 with the "Hello World" value. We then retrieve the first character from the string by accessing its index.
Python uses 0-based indexing, which means that the first character in a string has an index of 0, the second character has an index of 1, and so on. Therefore, when we write str1[0], we access the character at the first position of string str1, which is "H".
It's important to note that Python uses 0-based indexing for many data types, including lists, tuples, and arrays.
If you're coming from a language that uses 1-based indexing, like SQL, this may take some getting used to.
str1 = "Hello World"
print(str1[0])
Here is the output.

Slicing
In Python, you can use slicing to extract a specific portion of a string by specifying the starting and ending indices. In the given code, a string variable str1 with the value "Hello World" is created, and a portion of the string is retrieved using slicing.
To slice a string, use the syntax string[start_index:stop_index], where start_index is the index of the first character to include in the slice, and stop_index is the index of the first character to exclude from the slice.
In this case, str1[0:5] slices the string str1 to include characters starting from index 0 up to (but not including) index 5. This means that the slice includes the first 5 characters of the string: "Hello".
It's important to keep in mind that the stop index is not included in the slice, and if the start index is omitted, Python assumes that it should start from the beginning of the string. Similarly, if the stop index is omitted, Python assumes it should slice until the end of the string.
Here is the code.
str1 = "Hello World"
print(str1[0:5])
Here is the output.

Length
You can find the length of a string by using the len() function. The length of a string is represented by the number of characters.
The following code creates a string variable with the value "Hello World" and then finds the length of the string.
str1 = "Hello World"
print(len(str1))
Here is the output.

Finding Substrings
The "in" operator checks if a substring is present in a string.
This code below creates a string variable "Hello World" and then checks if the substring "World" is present in the string.
str1 = "Hello World"
print("World" in str1)
Here is the output.

Replacing Substrings
The replace() method can be used to replace a substring in a string.
This code creates a string variable with the value "Hello World" and then replaces the substring "World" with the substring "Universe".
str1 = "Hello World"
str2 = str1.replace("World", "Universe")
print(str2)
Here is the output.

Converting to Uppercase/Lowercase
The upper() and lower() methods can be used to convert a string to uppercase or lowercase, respectively.
This code creates a string variable with the value "Hello World" and then converts the string to uppercase and lowercase.
str1 = "Hello World"
print(str1.upper())
print(str1.lower())
Here is the output.

Stripping Whitespaces
The strip() method can be used to remove whitespaces from the beginning and end of a string.
Here’s the code that creates a string variable with whitespaces at the beginning and end and then removes the whitespaces from the string.
str1 = " Hello World "
print(str1.strip())
Here is the output.

Splitting into List
In Python, the split() method can be utilized to break down a string into a list of smaller substrings based on a specified delimiter.
The provided code initializes a string variable called str1 with the value "Hello World" and then splits the string into a list of substrings using the delimiter " ".
In this particular instance, whitespace is the separator, meaning the string is divided into individual words. The resulting list of substrings is saved in a variable named str_list.
The code used to split the string is str1.split(" "), where " " represents the delimiter utilized to break down the string. This instructs Python to divide the string whenever it detects whitespace.
It's important to remember that the split() method can use any specified delimiter to divide the string into smaller substrings, including commas, semicolons, or other characters.
In the absence of a specified delimiter, the method will utilize whitespace as the default delimiter to split the string.
str1 = "Hello World"
str_list = str1.split(" ")
print(str_list)
Here is the output.

Numeric Data Types in Python
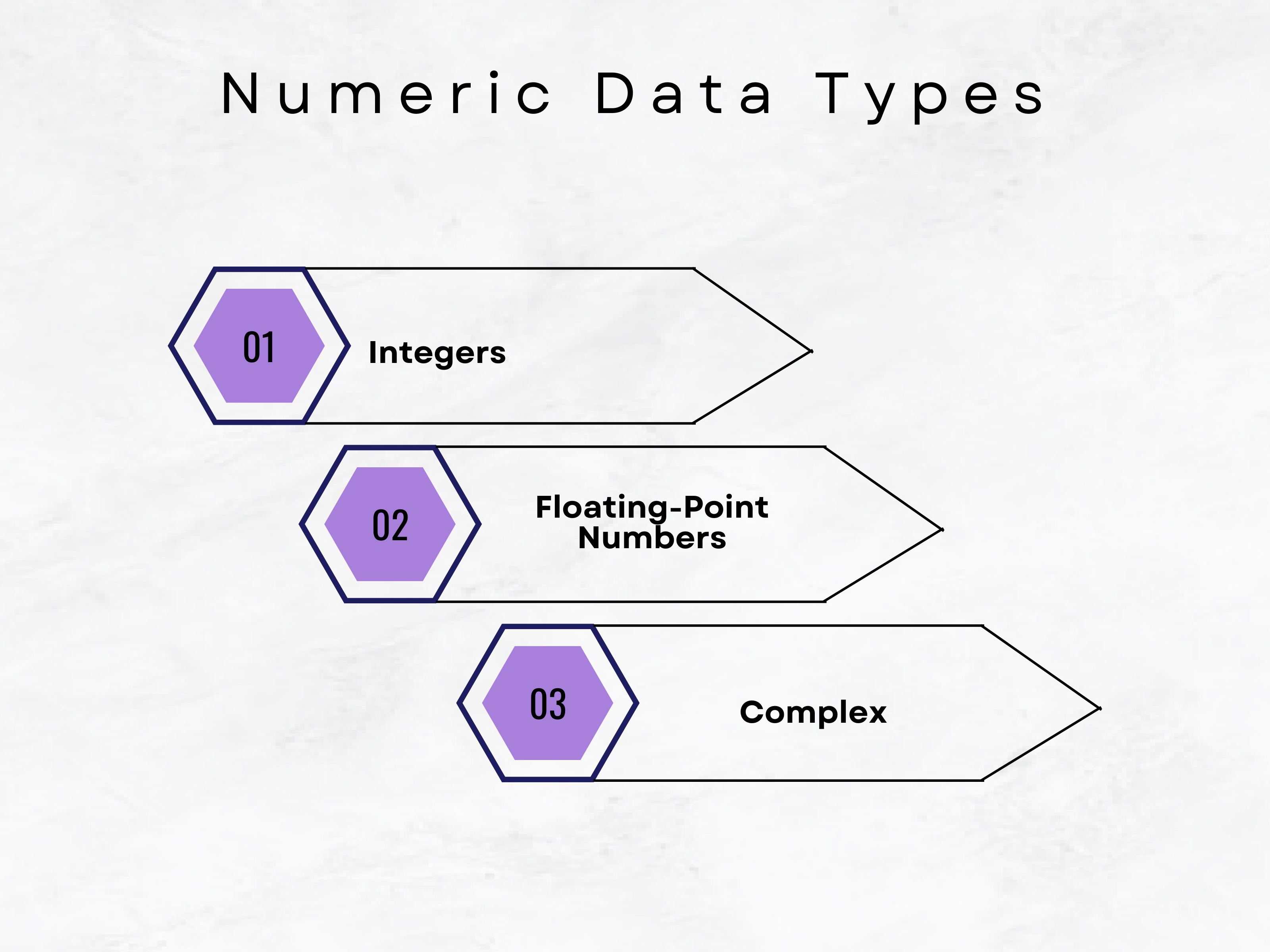
In Python, integers, floating-point numbers, and complex numbers are used to represent different types of numbers.
Integers are numbers without a decimal component.
Floating-point numbers, on the other hand, have a decimal component and are commonly used for scientific and engineering calculations.
Complex numbers are utilized in mathematical calculations requiring the use of imaginary numbers.
In this section, we will examine five different operations that can be performed on the numeric data types in Python.
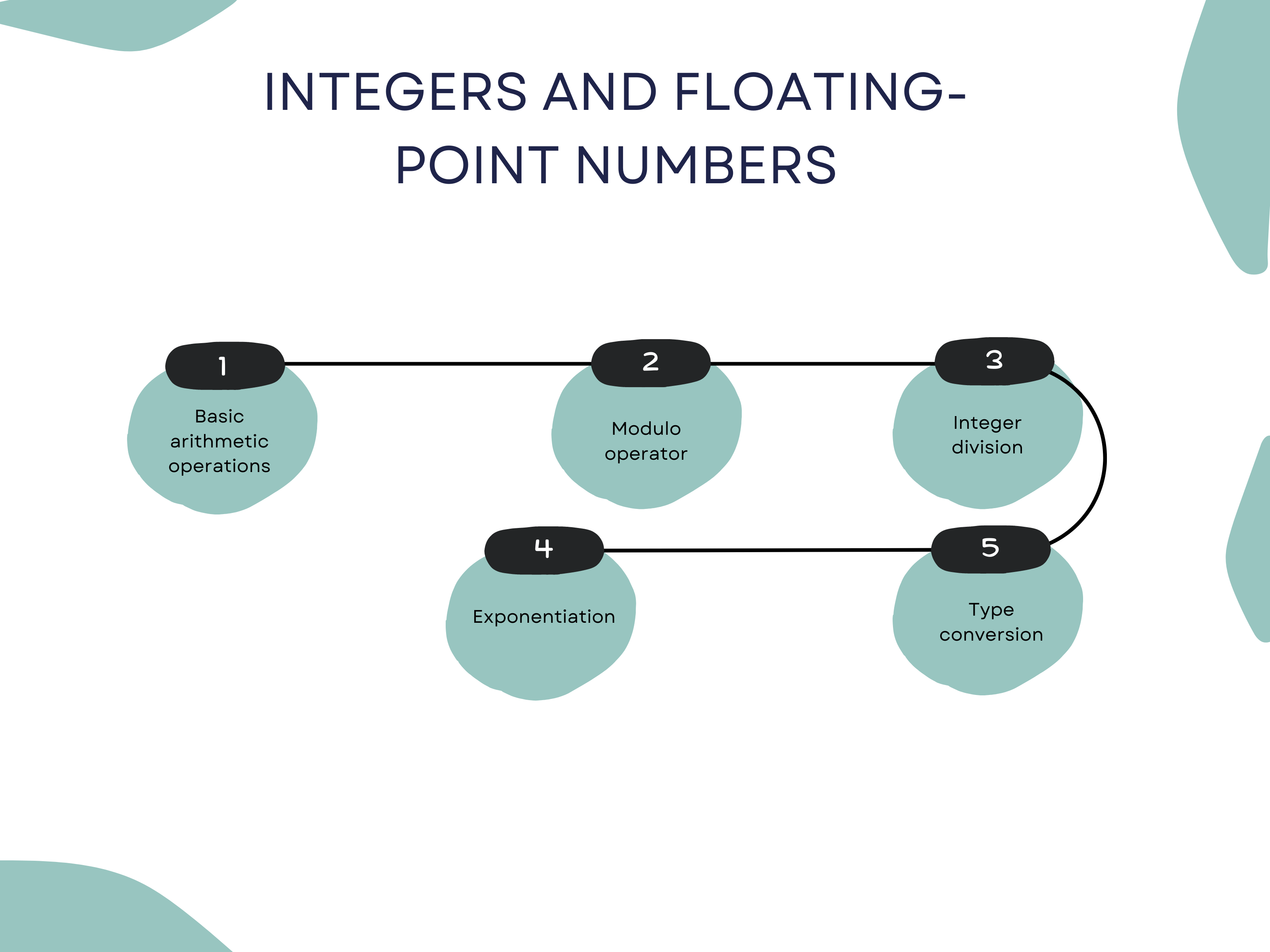
Each operation can be demonstrated through coding examples to better understand its usage and functionality.
Let’s get started with the basic arithmetic operations.
Basic Arithmetic Operations
Basic arithmetic operations include addition, subtraction, multiplication, and division.
The example code performs these four operations.The result of each operation is stored in a variable "c" and printed on the screen.
a = 5
b = 3
# Addition
c = a + b
print(c)
# Subtraction
c = a - b
print(c)
# Multiplication
c = a * b
print(c)
# Division
c = a / b
print(c)
Here is the output.
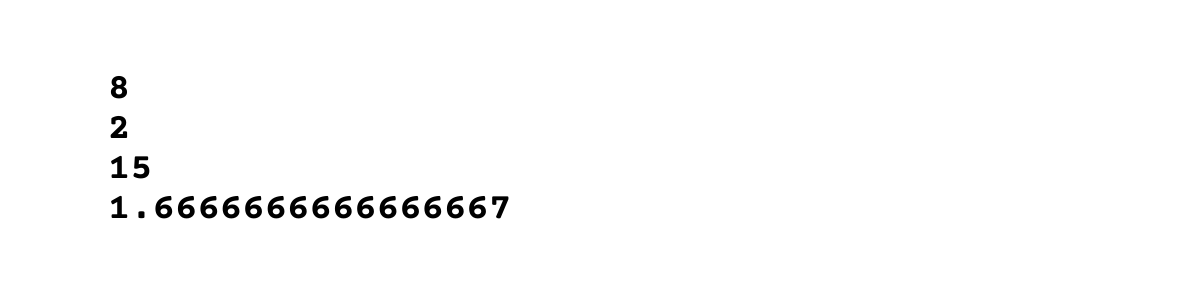
Modulo Operator
Modulo operator returns the remainder after division.
The code calculates the remainder when a number (a) is divided by another number (b) using the modulo operator (%). The result is stored in the variable "c" and printed on the screen.
a = 7
b = 3
c = a % b
print(c)
Here is the output.
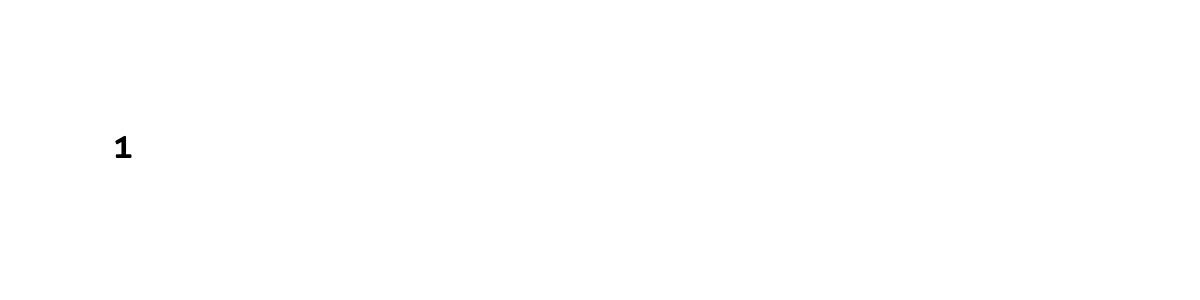
Integer Division
Integer division returns the quotient in integer form, discarding the fractional part.
The code performs integer division between two numbers (a and b). The result is stored in the variable "c" and printed on the screen.
a = 7
b = 3
c = a // b
print(c)
Here is the output.
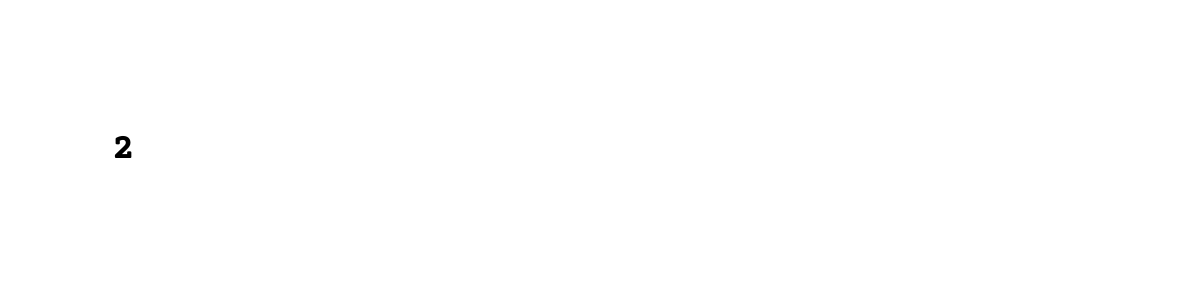
Exponentiation
Exponentiation raises a number to a power.
The following code calculates the exponent of a number (a) raised to another number (b) using the exponentiation operator (**). The result is stored in the variable "c" and printed on the screen.
= 2
b = 3
c = a ** b
print(c)
Here is the output.
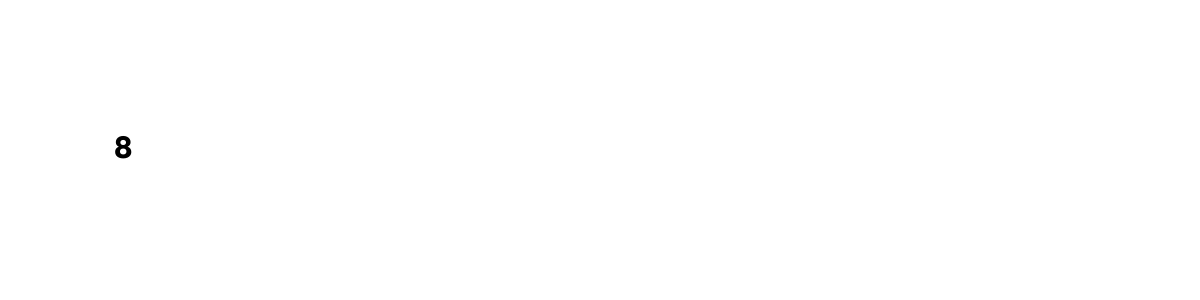
Type Conversion
Type conversion allows for converting a number from one data type to another, such as from integer to float or vice versa.
The code we’ll show demonstrates the type conversion of a number from integer to floating and vice versa.
The float() function converts a number to a floating-point value, while the int() function converts a number to an integer value. Type conversion can be performed separately on the real and imaginary components using these functions for complex numbers.
a = 5
c_num = 3 + 4j
d = float(a)
c_real_float = float(c_num.real)
c_real_int = int(c_num.real)
c_imag_float = float(c_num.imag)
c_imag_int = int(c_num.imag)
print("Float value of a:", d)
print("Float value of the real component of c_num:", c_real_float)
print("Integer value of the real component of c_num:", c_real_int)
print("Float value of the imaginary component of c_num:", c_imag_float)
print("Integer value of the imaginary component of c_num:", c_imag_int)
Here is the output.
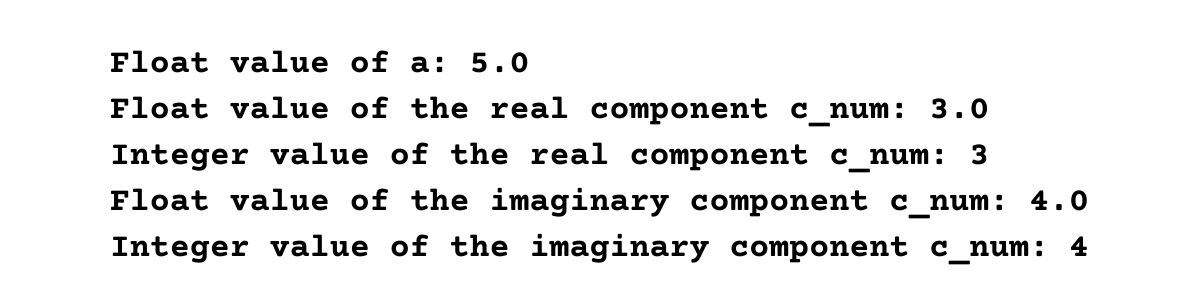
Sequence Data Types in Python
In Python, a sequence data type is utilized as a data structure to store ordered collections of elements.
The three main types of sequence data types in Python are tuples, lists, and ranges.
In this article, we will only focus on tuples and ranges since we’ll dedicate an entire article to lists.
Tuple
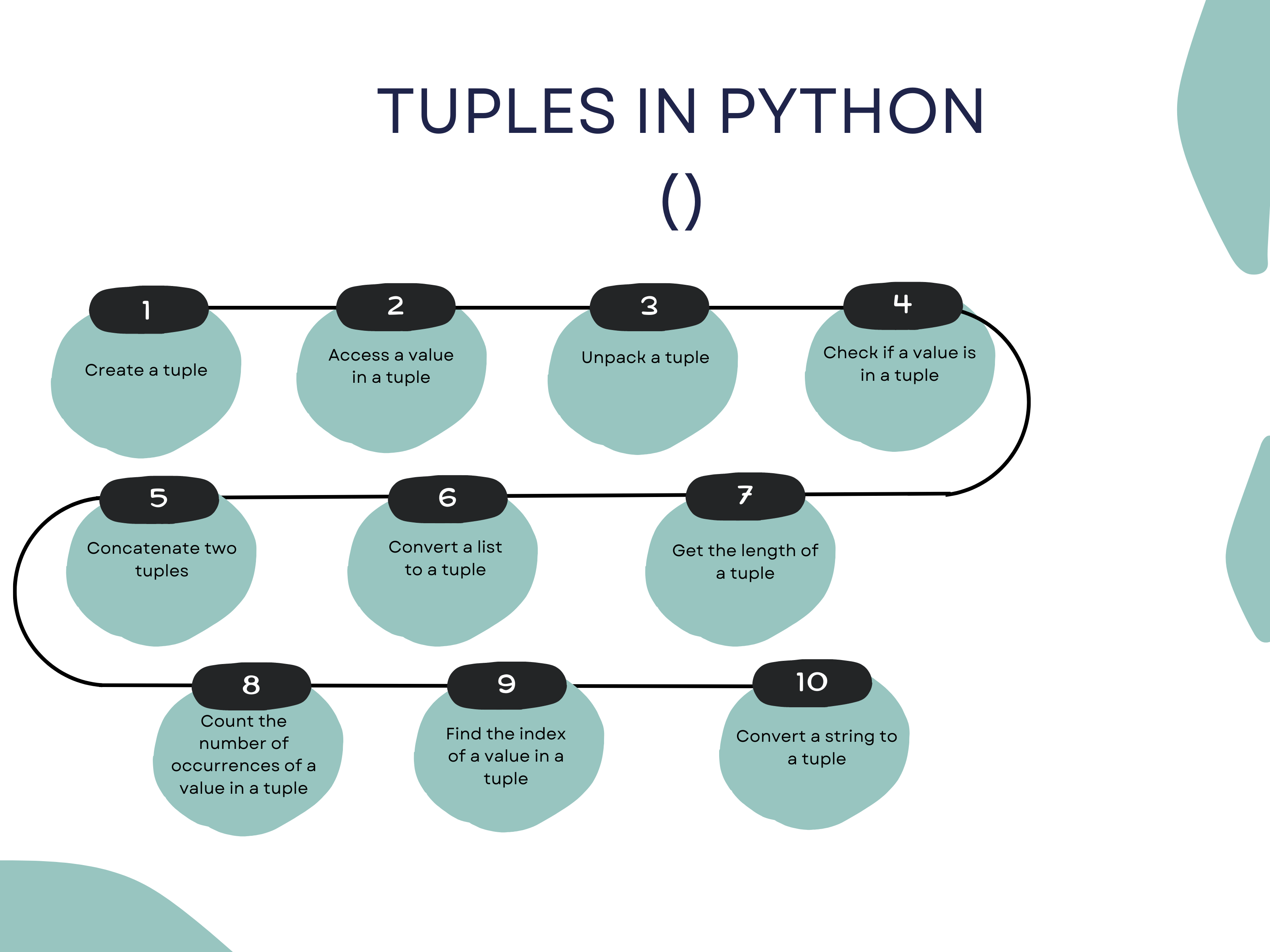
Tuples are immutable sequences in Python that can contain multiple items of different data types.
Create a Tuple
To create a tuple, you can enclose the values in parentheses and separate them with commas.
Here is the code.
my_tuple = (1, 2, 3, 'four', 'five')
my_tuple
Here is the output.

Access a Value in a Tuple
You can access a specific value in a tuple using indexing. In Python, indexing starts at 0, so the first value in a tuple is at index 0, the second value is at index 1, and so on.
Here is the code.
print(my_tuple[3])
Here is the output.

Unpack a Tuple
You can unpack a tuple to assign each value to a separate variable. This is done by listing the variable names on the left side of an assignment statement and the tuple on the right side.
a, b, c, d, e = my_tuple
print(a, b, c)
Here is the output.

Check if a Value Is in a Tuple
You can check if a value is in a tuple using the "in" keyword. If the value is in the tuple, this expression will return True; otherwise, it will return False.
print('four' in my_tuple)
Here is the output.

Concatenate Two Tuples
You can concatenate two tuples to create a new tuple containing all the values from both. This is done using the "+" operator.
my_tuple = (1, 2, 3, 'four', 'five')
my_tuple2 = ('six', 'seven', 8)
new_tuple = my_tuple + my_tuple2
print(new_tuple)
Here is the output.

Convert a List to a Tuple
To convert a list to a tuple, you can use the tuple() function. This creates a new tuple containing all the original list's values.
my_list = [1, 2, 3]
my_tuple = tuple(my_list)
print(my_tuple)
Here is the output.

Get the Length of a Tuple
You can get the length of a tuple using the len() function.
It returns the number of items in the tuple.
print(len(my_tuple)
Here is the output.

Count the Number of Occurrences of a Value in a Tuple
You can use the count() method to count the number of occurrences of a value in a tuple. This method returns the number of times the specified value appears in the tuple.
print(my_tuple.count(2))
Here is the output.

Find the Index of a Value in a Tuple
You can use the index() method to find the index of a value in a tuple.
This method returns the index of the first occurrence of the specified value in the tuple.
print(new_tuple.index('four'))
Here is the output.

Convert a String to a Tuple
You can convert a string to a tuple by using the tuple() function.
This creates a new tuple where each character in the string is a separate value in the tuple.
my_string = "hello"
my_tuple = tuple(my_string)
print(my_tuple)
Here is the output.

Range
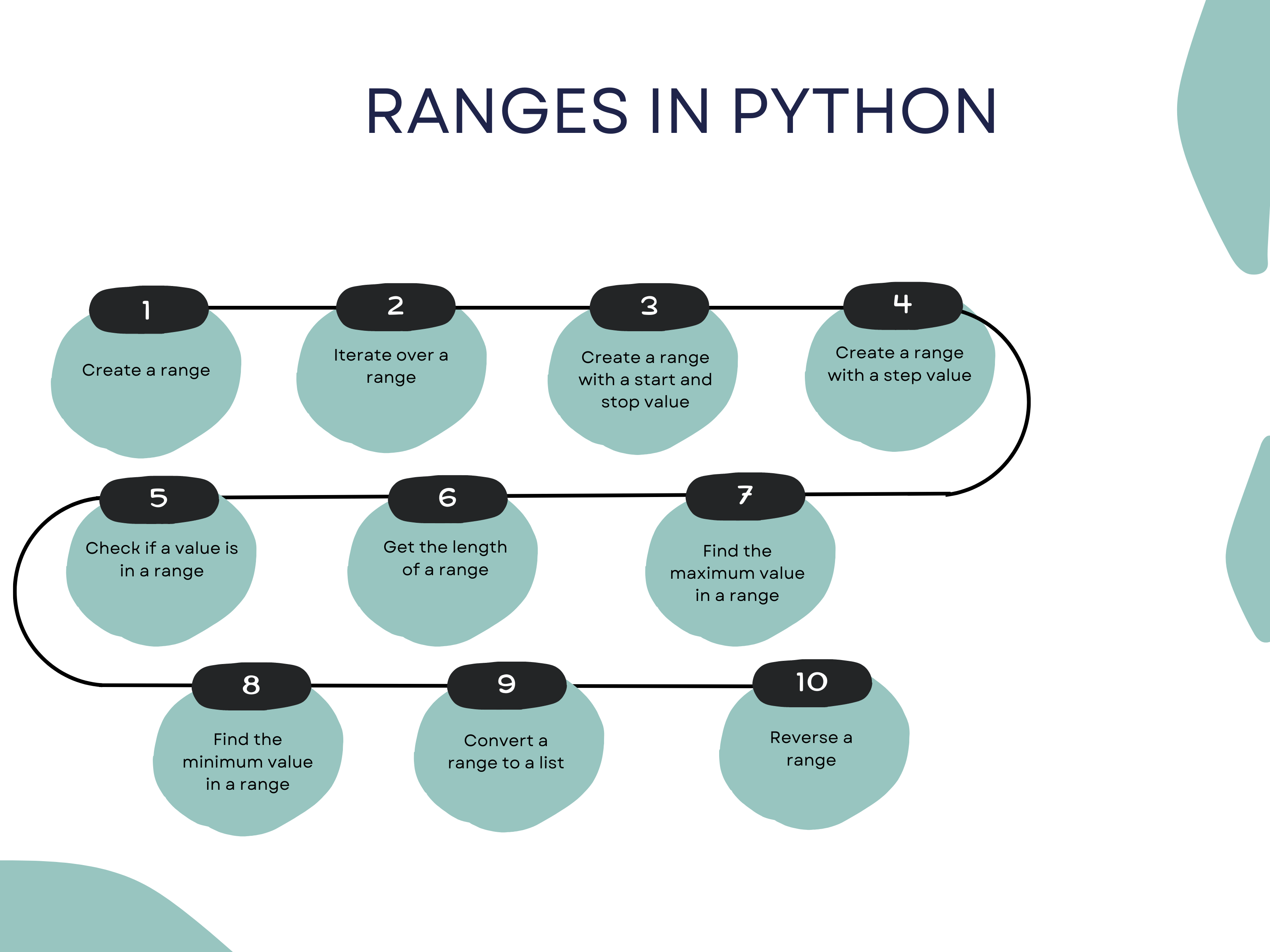
A range is a sequence of numbers that can be used in a for loop to iterate over a sequence of values.
Create a Range
In this example, we create a range called my_range with values from 0 to 9. We do that using the range() function with a stop parameter of 10.
The range() function generates a sequence of numbers from the start parameter (0 by default) up to, but not including, the stop parameter (10 in this case).
Here is the code.
my_range = range(10)
my_range
Here is the output.

Iterate Over a Range
You can use a for loop to iterate over a range and perform a task for each value in the sequence.
The following code uses a for loop to iterate over the values in the range(10) and print each value to the console.
for i in range(10):
print(i)
Here is the output.
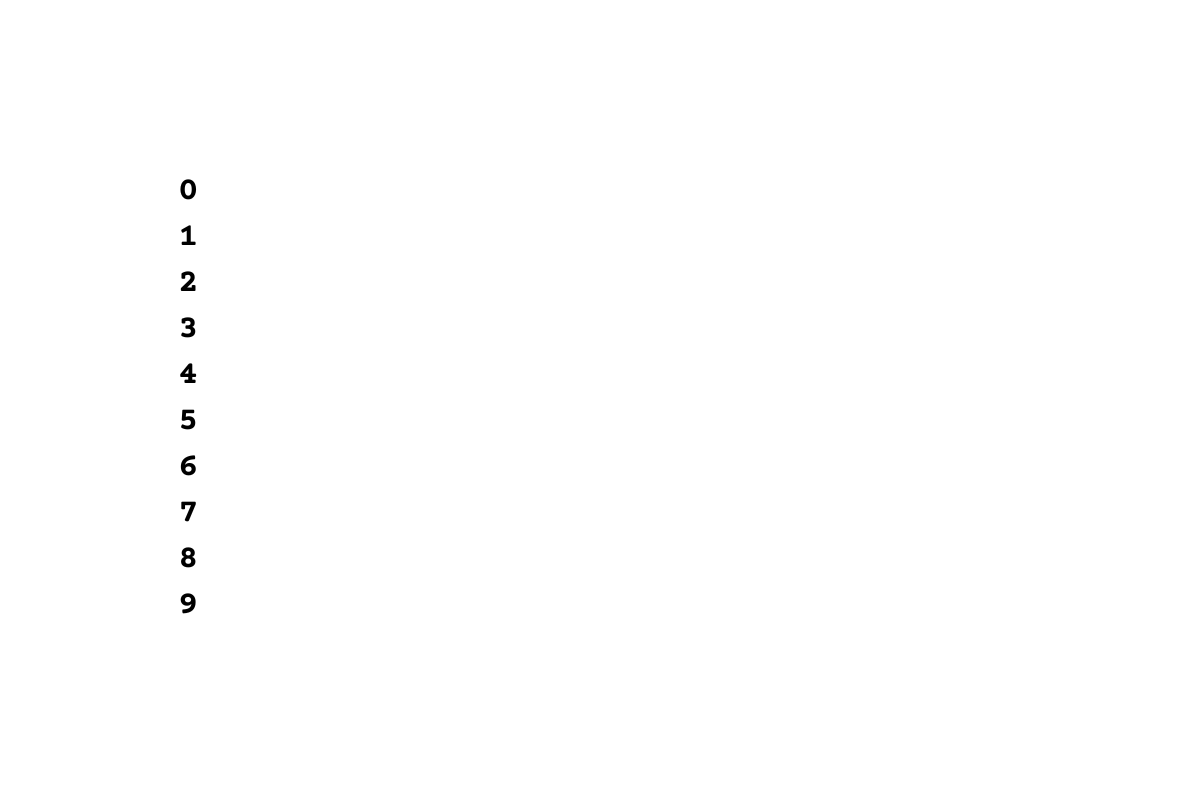
Create a Range With a Start and Stop Value
You can use the range() function in Python to create a range with a specified start and stop value.
The range() function takes two arguments: the start and stop values.
The start value specifies the first number in the range, while the stop value specifies the first number not included in the range.
In this example, my_range is assigned the range of numbers starting at 0 and stopping at 9 (exclusive), meaning that the range includes the numbers from 0 to 8.
The list() function converts the range object to a list, which is then printed to the console.
my_range = range(0, 9)
print(list(my_range))
Here is the output.

Create a Range With a Start, Stop, and Step Value
You can create a range and define the step value in the range() function.
Of the three arguments (start, stop, step), the third argument specifies the step value. This is the amount by which the sequence increases with each iteration.
In the following code, the range starts at 2 and stops at 8, but does not include 8.
The step value is set to 2, meaning the sequence will increase by 2 with each iteration.
This results in the sequence containing the numbers 2, 4, and 6. Then the range will be converted to a list using the list function and printed to the console.
my_range = range(2, 8, 2)
print(list(my_range))
Here is the output.

Check if a Value Is in a Range
You can use the "in" keyword to check if a value is in a range. If the value is in the range, this expression will say True; otherwise, it will be False.
The following code defines a function called check() that checks if a given number is in the range from 0 to 9.
The function prints a message indicating whether or not the number is in the range.
def check(num):
if num in range(10):
print("Yes, {} is in range".format(num))
else:
print("No, {} is not in range.".format(num))
check(5)
check(11)
Here is the output.

Find the Maximum Value in a Range
You can use the max() function to find the maximum value in a range.
The code below uses the max() function to find the maximum value from 0 to 9. The maximum value, which is 9, is printed to the console.
print("The maximum value in a range is {}.".format(max(range(10))))
Here is the output.

Find the Minimum Value in a Range
Use the min() function to find the minimum value in a range. You can find the example in the following code.
It finds the minimum value in the range from 0 to 9. The minimum value, which is 0, is printed to the console.
print("The minimum value in a range is {}.".format(min(range(10))))
Here is the output.

Convert a Range to a List
For converting a range to a list, here’s the list() function. This function creates a new list containing all the range values.
The following code creates a range from 0 to 4 using range(5). The range is then converted to a list using the list() function and printed to the console.
my_list = list(range(5))
print(my_list)
Here is the output.

Reverse a Range
You can reverse a range by using the reversed function.
The code creates a range from 0 to 4 using range(5). The reversed() function then reverses the range, which returns a new range with the values in reverse order.
The reversed range is converted to a list by the list() function and printed to the console.
my_range = range(5)
print(list(reversed(my_range)))
Here is the output.

Set Data Types in Python
The set data type in Python is an unordered collection of unique elements.
Set

A set is an unordered collection of unique elements.
Create a Set
You can create a set by enclosing a comma-separated list of elements in curly braces or using the set() function.
The following code creates a set called my_set with the values 1, 2, and 3. The set is printed on the console.
my_set = {1, 2, 3}
my_set
Here is the output.

Add an Element to a Set
You can add an element to a set using the add() method. If the element is already in the set, nothing happens.
The following code uses the add() method to add the value 4 to my_set. The updated set is printed on the console.
my_set.add(4)
print(my_set)
Here is the output.

Remove an Element From a Set
To remove an element from a set, there’s the remove() method. If the element is not in the set, a KeyError is raised.
This example uses the remove() method to remove the value 3 from my_set. The updated set is printed on the console.
my_set.remove(3)
my_set
Here is the output.

Check if an Element Is in a Set
You can check if an element is in a set using the "in" keyword.
If the element is in the set, this expression returns True; otherwise, it will return False.
The following code uses the "in" keyword to check if the value 2 is in my_set. The result of the expression, which is True, is printed to the console.
print(2 in my_set)
Here is the output.

Check if a Set Is a Subset of Another Set
To check whether a set is a subset of another set, use the issubset() method. If all the elements of the first set are also in the second set, this method will return True; otherwise, it will return False.
The code creates two sets, set1, and set2. It then checks if set2 is a subset of set1 using the issubset() method. The result of the method, which is True, is printed to the console.
set1 = {1, 2, 3, 4}
set2 = {2, 4}
print(set2.issubset(set1))
Here is the output.

Check if Two Sets Have No Common Elements
You can check if two sets have no common elements using the isdisjoint() method. If the sets have no elements in common, this method will return True; otherwise, it will return False.
The code creates two sets, set1, and set2. The isdisjoint() checks if it’s true that the sets have no common elements. The result of the method, which is True, is printed to the console.
set1 = {1, 2, 3}
set2 = {4, 5, 6}
print(set1.isdisjoint(set2))
Here is the output.

Create a Union of Two Sets
The union() method or the "|" operator creates a union of two sets. The output is a new set that contains all the unique elements from both sets.
The following creates the union of the set1 and set2 sets. The resulting set contains all the unique elements from both sets and is printed to the console.
set1 = {1, 2, 3}
set2 = {3, 4, 5}
print(set1.union(set2))
Here is the output.

Create an Intersection of Two Sets
You can create an intersection of two sets using the intersection() method or the "&" operator. This creates a new set containing all the elements common to both sets.
The following code creates two sets, set1 and set2. It then creates an intersection using the intersection() method. The resulting set contains all the elements common to both sets and is printed to the console.
set1 = {1, 2, 3}
set2 = {3, 4, 5}
print(set1.difference(set2))
Here is the output.

Create a Symmetric Difference Between Two Sets
You can create a symmetric difference between two sets using the symmetric_difference() method. The output will contain all the elements that are in either set but not in both sets.
The below example uses the symmetric_difference() method to create a symmetric difference between set1 and set2.
set1 = {1, 2, 3}
set2 = {3, 4, 5}
print(set1.symmetric_difference(set2))
Here is the output.

Frozenset
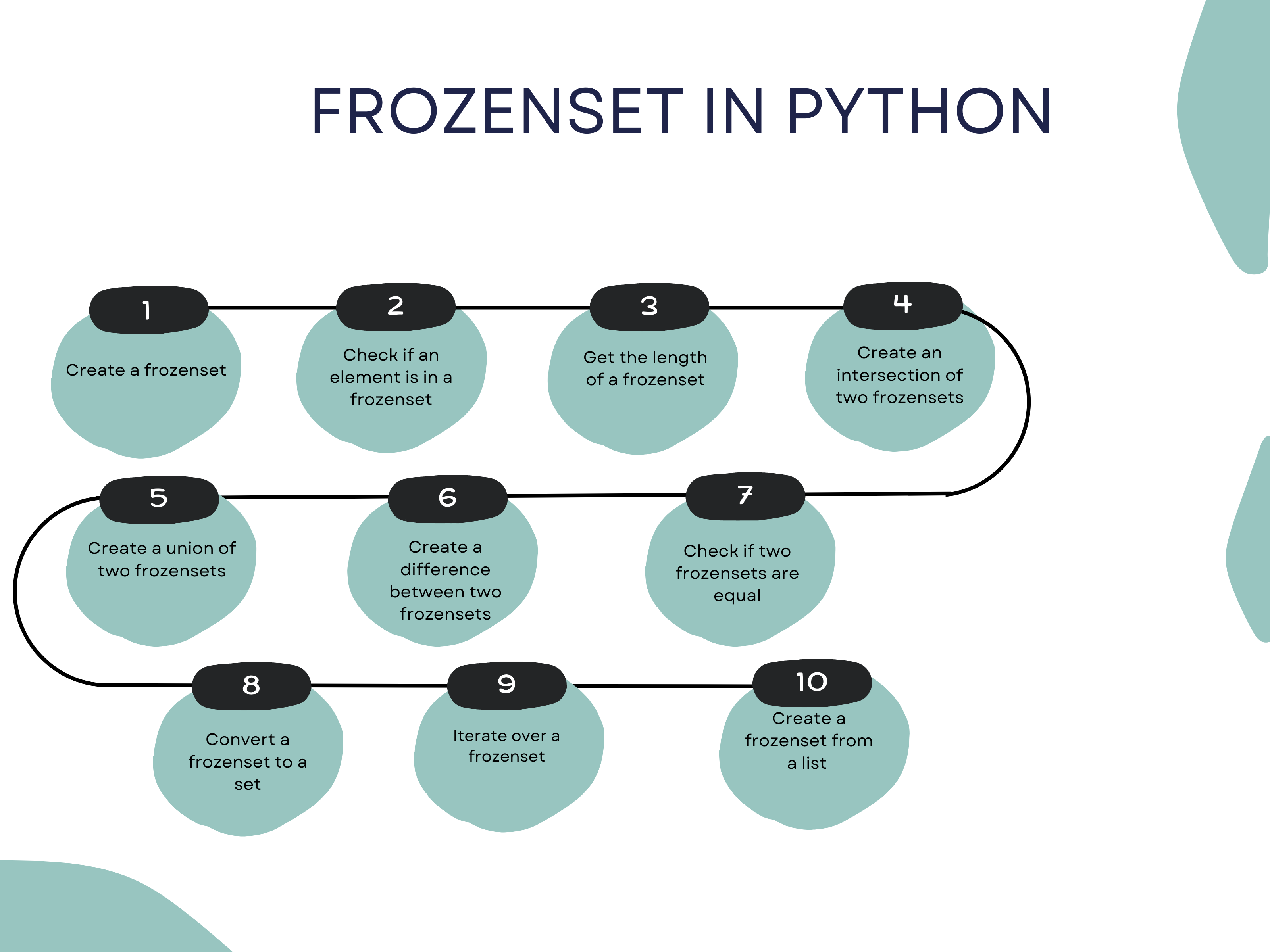
A frozenset is comparable to a set in that it contains a collection of unique elements that are not ordered.
The primary difference is that a frozenset is unchangeable and cannot be altered after its creation. In contrast, a set is mutable and allows for modifications to its elements.
Create a Frozenset
The following code creates a frozenset using the frozenset() constructor.
my_frozenset = frozenset({1, 2, 3})
my_frozenset
Here is the output.

Check if an Element Is in a Frozenset
The "in" keyword is used to check if an element is in the frozenset.
print(2 in my_frozenset)
Here is the output.

Get the Length of a Frozenset
The len() function is used to get the frozenset’s length.
print(len(my_frozenset))
Here is the output.

Create an Intersection of Two Frozensets
The intersection() method is used to create an intersection of two frozensets.
set1 = frozenset({1, 2, 3})
set2 = frozenset({3, 4, 5})
print(set1.intersection(set2))
Here is the output.

Create a Union of Two Frozensets
To create a union, use the union() method like in the below example.
set1 = frozenset({1, 2, 3})
set2 = frozenset({3, 4, 5})
print(set1.union(set2))
Here is the output.

Create a Difference Between Two Frozensets
The example shows how to use the difference() method to create a difference between two frozensets.
set1 = frozenset({1, 2, 3})
set2 = frozenset({3, 4, 5})
print(set1.difference(set2))
Here is the output.

Check if Two Frozensets Are Equal
The "==" operator is used to check if two frozensets are equal.
set1 = frozenset({1, 2, 3})
set2 = frozenset({3, 2, 1})
print(set1 == set2)
Here is the output.

Convert a Frozenset to a Set
Frozenset can be converted to a set using the set() constructor.
my_set = set(my_frozenset)
print(my_set)
Here is the output.

Iterate Over a Frozenset
A frozenset can be iterated over a frozenset using the for loop. An example of how to do this is shown below.
for i in my_frozenset:
print(i)
Here is the output.
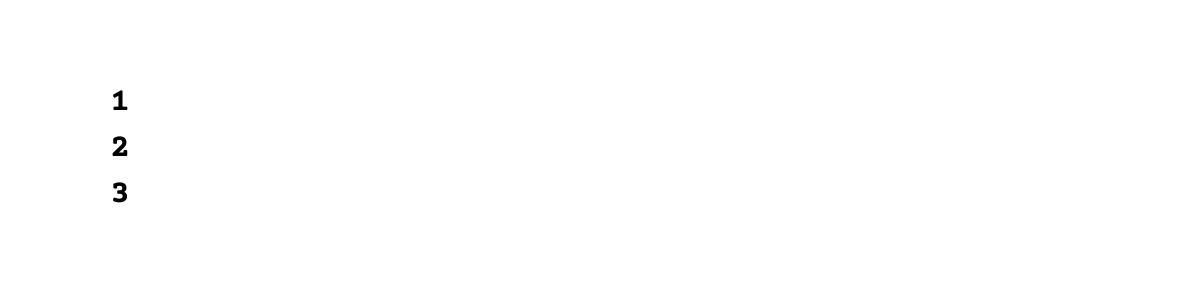
Create a Frozenset From a List
The frozenset() constructor can create a frozenset from a list like this.
my_list = [1, 2, 3]
my_frozenset = frozenset(my_list)
print(my_frozenset)
Here is the output.

Boolean Data Type
The Boolean data type in Python is a data type that represents one of two possible values: True or False. It is used for logical operations and conditional statements.
Now, let’s see how they are used in Python.
In this example, the expression x > y returns True because 10 is greater than 5. The resulting value of True is then assigned to the variable z, which is printed to the console.
Here is the code
x = 10
y = 5
z = x > y
print(z)
print(list(reversed(my_range)))
Here is the output.

Binary Data Type
The binary data types in Python are used to represent binary data comprising sequences of 0s and 1s. There are three main binary data types in Python: bytes, bytearray, and memoryview.
Bytes
The bytes data type is an immutable sequence of bytes representing a sequence of binary data. It stores data that should not be changed, such as the contents of a file or network message.
Creating Bytes Object
You can create a bytes object in Python by prefixing a string with a "b" or calling the bytes() constructor.
Here is an example code that creates a bytes object.
my_bytes = b'Hello'
my_bytes
Here is the output.

Indexing and Slicing a Bytes Object
You can access individual elements in a bytes object using indexing and slicing. The indexing starts from 0 and goes up to the length of the bytes object minus one.
Slicing works the same way as it does for other sequence types.
Here is an example code that demonstrates indexing and slicing.
my_bytes = b'Hello'
print(my_bytes[0])
print(my_bytes[1:3])
Here is the output.

Converting a Bytes Object to a Bytearray
A bytearray is a mutable sequence of bytes, and it is possible to convert a byte object to a bytearray using the bytearray() constructor.
An example code demonstrates converting a bytes object to a bytearray.
my_bytes = b'Hello'
my_bytearray = bytearray(my_bytes)
my_bytearray
Here is the output.

Converting a Bytes Object to a List
You can also convert a bytes object to a list using the list() constructor.
An example code demonstrates converting a bytes object to a list.
my_bytes = b'Hello'
my_list = list(my_bytes)
my_list
Here is the output.

Bytearray
The bytearray data type is similar to bytes but is mutable and allows for modification of its elements.
Let’s look at them in more detail.
Creating a Bytearray Object
A bytearray object is created using the built-in bytearray() function. This function takes a bytes-like object as an argument and returns a mutable bytearray object.
In this example, a bytearray object is created from the bytes object b'Hello'.
my_bytearray = bytearray(b'Hello')
my_bytearray
Here is the output.

Modifying a Bytearray Object
A bytearray object can be modified using different methods.
In this example, the first letter of the bytearray is changed from 'H' to 'G' by using replace method.
# Change the first letter from h to g
my_bytearray = bytearray(b'Hello')
print(my_bytearray)
my_bytearray = my_bytearray.replace(b'Hello', b'Gello')
print(my_bytearray)
Here is the output.

Converting a Bytearray Object to a Bytes Object
A bytearray object can be converted to a bytes object using the built-in bytes() function. This function takes a bytes-like object as an argument and returns an immutable bytes object.
In this example, the bytearray object my_bytearray is converted to an immutable bytes object my_bytes.
my_bytearray = bytearray(b'Hello')
my_bytes = bytes(my_bytearray)
my_bytes
Here is the output.

Converting a Bytearray Object to a List
A bytearray object can be converted to a list using the built-in list() function. This function takes a bytearray as an argument and returns a list object.
In this example, the bytearray object my_bytearray is converted to a list object my_list.
The list contains the ASCII values of the individual characters in the bytearray.
my_bytearray = bytearray(b'Hello')
my_list = list(my_bytearray)
my_list
Here is the output.

Memoryview
The memoryview data type is a way to view the contents of bytes or bytearray objects as a mutable sequence of bytes. It allows for the efficient manipulation of large amounts of binary data without making copies of the data.
Creating a Memoryview Object
A memoryview object is created using the built-in memoryview() function. This function takes a bytes-like object as an argument and returns a memoryview object that allows you to access and manipulate the underlying bytes.
In this example, a memoryview object is created from the bytes object b'Hello'.
my_bytes = bytes(b'Hello')
my_view = memoryview(my_bytes)
my_view
Here is the output.

Accessing and Modifying Bytes in a Memoryview Object
You can access and modify individual bytes in a memoryview object using indexing and assignment.
In this example, the first byte of the memoryview object my_view is changed from 'H' to 'G' by accessing its first element using indexing and assigning the value 71, which is the ASCII value of 'G'.
my_bytes = bytearray(b'Hello')
my_view = memoryview(my_bytes)
my_view[0] = 71
my_view
Here is the output.

Slicing a Memoryview Object
You can slice a memoryview object to create a new memoryview object that references a portion of the original memoryview object.
In this example, a new memoryview object my_slice references bytes 1 and 2 of the original memoryview object my_view.
my_bytes = bytearray(b'Hello')
my_view = memoryview(my_bytes)
my_slice = my_view[1:3]
print(my_slice)
Here is the output.

Converting a Memoryview Object to a Bytes Object
You can convert a memoryview object to a bytes object using the built-in bytes() function. This function takes a bytes-like object as an argument and returns an immutable bytes object.
In this example, the memoryview object my_view is converted to the immutable bytes object my_bytes_copy.
my_bytes = bytearray(b'Hello')
my_view = memoryview(my_bytes)
my_bytes_copy = bytes(my_view)
my_bytes_copy
Here is the output.

Conclusion
In this article, we've covered the basics of Python's built-in data types and their uses.
We explored the characteristics and behaviors of each Python data type, as well as the methods and operations that you can use to manipulate and work with them.
Mastering these fundamental building blocks of Python programming gives you a solid foundation to build more complex applications that handle different kinds of data.
Understanding the strengths and limitations of each Python data type can help you choose the right data structure for your problem and optimize your code for efficiency and performance.
Python data types are a crucial component of the language, and mastering them is an essential step toward becoming a proficient Python developer.
Whether you're working on web development, data analysis, machine learning, or any other application, the skills you've learned in this article, along with the resources available on the platform, will be a valuable asset to your programming toolkit.
