The Python List Methods I'll Use As a Data Scientist
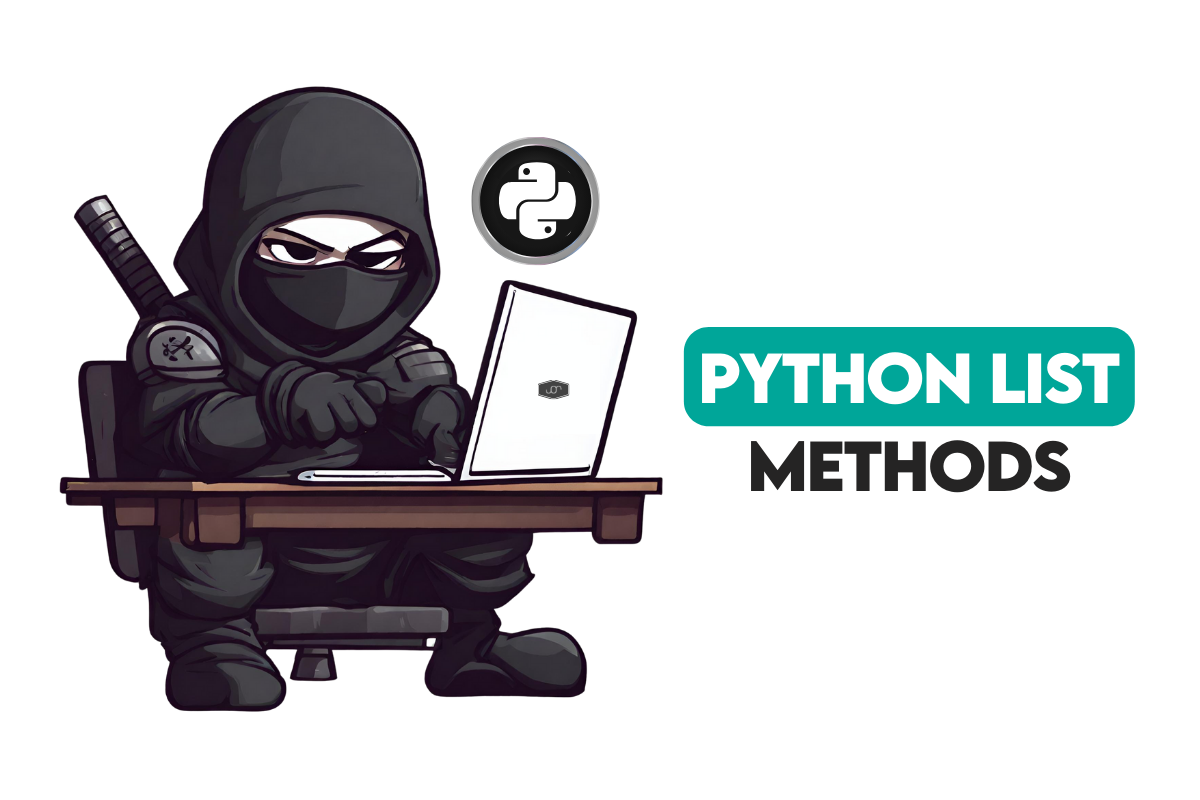
Categories
Master Python list methods: advanced techniques in list manipulation, data analysis, and efficient coding practices
Have you ever wondered how data manipulation and analysis in Python may be centered on something as basic as a list?
Discovering Python list methods opens us to possibilities, ranging from simple operations like element addition and removal to complex ones like matrix manipulations and list comprehensions.
I'll walk you through the nuances of Python lists, highlighting methods that improve programming efficiency and simplify data handling.
What is a Python List?
A Python list methods similarly to a container for a group of objects. Any item, including strings, numbers, and other lists, may be one of these. It's comparable to how various things may be stored in a box.
You can search through the list, add items, and remove items. Consider it as an extensible basket that you may fill and empty.
Basic List Operations
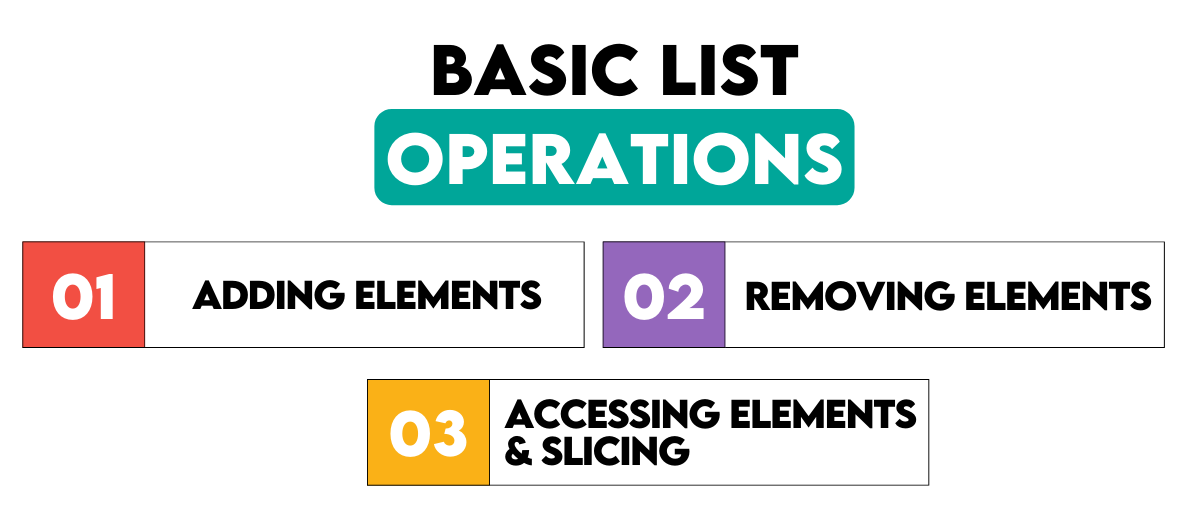
In this section, we will see different methods to add/ remove or access elements of lists. Let’s start with methods to add elements.
Adding Elements
The append() method may be used to add an item at the end of a list, or insert() can be used to add an item at a given location. The best method for adding numerous things is to use extend().
Now, let’s see the code.
First, let’s define a list and then add one element by using append(). Let’s start.
fruits = ['apple', 'banana']
# Adds at the end
fruits.append('cherry')
fruits
Here is the output.

Next, let’s use the insert() method to add an element in a given position. Let’s see the code.
# Inserts at position 1
fruits.insert(1, 'orange')
fruits
Here is the output.

Now, let’s use our final method, extend(). Here is the code.
# Adds multiple items
fruits.extend(['date', 'elderberry'])
fruits
Here is the output.

- append() and extend() are generally efficient for their purposes. append() has a constant time complexity, making it very efficient for adding single items.
- insert(): It might not be as effective for long lists because all the following components in the list might need to be shifted to make room for the new item.
- extend() is more efficient than using append() in a loop to add multiple elements because it minimizes the overhead of repeatedly resizing the list.
Removing Elements
There are other ways to remove elements:
- del to remove items by index or slice
- pop() to remove an item at a specified position (or the final item if no position is given),
- remove() to delete an item by value.
Let’s do this one by one, using the remove() method. Here is the code.
# Removes by value
fruits.remove('banana')
fruits
Here is the output.

Now let’s use our second method, pop(). Here is the code.
# Removes by index and gets the removed item
popped_fruit = fruits.pop(2)
popped_fruit
Here is the output.

Finally, this one will be our final method, del, let’s see. Here is the code.
# Deletes item at index 0
del fruits[0]
fruits
Here is the output.

- remove(value): Removes the initial instance of a given value. It's good when you know the value to eliminate but not its location.
- pop(index): Removes the object and returns it to the designated spot. The final item is removed and returned if no index is supplied. Perfect if you know exactly where to put the object to be removed or if you require the removed item.
- del list[index]: Removes the item from a given slice or index. The object that was deleted is not returned. Ideal for removing a slice or item based on location when the removed item is not needed.
Accessing Elements and Slicing
Using an item's index is a simple way to access elements in a list. Slicing the list will give a subset of it.
Let’s use our first method, index access. Here is the code.
# Accesses the first element
first_fruit = fruits[0]
first_fruit
Here is the output.

Now, let’s use our second method. This time, we’ll slice it between different elements. Here is the code.
# Gets a slice from index 1 to 2
fruit_slice = fruits[1:3]
Here is the output.

- Index Access: Ideal for use when a specific item from the list is required. It's similar to choosing a book off a shelf and knowing exactly where it is.
- Slicing: Excellent for a variety of needs. It's like choosing between two spots on a bookshelf for a series of books.
Searching and Sorting Lists
Next, let’s see methods for searching and sorting lists.
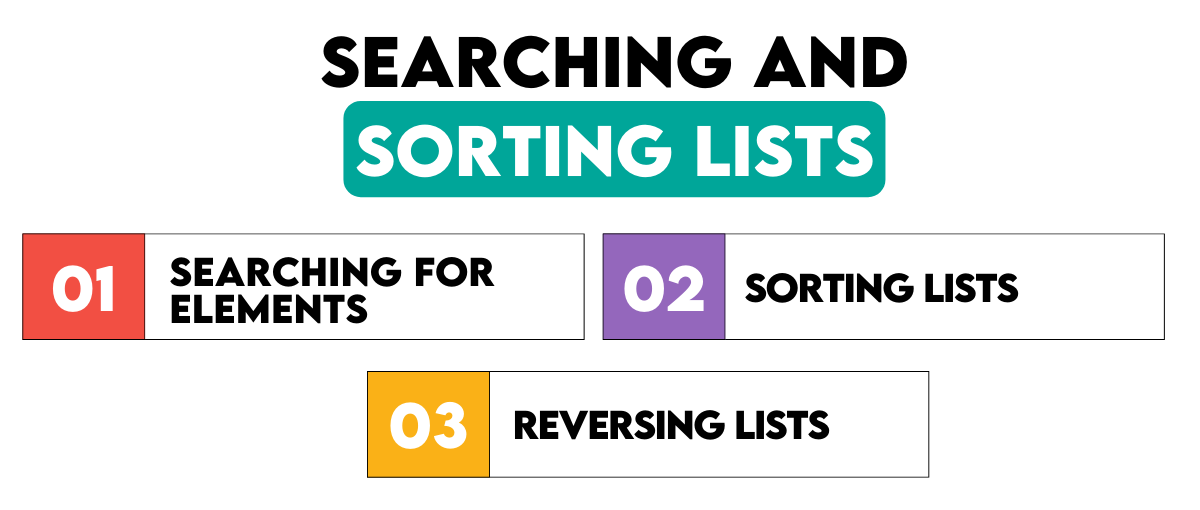
Searching for elements
The in keyword can be used to locate an element in a list and determine its existence. Use the index() method for more in-depth searches, such as determining an item's index. Here is the code.
First, we’ll create a list.
fruits = ['apple', 'banana', 'cherry']
Next, let’s use the straightforward method. Here is the code.
# Returns True if 'banana' is in the list
is_banana_present = 'banana' in fruits
is_banana_present
Here is the output.

Since our list contains this element, let’s see where it is. Here is the code.
# Gets the index of 'banana'
banana_index = fruits.index('banana')
Here is the output.

Sorting lists
The sort() method for in-place sorting, which modifies the underlying list, makes sorting a list simple. Use sorted() to get a sorted version without changing the original.
First, let’s create a list that contains numbers.
numbers = [3, 1, 4, 1, 5, 9, 2]
Next, let’s use the sort method and see what happens after. Here is the code.
# Sorts the list in-place
numbers.sort()
# Returns a new sorted list. The original remains unchanged
sorted_numbers = sorted(numbers)
sorted_numbers
Here is the output.

Reversing lists
Use the reverse() method to revert a list in place or slice with a step of -1 to create a reversed list duplicate.
To do this, we are going to use two different methods. Let’s see how we can do this reverse() method.
# Reverses the list in place
numbers.reverse()
numbers
Here is the output.

Now, let’s use our second method. Here is the code.
# Gets a reversed copy
reversed_numbers = numbers[::-1]
Here is the output.

- Use reverse() when you need to reverse the elements of a list and do not need the original ordering anymore.
- Use slicing with -1 step when you need a reversed version of the list while retaining the original list's order for further use.
Advanced List Manipulation Techniques
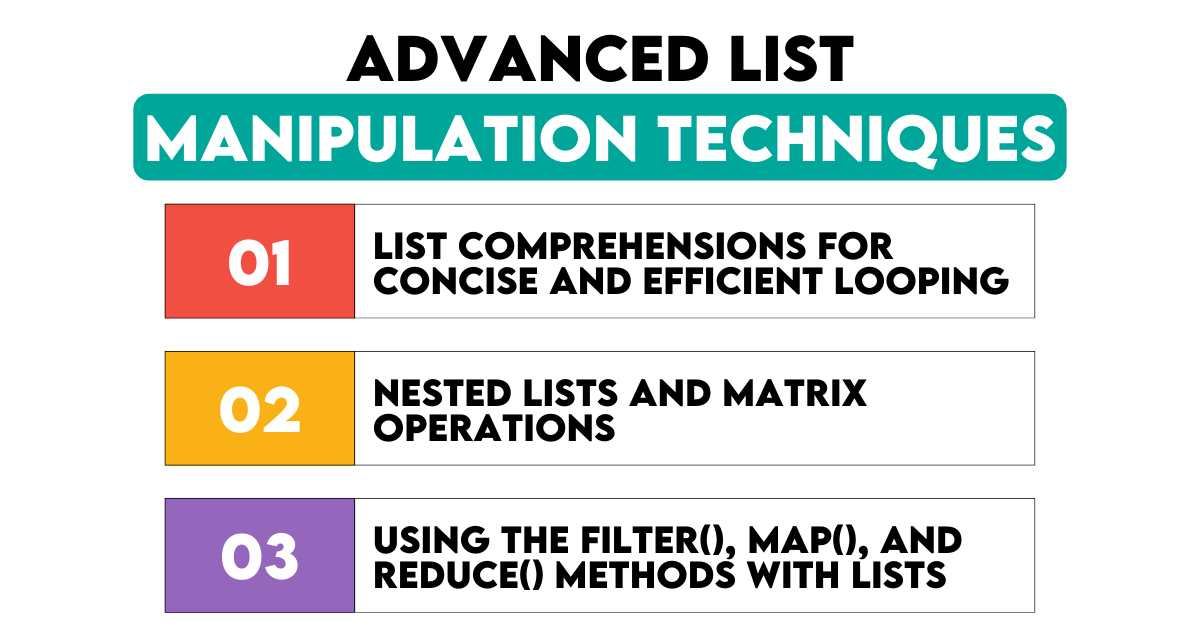
Since we know basic list methods and techniques, let’s look at the more advanced list manipulation techniques. Let’s start with list comprehension and see how it can be used with lists.
List comprehensions for concise and efficient looping
A simple method for creating lists based on existing lists is to use list comprehensions. It's similar to writing a loop with only one line. This is helpful when applying methods to data science pieces or filtering data.
Let’s create a list of squares. Here is the code.
# Creates a list of squares
squares = [x**2 for x in range(10)]
Here is the output.

Now, this time let’s do this by adding conditions. Here is the code.
# Squares of even numbers only
even_squares = [x**2 for x in range(10) if x % 2 == 0]
Here is the output.

Nested lists and matrix operations
Lists inside lists are called nested lists. In data science, they are frequently used for showing matrices or 2D data.
Let’s first create a 3x3 matrix. Here is the code.
# A 3x3 matrix
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
matrix
Here is the output.

Now let’s access the first row. Here is the code.
# Accessing the first row
first_row = matrix[0]
first_row
Here is the output.

Using the filter(), map(), and reduce() methods with lists
These methods provide methods for interacting with lists in functional programming:
- filter() selects items based on a method.
- map() applies a method to each item.
- reduce() aggregates all items into a single output.
Now let’s apply them one by one. Here we can create a list and filter afterward. Here is the code
# Filter: Get only even numbers
even_numbers = list(filter(lambda x: x % 2 == 0, range(10)))
Here is the output.

Now, let’s use the list method with a map. Here is the code.
# Map: Square each number
squares = list(map(lambda x: x**2, range(10)))
squares
Here is the output.

Now let’s use reduce to sum all numbers between 1 and 10.
from functools import reduce
# Reduce: Sum all numbers
sum_of_numbers = reduce(lambda x, y: x + y, range(10))
sum_of_numbers
Here is the output.

Working with List Copies
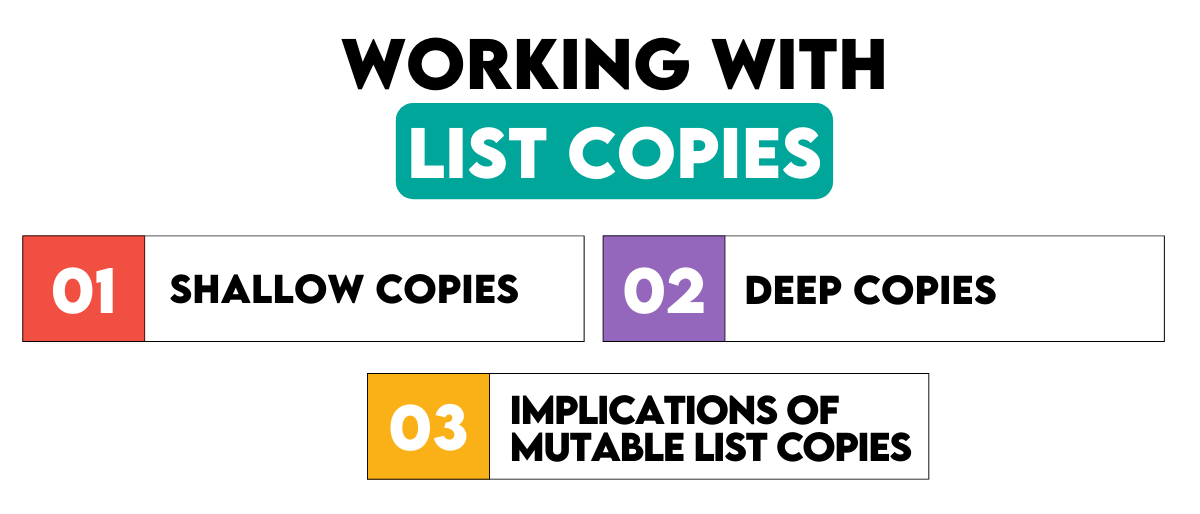
There are two types of list copies: shallow copies and deep copies. Although a shallow copy makes a new list, it doesn't make copies of its items.
This implies that if you have nested lists, the original and the duplicate share the nested lists. A deep copy, on the other hand, is entirely independent of the original as it generates a new list and duplicates of each item in it.
Shallow copies
Now let’s do shallow copies.
import copy
original_list = [[1, 2, 3], [4, 5, 6]]
shallow_copied_list = copy.copy(original_list)
shallow_copied_list[0][0] = 'changed'
Here is the output.

Let’s see original_list again.
original_list
Here is the output.

Deep copies
Next, let’s do deep copies. Here is the code.
import copy
original_list = [[1, 2, 3], [4, 5, 6]]
deep_copied_list = copy.deepcopy(original_list)
deep_copied_list[0][0] = 'deep change'
Here is the output.

Now let’s see original_list again to compare both methods. Here is the code.
original_list
Here is the output.

- Shallow Copy: Changes to nested objects within the shallow copy affect the original list because both reference the same nested objects.
- Deep Copy: Changes to any part of the deep copy (including nested objects) do not affect the original list. Each copy is completely independent.
Implications of mutable list copies
The most important thing to remember when working with changeable objects, such as lists, is that if you make changes to one, they may show in other locations if they share a reference.
Knowing the difference between shallow and deep copies is essential when dealing with complicated data structures in data research to prevent unexpected consequences.
Tips for optimizing list manipulation
- Use list comprehensions instead of loops when building lists; they are frequently quicker and easier to understand.
- Reduce Appending in Loops: Generating lists using comprehensions or map() is more efficient than appending in a loop wherever feasible.
- Sort List Sizes in Advance: Preallocate the list in advance if you know how big it will be to save money on resizing during add operations.
Common Pitfalls and How to Avoid Them
- Modifying Lists While Iterating: Make a copy of the list for iteration purposes if you plan to modify it to avoid unexpected behavior.
- Confusing Shallow and Deep Copies: Understand the difference to prevent unintended modifications.
- Overusing List Comprehensions: While powerful, they can become unreadable if too complex. Keep them simple.
To learn more about Python lists, you can read this one too.
Final Thoughts
After exploring Python list methods, it's evident that these resources are essential for effective programming and data analysis, serving as more than just a means of storing and modifying data.
Anyone wanting to succeed in data science must learn these approaches, which range from basic addition and subtraction to more complex strategies like matrix operations and list comprehension. Keep in mind that practice is the only way to become fully competent.
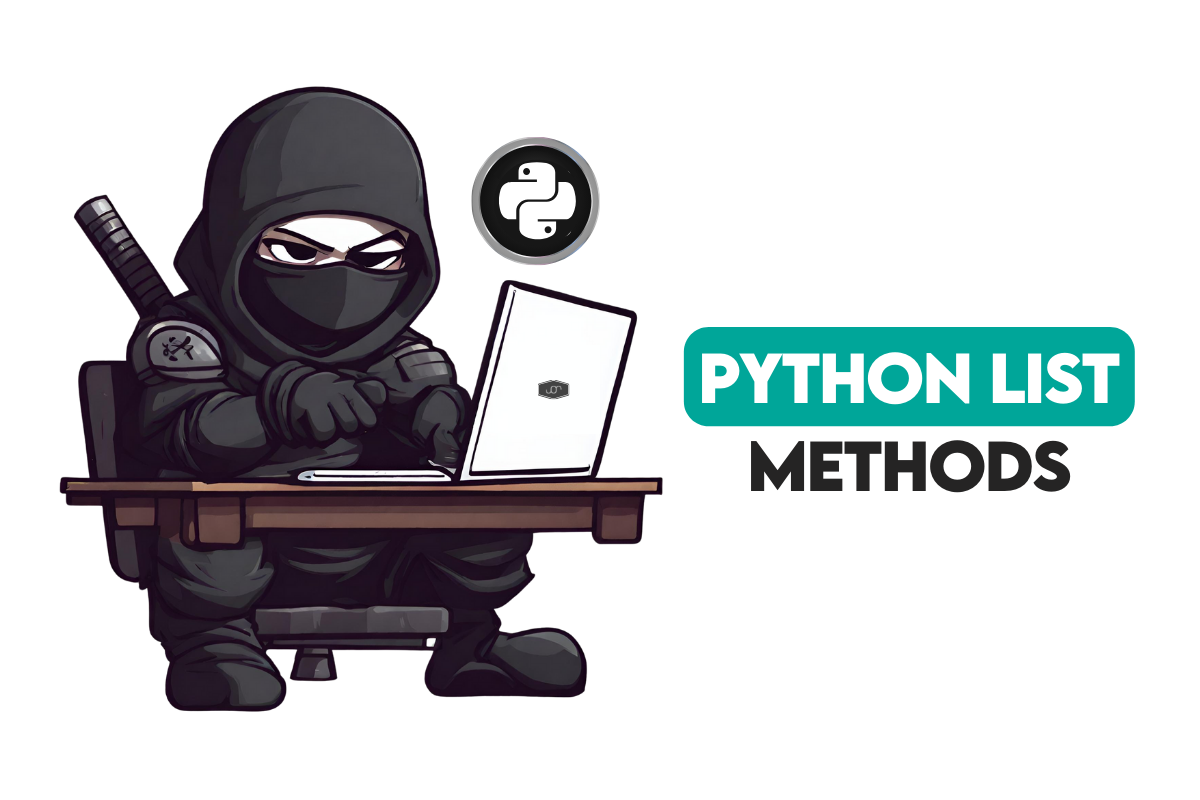