Python Programming Standards You Should Know and Apply
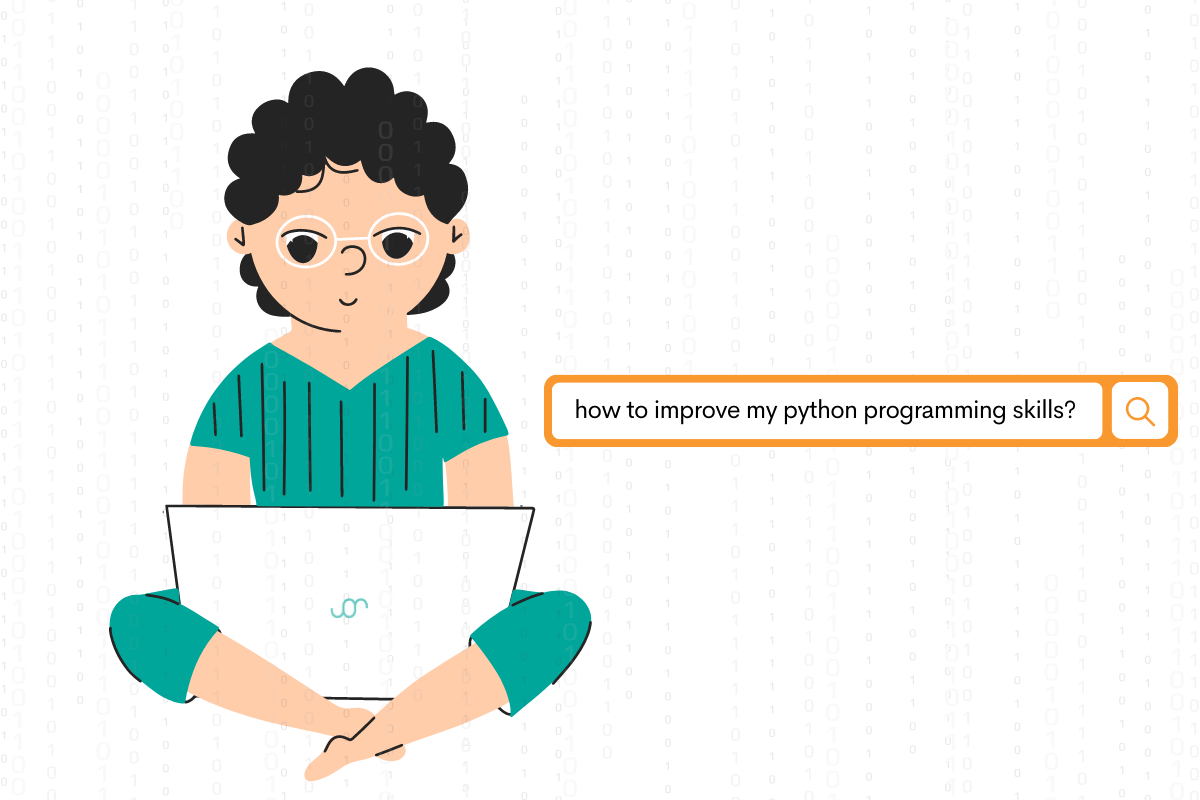
Categories
In this article, we will cover some of Python applications, its best practices and standards. You need to apply them to improve your Python programming.
Coding is an art of communicating with computers and developing programs/applications that help humans to ease their life. It helps the developers to provide the input to the computers through programs and get the desired output.
Coding can be compared with the natural languages that we speak, for example English. Just the way English has certain grammatical rules, coding also has semantic and syntactic rules that the developers need to learn. There are many styles in which the same algorithm can be written. When a team of developers work together on a specific problem, they might write the code in different ways and thus, consistency and readability becomes critical in writing any program. Thus, developers follow certain rules or standards that are used widely in any programming language.
In this article, we will look at some of the best practices in the Python programming language so that you are aware of the Python programming standard in your job or when answering Python interview questions.
What is Python?
Python is one of the most widely used and a popular programming language today. It was developed by Guido van Rossum which was released in 1991. Python is used in many fields due to its variety of applications:
- Mathematics
- Data Science and Machine Learning
- Web Development
- Software Development
- Blockchain Technology
- Scripting Language
- Data Engineering, etc.
Python was mainly designed for its readability and has some similarities to the English language and with some influence from the field of mathematics. Due to its ease of use and readability, it has become the most widely used language among most of the industries today. Due to its popularity, a lot of people have started using it and thus, the open source community of Python has increased exponentially. This led to the development of many Python libraries that users can use directly with minimal effort. We have created a Python cheat sheet that will help the users in navigating through all the functions that are used by Data Scientists along with their applications.
In the next section, we will see some of the examples where Python is widely used and what it can do.
What Python Programming Can Do
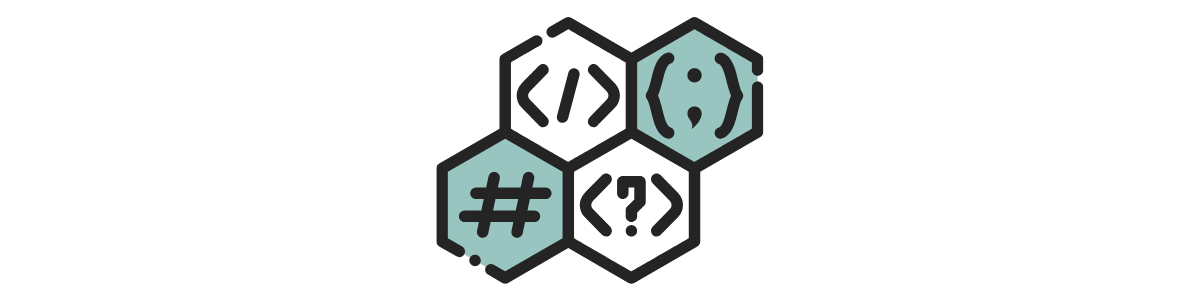
Due to its simplicity and ease of use, Python is used in many industries including data analytics, machine learning, AI, web development, game development, software engineering, etc. We will be discussing only a few examples below but there are a large number of examples where Python can be used.
Machine Learning and AI
Python is usually the first choice among Data Scientists and Data Analysts for machine learning due to its ease of use, readability and adaptability. Thus, most of the engineers prefer this language for implementing machine learning and AI algorithms. Since this is an open source language, it has a great community of developers who has build many modules for various types of algorithms and thus, it becomes easy for Data Scientists to use these models directly without writing the logic from scratch.
Data Analytics and Visualisation
Data analytics and Data Visualisation is another quickly evolving subject that makes use of Python programming, much like AI and machine learning. People who can gather, manipulate, and organize information are needed at a time when we are producing more data than ever before. Python is helpful for manipulating data and doing repeated operations while working with enormous amounts of information.
Application/Software Development
Python is used in almost all the industries and many types of applications can be written using this language. Python may be used to create any type of program, including blockchain applications, audio and video applications, and machine learning applications.
Why use Python?
- Python is very easy to read since it's simply written in the English language and there are many references to this language.
- Python does not need to employ semicolons or parentheses as in the other languages. Thus, syntax becomes a bit easy to follow
- Indentation, which utilizes whitespace, is how Python defines scope, including the scope of loops, functions, and classes. In other languages, usually a series of curly brackets are used for this purpose.
Best Practices for Writing Industry Standard Python Code
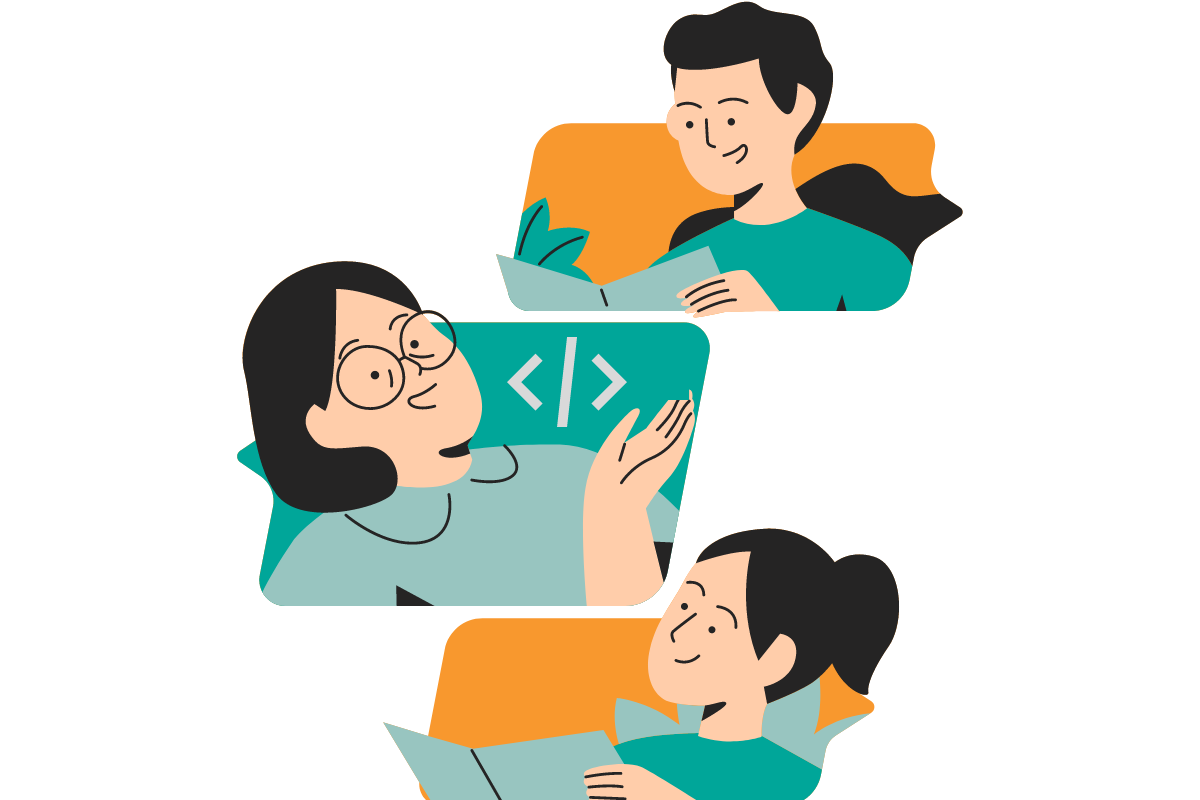
In this section, we will discuss some of the best practices that everyone must know while writing a Python code.
Python, being one of the most popular languages today, is known for its simplicity, readability and collaborative use. If you are someone who is very new to the Data Science field or has been around in this field for a while, these Python best practices would help you boost in your career and also make your teammate’s life easier for readability. Coding is an art, so you are the artist who will make wonders if you follow best practices in Python.
Indentation:
Python needs to have indentation of the code in order to run successfully. Indentation can be done by using some spaces at the start of the code line. It is a requirement in this language and thus, it’s mandatory to follow the rules of indentation. Below is the example of indentation. In the “for” loop, the condition “if x%2 == 0” is indented by giving 4 spaces after the for loop. If we don’t give these spaces, the code will throw an error. Due to this indentation, it becomes easy to read Python code.
Python Code:
for x in range(10):
if x%2 == 0:
print("{} is an even number".format(x))
else:
print("{} is an odd number".format(x))
In Python, indentation is mandatory. For example, in the above code if you write the if statement exactly below the for loop, then the Python code will throw an indentation error. Due to indentation, it’s easier to understand and read the code.
Maximum Line Length
Python was developed in such a way that readability and cleanliness would be at the center of every code. Thus, in Python, the maximum number of characters in one line cannot exceed 79. These are requirements of a standard Python library. Due to this limit, Python codes are often easier to read and you can work on multiple Python files side by side due to this limit.
Line Breaks
Instead of writing a long line or a lengthy line of code, you can split that line across multiple lines to enable better readability. All the expressions can be placed in the parentheses and each expression can be written onto a different line. There can be different types of line breaks.
For example, if you are writing a text and assigning it to a variable, you can use backslashes for breaking up the lines. In the case of formulas, the best method is to write each expression in a separate line. Below is the screenshot of bad practice of writing long lines of code versus the best practice is to do it.
Bad Practice:
# Without using line breaks
Total_Cost = Rental_Cost + Furniture_Cost + Electricity_Cost + Resourcing_Cost + Gas_Cost
Good Practice:
# Use Line breaks
Total_Cost = (Rental_Cost
+ Furniture_Cost
+ Electricity_Cost
+ Resourcing_Cost
+ Gas_Cost)
From the above screenshots, it is evident that using line breaks makes it easier to read than writing all the expressions in a single line.
Importing Libraries
When you are working with Python, it’s recommended that you import all the Python libraries, classes at the start of the program. This allows the user to check what libraries are being used in the program and see if any dependencies are needed or not. Also, it’s not ideal to import a library just above the function call from that library. Ideally, you should import all the libraries in separate lines but if you need to import multiple modules from the same library, it’s okay to mention those in the same line.
Bad Practice:
# Import your Libraries in same line
import pandas as pd, numpy as np
Good Practice:
# Import your Libraries in separate lines
import pandas as pd
import numpy as np
# Import multiple modules from the same library in one line
from sklearn.metrics import confusion_matrix, classification_report
Commenting the Code
The cornerstone of sound coding procedure is the comment. It's crucial to thoroughly document your Python code and to update all of the comments whenever the code is modified.
Complete sentences, preferably in English, should be used while leaving comments. There are three different types of comments used in any programming language:
1. Inline Comments - These comments are written on the same line as that of the Python code. These comments are useful when you are performing a certain operation and need to make sure that the code reviewer understands what that specific operation is doing. For example, if you are replacing the missing values from a certain column with 95th percentile value, it’s easier to write the comment inline with the function. The comments are started by #. Inline comments are shown in the screenshot below.
# Import your Libraries in same line
import pandas as pd, numpy as np
# Calculate addition of x and y and store in z
z = x + y
2. Block Comments - These comments typically have one or more paragraphs. These are also started with # but if you need to have comments in multiple lines, then use # in different lines. It’s a good idea to give a space after # before you start writing the actual comment.
# This is a comment
# written in
# more than just one line
print("Hello, World!")
3. Docstrings or Document Strings - These types of comments are typically used when there is an explanation needed. It’s used before a class, module or a function in Python. These comments are surrounded by “””Three Double Quotes”””.
"""
This is the docstring comment usually used to describe a function, class or a module. We can write the comments in multiple lines by using three double quotes.
"""
print("Hello, World!")
Summary
In this article, we learnt about what Python is, how it can be useful in today’s world and what are the best practices for using Python programming language. Once you go through this article, you will realize all the other blog posts on Python are written with the standards that we discussed today on the StrataScratch platform.
There are many blog posts on StrataScratch around Python programming covering concepts like types of pandas joins in Python, Python functions, machine learning projects, etc. and all of them follow best practices of programming. If you want to practice SQL or Python programming for your next interview, go through practical coding questions on the platform which will help you in gaining confidence.
All the best!
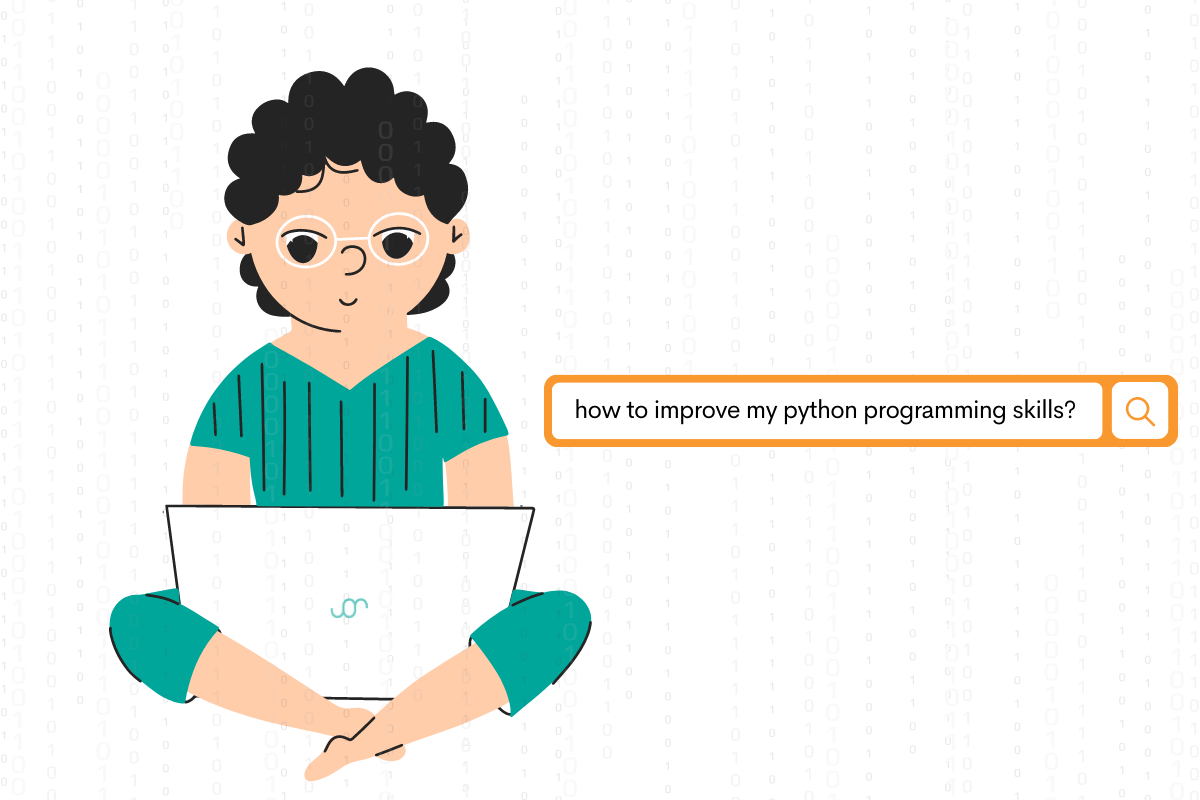