Python Collections: Essentials to Advanced
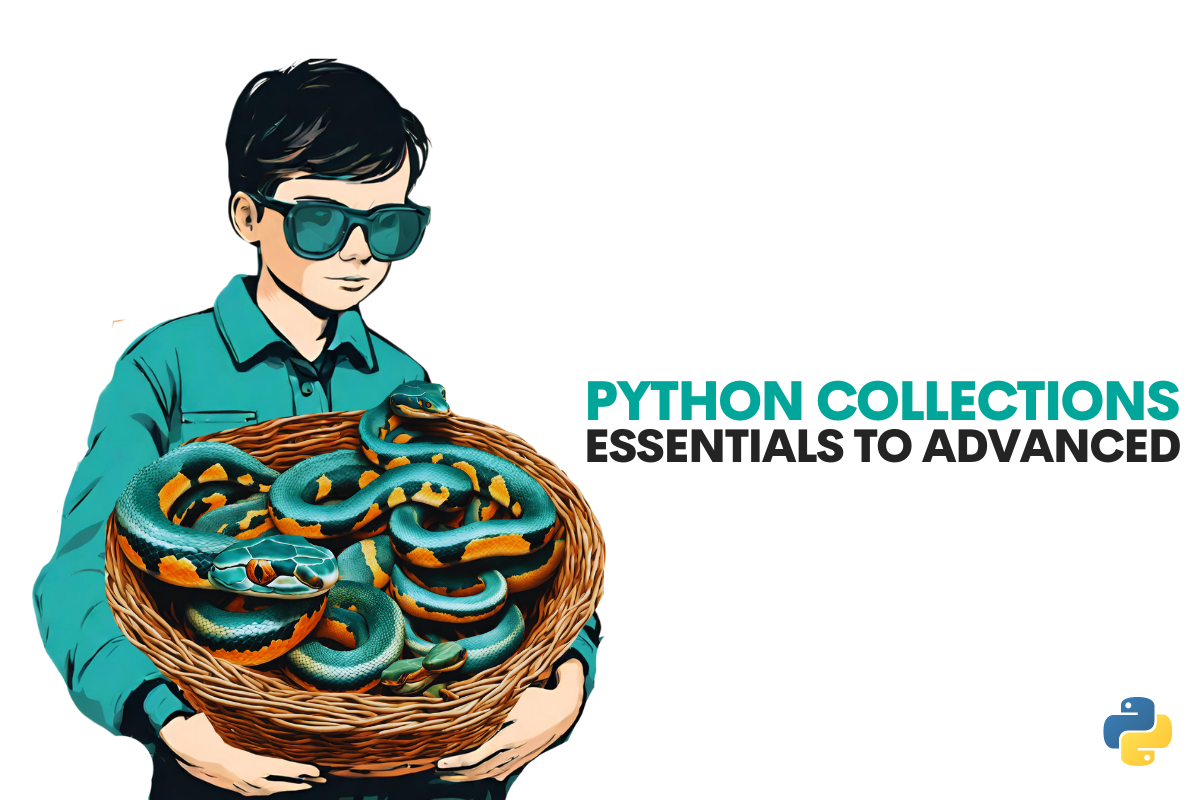
Categories
Mastering Python Collections: In-depth exploration of Lists, Dictionaries, Tuples, and advanced data structures for optimal coding efficiency
In programming, picking the right way to store data is very important. It makes solving hard problems easier. Python is great for this, simple and well-designed. Python Collections are a big part of this.
Python collections make handling data easy and smart, which means your code works well and quickly.
In this article, we will talk about the Python Collections module. We will look into its parts and learn why they are important. We will also see how to use them in real situations. From lists and tuples to ChainMaps and special data structures, we want to give you a full understanding.
Beginner Collections in Python
Let's focus on beginner Python collections: lists, dictionaries, and tuples.
Python Lists allow you to store items in a sequence. You can add or take away items, making them flexible and easy for beginners. Here is it’s syntax.
list = ["here", "is", "a", "simple","list"]
list

Python Dictionaries, on the other hand, are about matching keys to values. It is as a way to store information where you can quickly find something by its name. This makes them perfect for organizing and retrieving data, especially when you know exactly what you're looking for.
dict = {"here": "is", "a": "simple","list" : "."}
print(dict.keys())
print(dict.values())

Tuples, on the other hand, are used to store items, but you can't change them once they're made. This is useful for data that should stay the same, providing a simple and reliable way to handle constant information.
my_tuple = ("here", "is", "a", "simple", "tuple")
my_tuple

Together, these collections form the foundation of data handling in Python, offering different ways for beginners to manage and use data effectively. Now let’s look at advanced collections.
Advanced Collections in Python
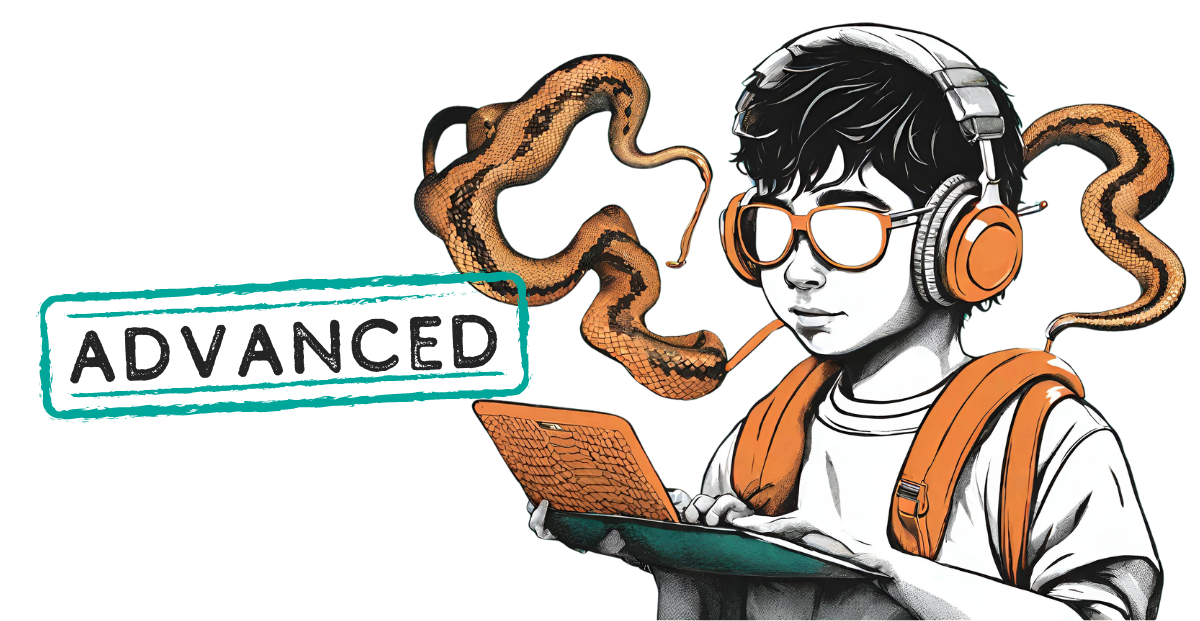
The Counter, for example. It's a special kind of dictionary for counting things that can be counted, like words in a text or items in a list. It shows how Python can easily solve common problems.
Then, there's the OrderedDict. Before Python 3.7, dictionaries did not save the order of items. OrderedDict solved this, so developers can rely on the order of items for their work.
Another important one is the defaultdict. It gives a default value to keys that are not there, which is very useful for complex data and stops errors with keys.
These advanced collections show Python's commitment to providing strong and flexible ways to store data, making programming a lot easier.
Practical Applications of Advanced Python Collections
The following practical applications showcase how Python Collections can be used to solve real-world problems in a Pythonic, efficient manner, making them an indispensable part of any Python developer's toolkit.
Python Collections come to life when applied to real-world problems. Let's look at some practical examples with code to illustrate their usage.
1. Managing User Sessions with defaultdict:
Take, for instance, a web application that needs to manage user sessions. A defaultdict can be used to handle session data, automatically creating a new session for users who don't have one yet. This simplifies the logic required to check and create sessions, making the code cleaner and more efficient.
from collections import defaultdict
# Initialize a defaultdict with an empty dictionary as the default value
user_sessions = defaultdict(dict)
# Simulate user sessions
user_sessions['Alice']['last_login'] = '2023-01-01'
user_sessions['Bob']['last_login'] = '2023-01-02'
# Accessing a new user's session creates it automatically
print(user_sessions['Eve'])
Here is the output.

This code above automatically handles the creation of a session for new users, avoiding the need for manual checks or session initialization.
2. Data Analysis with Counter
In data analysis, a Counter is invaluable.
Analyzing datasets often involves counting occurrences of various elements. With a Counter, this task becomes a matter of a few lines of code, allowing you to focus more on the analysis part rather than the counting mechanics.
Let’s see how the Counter class makes this process straightforward.
from collections import Counter
# Sample data: words in a sentence
words = ['apple', 'banana', 'apple', 'orange', 'banana', 'apple']
# Count the occurrences of each word
word_count = Counter(words)
print(word_count)
Here is the output.

This simple example demonstrates how Counter can be used to tally up items, a frequent requirement in data analysis.
3. Configuration Management with ChainMap:
And when dealing with configurations or settings, a ChainMap can be a game-changer. It allows you to create a single view of multiple dictionaries, like default settings and user-specific settings. This way, you can easily manage and override configurations without the need for complex merging logic. Let’s see how ChainMap is useful to deal with multiple layers of settings.
from collections import ChainMap
# Default settings
default_settings = {'theme': 'light', 'notifications': True}
# User-specific settings
user_settings = {'theme': 'dark'}
# Combine settings with ChainMap
combined_settings = ChainMap(user_settings, default_settings)
print(combined_settings['theme'])
print(combined_settings['notifications'])
Here is the output.

This code elegantly handles the combination of two dictionaries, prioritizing the user settings over the defaults.
4. Efficient Queue Operations with Deque
Deque stands for "double-ended queue" and is a collection that supports adding and removing items from either end efficiently. This makes it perfect for tasks that require a queue structure, such as processing tasks in order or managing states in games.
from collections import deque
# Initialize a deque
tasks = deque(['task1', 'task2', 'task3'])
# Enqueue tasks to the front and back
tasks.append('task4') # add to the end
tasks.appendleft('task0') # add to the front
print(tasks)
Here is the output.

5. Structured Data with NamedTuple
Namedtuple provides a way to create tuple-like objects that have fields accessible by attribute lookup as well as being indexable and iterable. This brings clear advantages in terms of code readability and structure.
from collections import namedtuple
# Create a namedtuple to represent a point in 2D space
Point = namedtuple('Point', ['x', 'y'])
# Instantiate a Point object
p = Point(10, y=20)
# Accessing the elements
print(p.x, p.y)
Here is the output.

6. Maintaining Order with OrderedDict
OrderedDict is a special type of dictionary that remembers the order in which its contents are added. Even though regular dictionaries now maintain insertion order as of Python 3.7,
OrderedDict can still be useful when you need to ensure the order is maintained, such as when you're exporting data to a CSV file where the column order matters.
from collections import OrderedDict
# Create an OrderedDict to remember the order items are added
favorite_fruits = OrderedDict()
# Add items to the OrderedDict
favorite_fruits['apple'] = 'green'
favorite_fruits['banana'] = 'yellow'
favorite_fruits['cherry'] = 'red'
print(favorite_fruits)
Here is the output.

Best Practices and Tips for Using Python Collections
In this article, we explored various Python collections like lists, dictionaries, tuples, and more advanced structures such as ChainMaps, Counters, and defaultdicts. Now, let's see on some best practices and tips we learned along the way.
Choosing the Right Collection: We saw how different collections serve different purposes. Picking the right one, like using lists for ordered items or dictionaries for key-value pairs, is crucial for efficient programming.
Efficiency Matters: Throughout our examples, efficiency was key. Remember to use collections in a way that keeps your code running fast. For example, using sets for fast membership testing.
Mutable vs Immutable: We discussed mutable collections like lists and immutable ones like tuples. Choosing between them depends on whether your data should change or stay the same.
Nested Collections: We also saw collections within collections, like dictionaries inside lists.This can be complex, but it's powerful for organizing data.
Error Handling: Common mistakes with collections, such as key errors in dictionaries, were highlighted. Learning to handle these errors gracefully is important.
Functional Programming with Collections: We didn't cover this in depth, but using collections with functional programming concepts like map and filter can make your code more elegant.
Comprehensions for Simplicity: Finally, using comprehensions to create collections can make your code more readable and concise.
Conclusion
We have learned about Python Collections. They come in many types and uses, and they make Python programming look good. We saw simple ones like lists, tuples, and dictionaries, and more complex ones like ChainMaps, Counters, defaultdicts, and more.
Keep practicing if you're a data scientist, developer, or Python fan.Here, you can see top 30 python interview questions and answers. The more you use the concepts you have learned, the easier they get.
On StrataScratch platform, there is a bunch of interview questions from big companies, including Fortune 500 and FAANG, which will make your path easier to pass through.
FAQ’s
What are Python collections?
Python collections are ways to store and organize data. They help you keep your data in order and make it easy to work with. Examples include lists, dictionaries, and tuples.
How many collections are there in Python?
Python has several collections. The main ones are lists, dictionaries, tuples, sets, and a few special ones from the collections module like Counter, defaultdict, and OrderedDict.
What is the Python equivalent of Java collections?
In Python, collections like lists, dictionaries, and sets are similar to Java's ArrayList, HashMap, and HashSet. Python's collections module also has more advanced types, similar to some in Java's collections framework.
What are the collections elements in Python?
Collection elements in Python are the items or data stored in a collection. For example, in a list, each item is an element. In a dictionary, each key-value pair is an element.
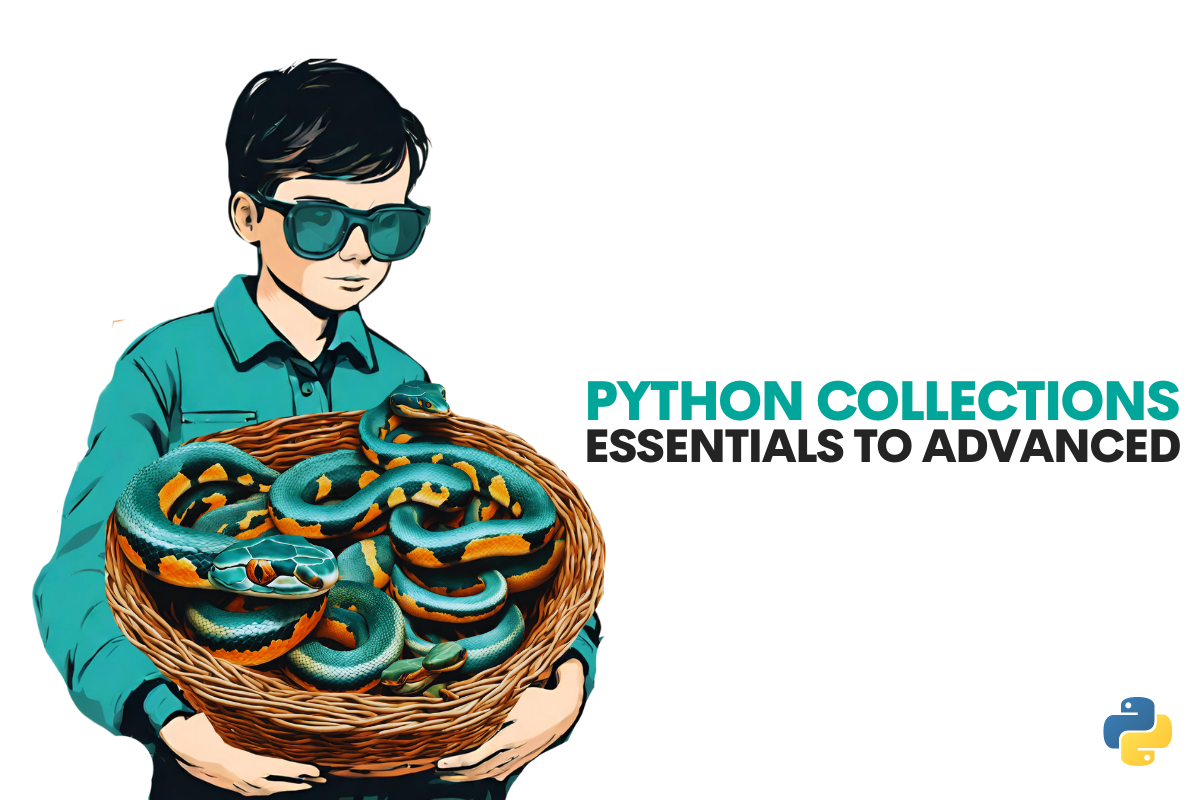