A Comprehensive Guide to Python Lists and Their Power
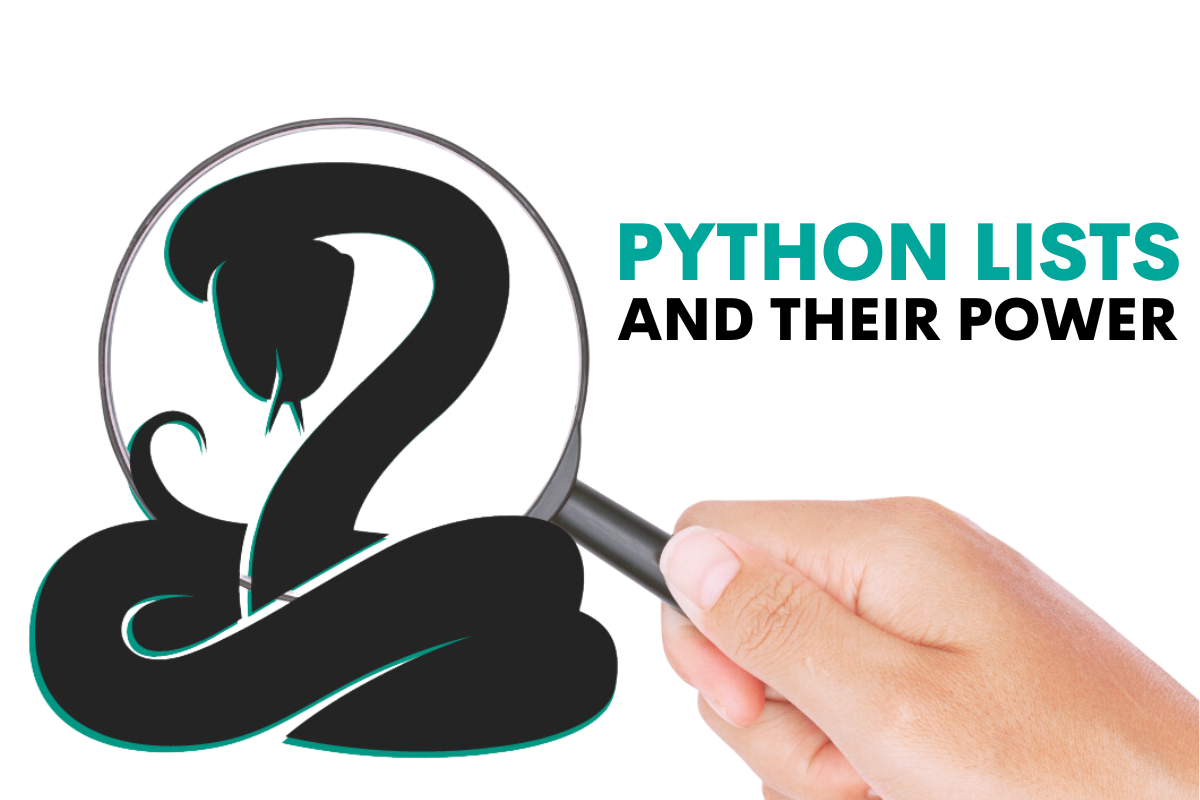
- Written by:
Nathan Rosidi
Python lists from A to Z. Learn about creating, modifying, and performing complex operations, along with valuable list methods to elevate your coding skills.
Are you still struggling with managing data in Python? Have you ever stayed awake at night thinking about how to store and manipulate a collection of data points?
Jokes aside, this article will help you go through the ins and outs of Python lists – one of Python's most useful data structures and a crucial tool in every data scientist's toolbox.
As you develop Pythonic skills, Advanced Python Concepts will become increasingly important to you.
One of these concepts is Python Data Types, which we covered in our latest article in the series.
Moreover, Python operators are essential for manipulating data, a core part of data science.
Now let’s get back to the Python lists; as being said, it also is a vital tool in every data scientist’s toolbox.
What Is a Python List?
A Python list is a built-in data structure that stores a collection of items.
You can store different types of items in a list, including integers, floats, strings, and even other lists!
This makes Python lists incredibly useful. A list in Python is ordered and changeable, meaning you can access items by referring to their index number. You can change, add, and remove items in a list after it has been created.
How to Create Python Lists?
Python lists are written in and can be created with square brackets. The items are separated by commas, like in the following example.
pyton_list = ["element_1", "element_2"]
print(python_list)
It's no secret that mastering Python lists can significantly simplify your data-handling tasks and enhance your data science workflow.
Ready to learn more about Python lists?
Let's go!
Common Python List Methods You Should Know
Best of all, Python lists come with built-in methods that make them not just easy but intuitive to use.
These methods allow us to interact and manipulate lists in many different ways, proving their importance when managing and wrangling data.
From creating Python lists, accessing individual elements, and modifying elements, to adding or removing elements from the list - Python equips you with a suite of tools to perform these tasks with simplicity and efficiency.
That's why, in the following sections, we're going to delve deep into these methods, explaining each in detail to give you a coding example of its functionality.
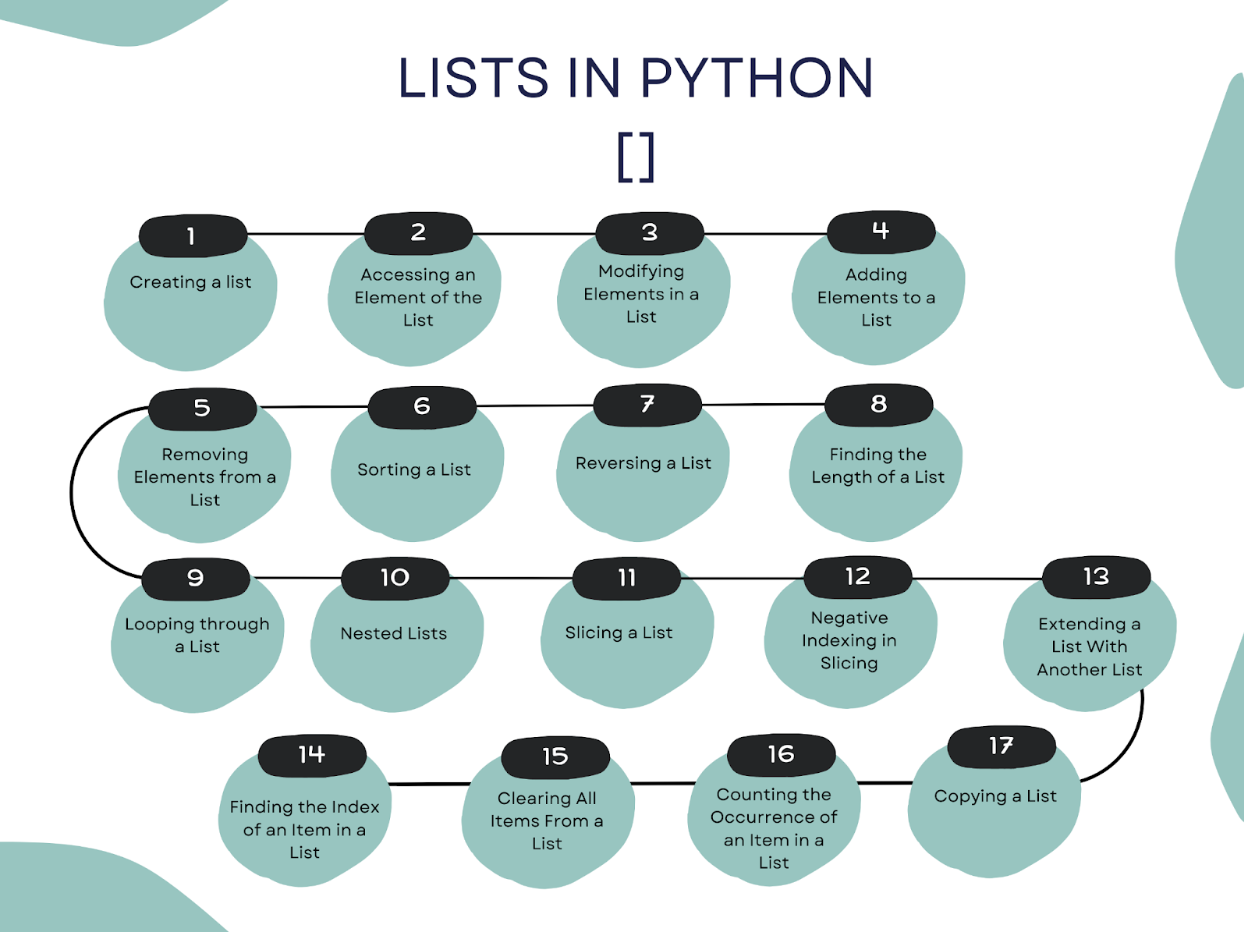
1. Creating a List
Creating a list involves placing elements within square brackets and separating them with commas.
The following code creates a list named "fruits" and assigns three elements to it: "apple", "banana", and "cherry".
fruits = ["apple", "banana", "cherry"]
print(fruits)
Here is the output.

2. Accessing an Element of the List
Accessing an element of the list is done by referencing its index within square brackets.
The following code accesses the second element of the list "fruits" which is "banana".
fruits = ["apple", "banana", "cherry"]
print(fruits[1])
Here is the output.

3. Modifying Elements in a List
Modifying elements in a list can be done by assigning a new value to a specific index.
The following code changes the second element of the list "fruits" from "banana" to "grapes".
fruits = ["apple", "banana", "cherry"]
fruits[1] = "grapes"
print(fruits)
Here is the output.

4. Adding Elements to a List
Adding elements to a list can be done using the append(), insert(), or extend() methods.
The following code adds a new element, "grapes", to the end of the list of fruits.
fruits = ["apple", "banana", "cherry"]
fruits.append("grapes")
print(fruits)
Here is the output.

5. Removing Elements From a List
Removing elements from a list can be done using the remove() or pop() methods.
The following code removes the element "banana" from the list of fruits.
fruits = ["apple", "banana", "cherry"]
fruits.remove("banana")
print(fruits)
Here is the output

6. Sorting a List
For sorting a list, use the sort() or the sorted() function.
The following code sorts the elements of the list of fruits in ascending order.
fruits = ["apple", "banana", "cherry"]
fruits.sort()
print(fruits)
Here is the output.

7. Reversing a List
To reverse a list, you can use the reverse() method or the slicing operator ":" (colon) with a step of -1.
The following code reverses the order of the elements in the list "fruits".
fruits = ["apple", "banana", "cherry"]
fruits.reverse()
print(fruits)
Here is the output.

8. Finding the Length of a List
Finding the length of a list can be done using the len() function.
The following code returns the number of elements in the list "fruits", which is 3.
fruits = ["apple", "banana", "cherry"]
print(len(fruits))
Here is the output.

9. Looping Through a List
Quite intuitively, looping through a list can be done using the for loop.
The following code loops through each element in the list "fruits" and prints each one.
fruits = ["apple", "banana", "cherry"]
for fruit in fruits:
print(fruit)
Here is the output.
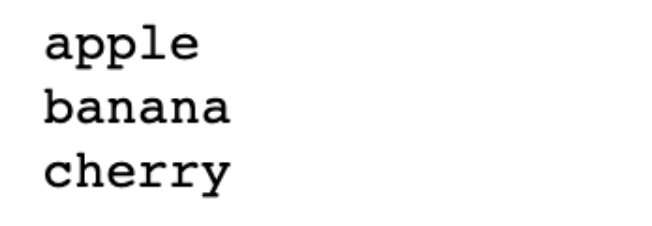
10. Nested Lists
A list can contain another list as an element, forming a nested list.
The following code creates two separate lists: "fruits" and "numbers". It then nests them into a third list "lists".
fruits = ["apple", "banana", "cherry"]
numbers = [1, 2, 3]
lists = [fruits, numbers]
print(lists)
Here is the output.

11. Slicing a List
Slicing a list involves selecting a sub-section of the list by specifying the start and end indices.
The following code creates a new list from the original list of "numbers" by slicing it from the 3rd element.
This element’s index is 2 (inclusive) because Python is zero indexing programming language. That means the first element’s index is 0.
The slicing operation will end at index 7, which is the number 8 (exclusive).
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
print(numbers[2:7]) # [3, 4, 5, 6, 7]
Here is the output.

12. Negative Indexing in Slicing
Negative indexing can be used in slicing to access elements from the end of the list.
The following code creates a new list from the original list "numbers" by slicing it from the third element from the end.
This element's index is -3 because, in Python, negative indexing starts from the end of the list. It means the last element's index is -1 (number 10), the second last is -2 (number 9), the next one is -3 (number 8), and so on.
So slicing starts from index -3 (inclusive) and goes to index -1 (exclusive).
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
print(numbers[-3:-1])
Here is the output.

13. Extending a List With Another List
The extend() method can be used to add elements from one list to another.
The following code extends a list with another list.
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
more_numbers = [11, 12, 13, 14, 15]
numbers.extend(more_numbers)
print(numbers)
Here is the output.

14. Finding the Index of an Item in a List
The index() method is used to find the index position of a particular element in a list. It returns the first occurrence of the specified value. If the element is not found, it raises a ValueError.
In this code, we create a list of numbers and then use the index() method to find the index of the number 5 in the list.
Python lists are zero-indexed, meaning the first element is at index 0, the second element is at index 1, and so forth. So when we ask for the index of 5, it returns 4 because 5 is the fifth element in the list.
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
print(numbers.index(5))
Here is the output.

15. Clearing All Items From a List
The clear() method removes all items from a list. It doesn't take any parameters and doesn't return any value.
The following code demonstrates removing all elements from a list using the clear() method.
We start with a populated list of numbers, then call numbers.clear(), which removes all elements from the list "numbers". When we print the list, it returns an empty list.
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
numbers.clear()
print(numbers)
Here is the output.

16. Counting the Occurrence of an Item in a List
The count() method returns the number of times a specified value appears in the list.
In the following code, we created a list with some repeating numbers. The count() method is used to count the number of times a particular element (in this case, the number 5) appears in the list. It returns 4, indicating that the number 5 appears four times in the list.
Here is the code.
numbers = [1, 2, 2, 3, 4, 4, 4, 5, 5, 5, 5]
print(numbers.count(5))
Here is the output.

17. Copying a List
The copy() method returns a copy of the list. It's a way to duplicate a list without affecting the original.
The following code shows how to create a copy of a list.
The copy() method doesn't affect the original list. It creates a new list that is a copy of the original. This can be helpful when you want to manipulate a list but also need to preserve the original data. After making a copy of the list, we print it to confirm that it matches the original.
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
numbers_copy = numbers.copy()
print(numbers_copy)
Here is the output.

Conclusion
In conclusion, Python lists, with their flexibility and the extensive range of built-in methods, form a vital part of any data scientist or programmer's toolkit.
From manipulating data to effectively managing complex data structures, the power and simplicity of Python lists are undeniable.
Mastering these methods enables you to write cleaner, more efficient code and gives you a solid foundation for exploring more complex data structures.
So remember, practice these methods, incorporate them into your regular coding routine, and watch how they transform your Python programming experience.
Sounds intriguing? Don't forget to visit our platform for more insightful Python tips and tricks to boost your data science skills.
There's always more to learn, and we're here to guide you through it.
Share