AI Interview Questions: A Guide to Crafting Winning Answers
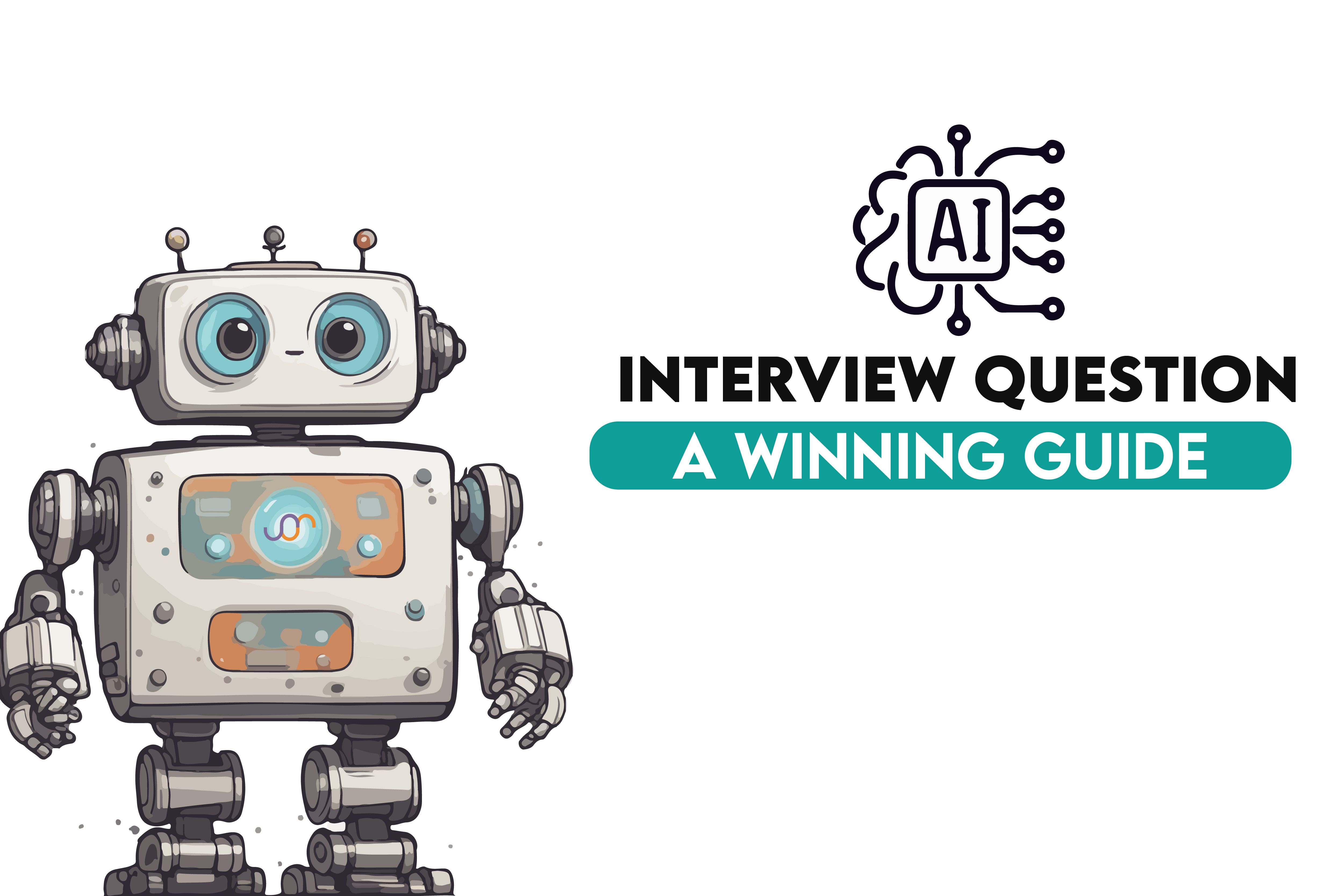
- Written by:
Nathan Rosidi
Master the AI Job Interview: Coding, Theoretical, and Scenario-Based Questions Uncovered
Today, more than ever, AI affects not just our daily lives but also our professional lives. According to Mc Kinsey Report, by 2030 at least 12 million people will need to change their careers, because it will be replaced by AI.
What does this mean? It means that AI is not only a good career choice for now but might become mandatory in the future. It's safe to say that AI still requires human supervision, as evidenced by platforms like ChatGPT. To land a job in this industry, preparation is key.
In this article, you'll learn about AI interview questions divided into three categories: coding, theoretical, and scenario-based. We'll also cover frequently asked questions at the end. Let's get started!
AI Interview Questions
AI interviews can be challenging at first, which is why breaking them into subsections can help. Typically, AI interview questions are designed to evaluate your technical skills through coding your theoretical questions and your problem-solving capabilities with scenario-based questions.
In this section, we will go through each type, starting with coding questions. We'll focus on Python, as it's the go-to programming language for AI coding.
AI Coding Interview Questions
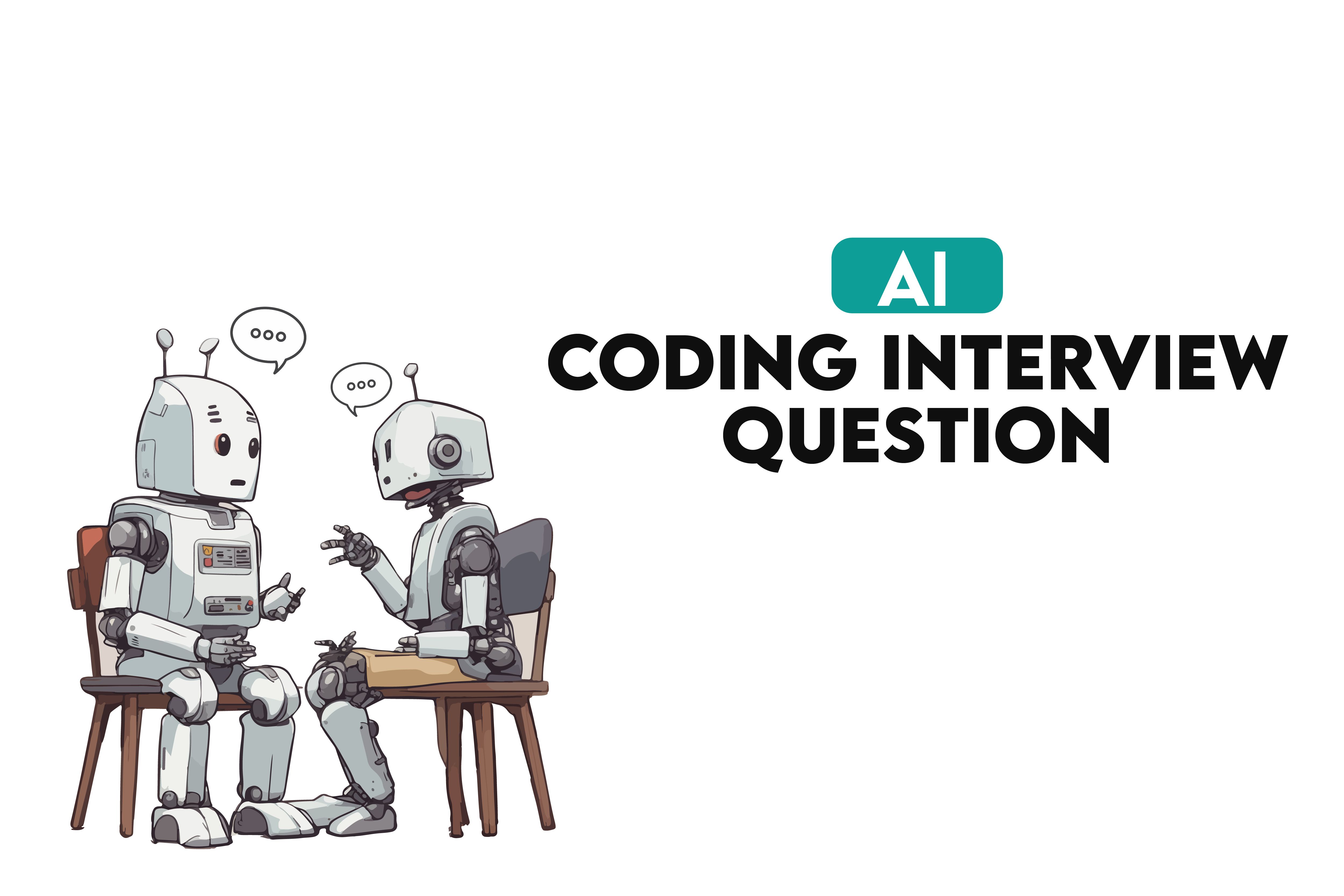
In this section, we'll explore AI interview questions that test your hands-on coding skills. You may be asked to write code snippets or algorithms that perform certain tasks, beginning from implementing a simple regression model to implementing CNN.
You'll be evaluated on your code's efficiency, clarity, and sometimes creativity. These AI interview questions often require you to use programming languages like Python and may use libraries like scikit-learn, TensorFlow, or PyTorch. Let’s start!
Simple Linear Regression
Write a Python function to implement simple linear regression using scikit-learn.
from sklearn.linear_model import LinearRegression
import numpy as np
# Sample data
X = np.array([1, 2, 3, 4, 5]).reshape(-1, 1)
y = np.array([2, 4, 3, 3, 5])
# Initialize and fit the model
model = LinearRegression()
model.fit(X, y)
# Predict
new_data = np.array([6]).reshape(-1, 1)
prediction = model.predict(new_data)
print("Prediction:", prediction)
This code above uses Python's scikit-learn library to make a simple linear regression model. It uses sample data X and Y to train the model. Then, it predicts the y-value for a new X-value of 6.
Decision Tree Classifier
Create a Decision Tree Classifier using scikit-learn.
from sklearn.tree import DecisionTreeClassifier
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
# Load data and split
iris = load_iris()
X_train, X_test, y_train, y_test = train_test_split(iris.data, iris.target)
# Initialize and fit the model
clf = DecisionTreeClassifier()
clf.fit(X_train, y_train)
# Evaluate
accuracy = clf.score(X_test, y_test)
print("Accuracy:", accuracy)
Here, we're using a decision tree to classify types of iris flowers. We split the dataset into training and test sets, then measure how well the model performs with the test set by checking its accuracy.
K-Means Clustering
Write a Python function to perform k-means clustering.
from sklearn.cluster import KMeans
import numpy as np
# Sample data
X = np.array([[1, 2], [5, 8], [1.5, 1.8], [8, 8], [1, 0.6], [9, 11]])
# Initialize and fit the model
kmeans = KMeans(n_clusters=2)
kmeans.fit(X)
# Get cluster centers and labels
centers = kmeans.cluster_centers_
labels = kmeans.labels_
print("Cluster Centers:", centers)
print("Labels:", labels)
The code is for k-means clustering, another machine-learning model. We provide it with some data points, and it organizes them into two clusters. Then, it tells us the center points of these clusters.
Sentiment Analysis
Implement a sentiment analysis model.
import nltk
from nltk.sentiment.vader import SentimentIntensityAnalyzer
# Initialize
sia = SentimentIntensityAnalyzer()
# Sample sentence
sentence = "I love this product!"
# Analyze sentiment
score = sia.polarity_scores(sentence)['compound']
# Classify
if score >= 0.05:
print("Positive")
elif score <= -0.05:
print("Negative")
else:
print("Neutral")
This is a sentiment analysis model using the nltk library. It takes a sample sentence and gives it a sentiment score. Based on this score, it decides if the sentiment is positive, negative, or neutral.
Convolutional Neural Network
Build a Convolutional Neural Network (CNN) using TensorFlow
import tensorflow as tf
from tensorflow.keras import layers, models
from tensorflow.keras.datasets import cifar10
# Load and preprocess data
(train_images, train_labels), (test_images, test_labels) = cifar10.load_data()
train_images, test_images = train_images / 255.0, test_images / 255.0
# Build the CNN model
model = models.Sequential([
layers.Conv2D(32, (3, 3), activation='relu', input_shape=(32, 32, 3)),
layers.MaxPooling2D((2, 2)),
layers.Conv2D(64, (3, 3), activation='relu'),
layers.MaxPooling2D((2, 2)),
layers.Conv2D(64, (3, 3), activation='relu'),
layers.Flatten(),
layers.Dense(64, activation='relu'),
layers.Dense(10)
])
# Compile and fit the model
model.compile(optimizer='adam',
loss=tf.keras.losses.SparseCategoricalCrossentropy(from_logits=True),
metrics=['accuracy'])
model.fit(train_images, train_labels, epochs=10)
# Evaluate
test_loss, test_acc = model.evaluate(test_images, test_labels)
print("Test Accuracy:", test_acc)
The code is for building a Convolutional Neural Network using TensorFlow. It's used for image classification. We train the model with pictures of different objects and then test its accuracy on a test dataset.
AI Theoretical Interview Questions
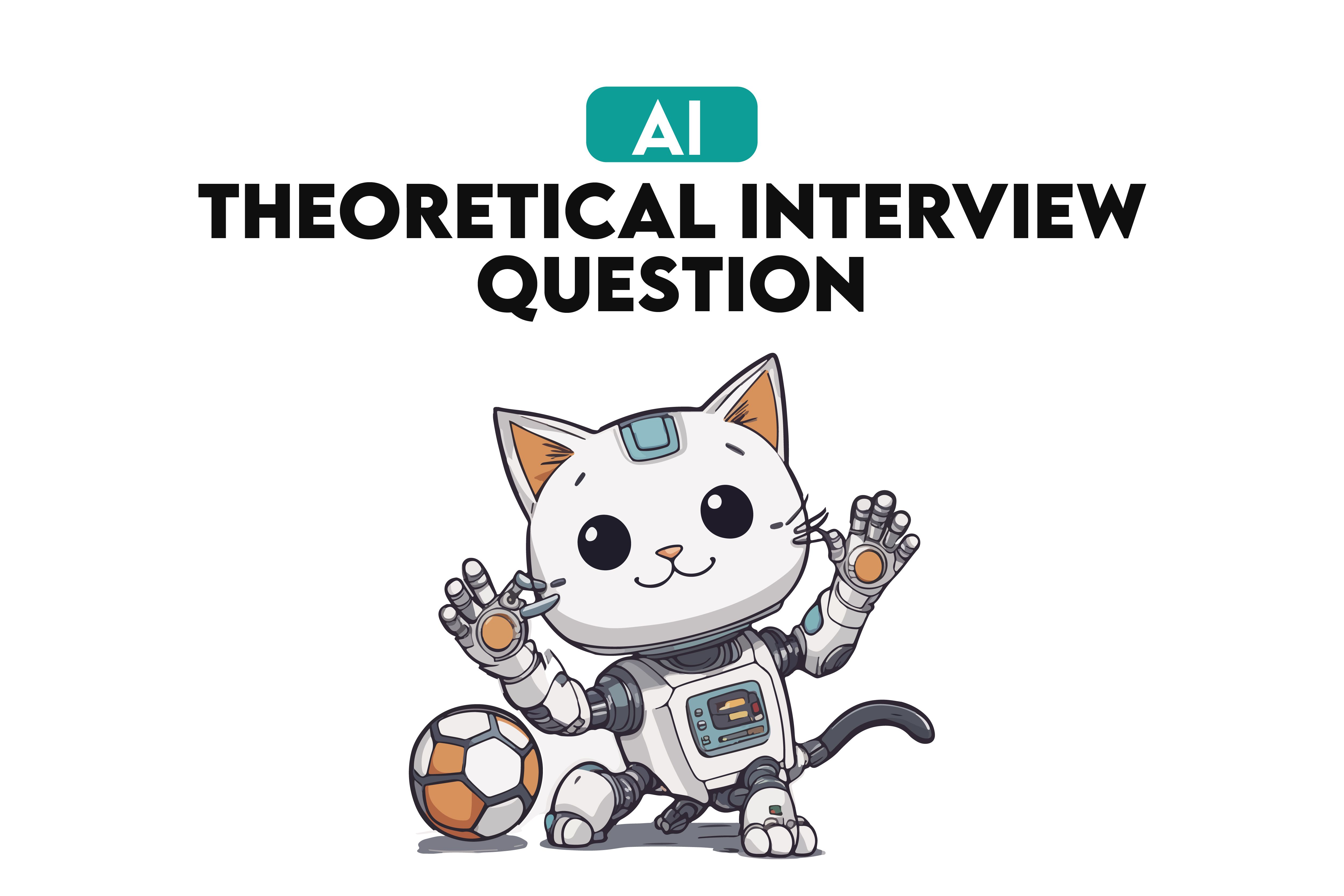
Theoretical questions test your understanding of the fundamental principles behind AI, machine learning, and data science. This could range from explaining the algorithm simply to discussing the importance of regularization.
You might be asked to explain how a particular machine-learning model works, and the differences between similar models.
SVM Classifier
This AI interview question was asked in the Data Science interview by Uber.
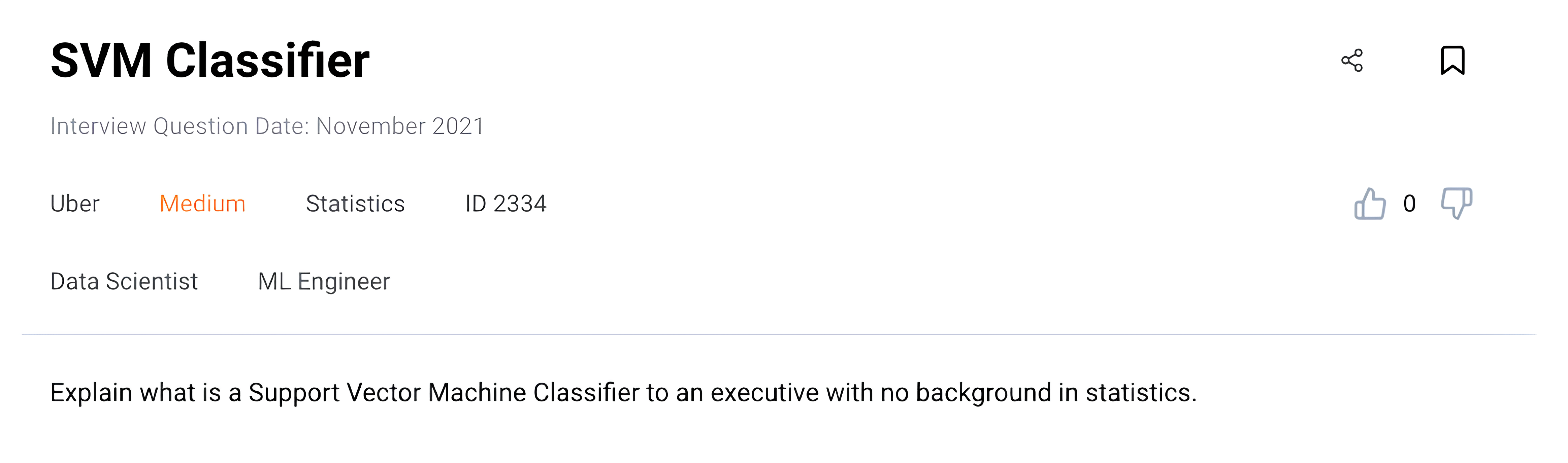
Link to this question: https://platform.stratascratch.com/technical/2334-svm-classifier
Imagine you have red and blue balls on a table. A Support Vector Machine (SVM) helps you draw a straight line that separates these balls as cleanly as possible. It's a tool for making decisions based on data.
Selecting K
This AI interview question was asked in the Machine Learning Engineer Interview at Oracle.
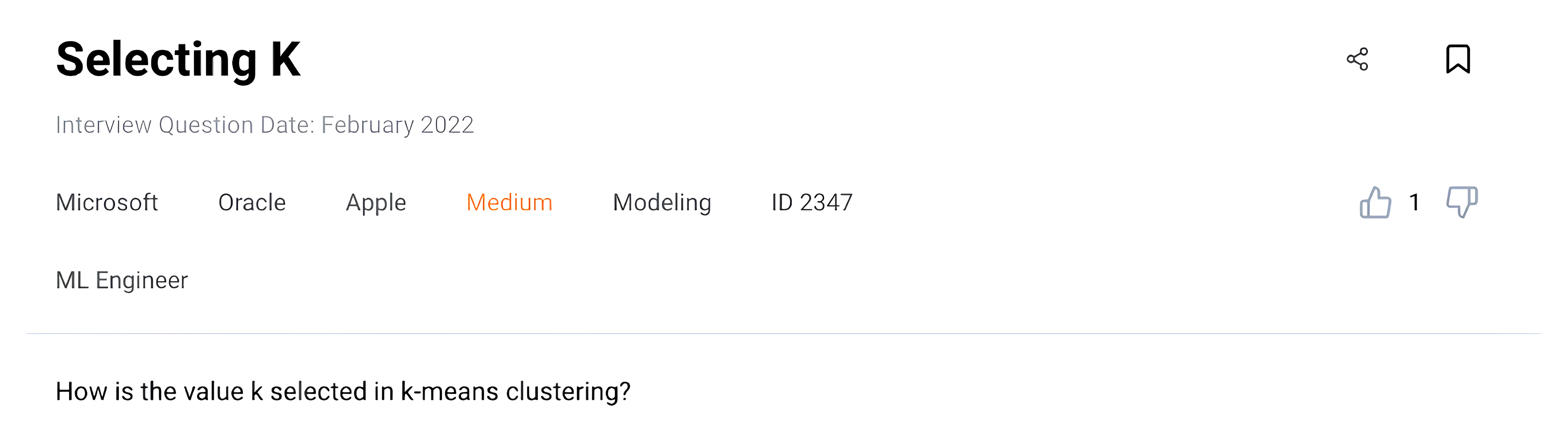
Link to this question: https://platform.stratascratch.com/technical/2347-selecting-k
The value 'k' is the number of groups you want to divide your data into. Methods like the "elbow method" help you find the best 'k' by plotting different values and looking for a point where adding more clusters doesn't improve much.
Regularization
This question was asked in Machine Learning Engineer interviews by Galvanize.
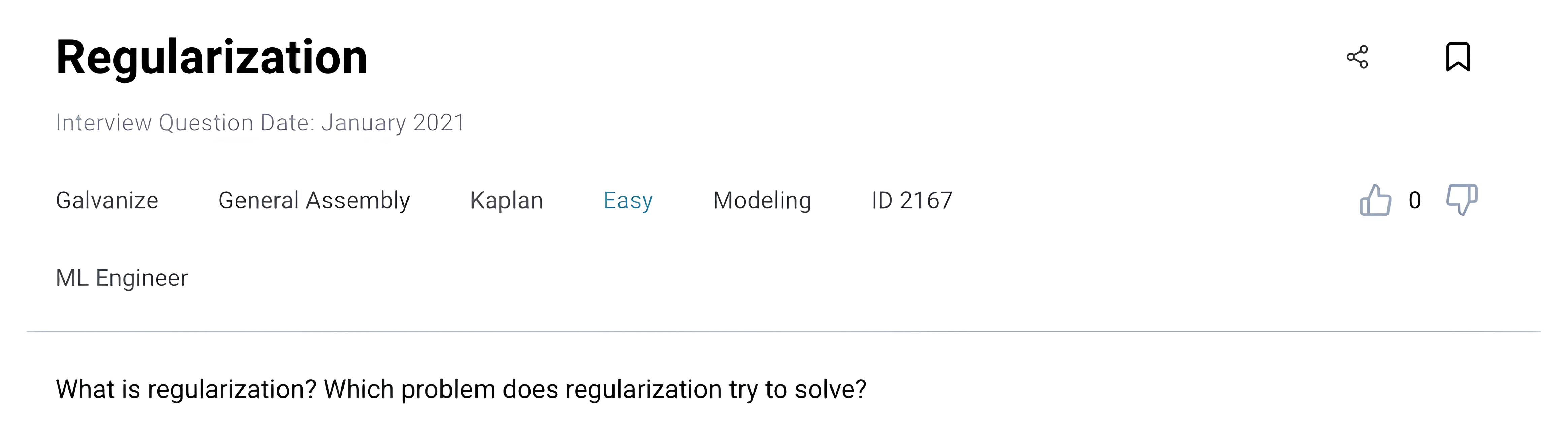
Link to this question: https://platform.stratascratch.com/technical/2167-regularization
Think of it as a penalty for complexity. If your model is too complicated, it might not work well on new data. Regularization helps by adding a term that discourages complexity.
Xgboost & Random Forest
This question was asked in the Machine Learning Engineer interview by Capital One.
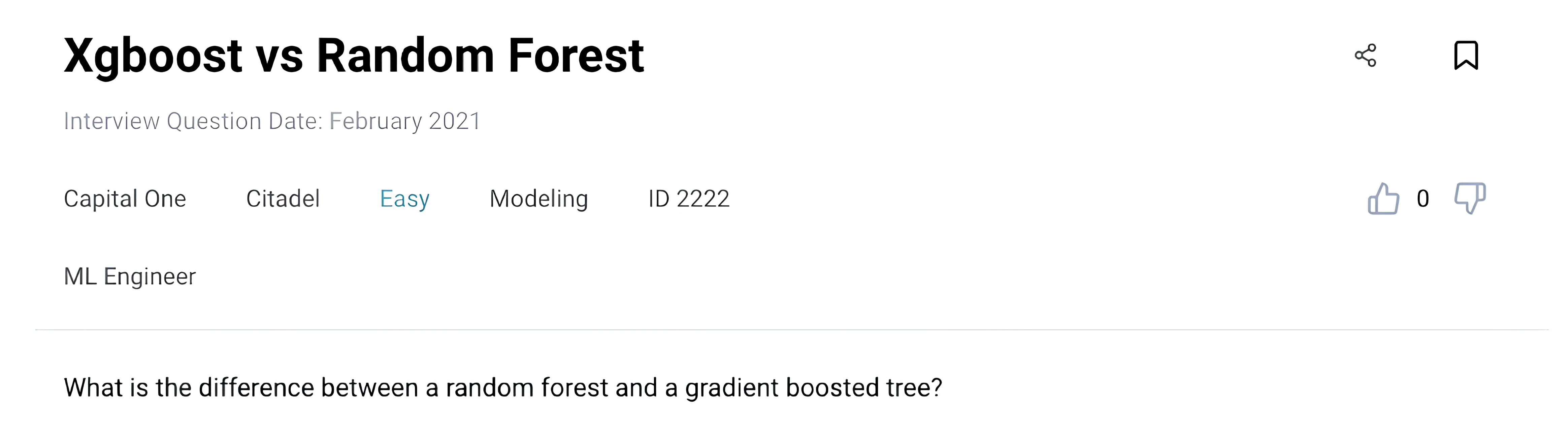
Link to this question: https://platform.stratascratch.com/technical/2222-xgboost-vs-random-forest
Random Forest builds many trees and averages their predictions. Gradient Boosted Trees build one tree at a time, learning from the mistakes of the previous ones. Both are good but used for different needs.
Regression and Classification Problem
This question was asked in the Machine Learning Engineer interview by Galvanize.
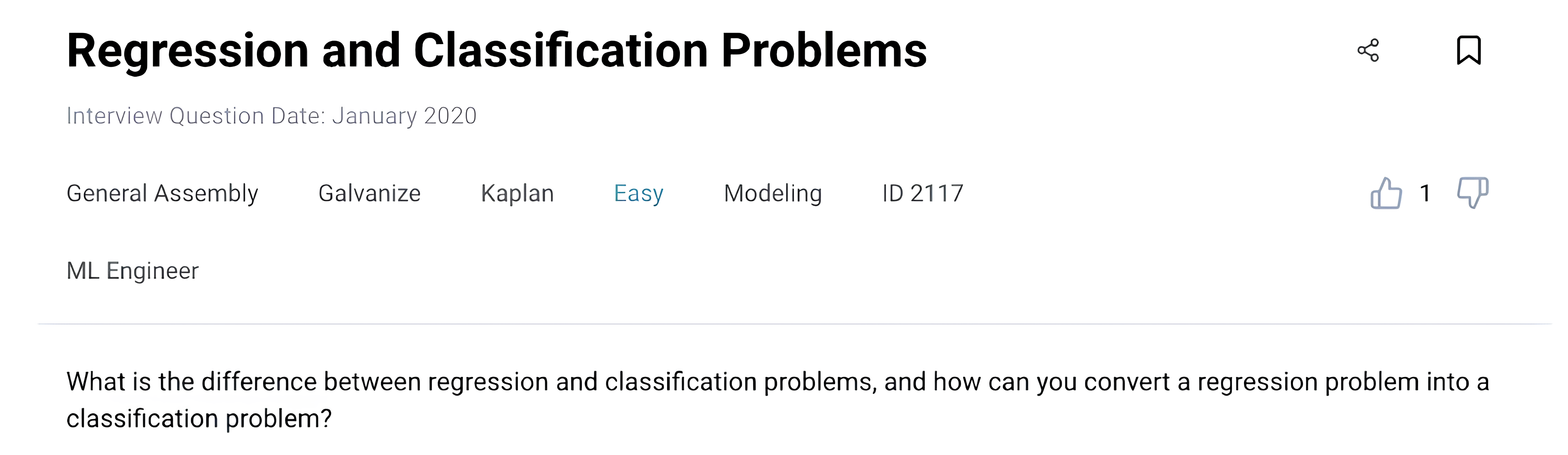
Link to this question : https://platform.stratascratch.com/technical/2117-regression-and-classification-problems
Regression predicts a number (like house price), while Classification puts data into categories (like spam or not-spam). To convert a regression problem into classification, you can divide the numerical output into ranges (like 'low', 'medium', 'high').
AI Scenario-Based Interview Questions
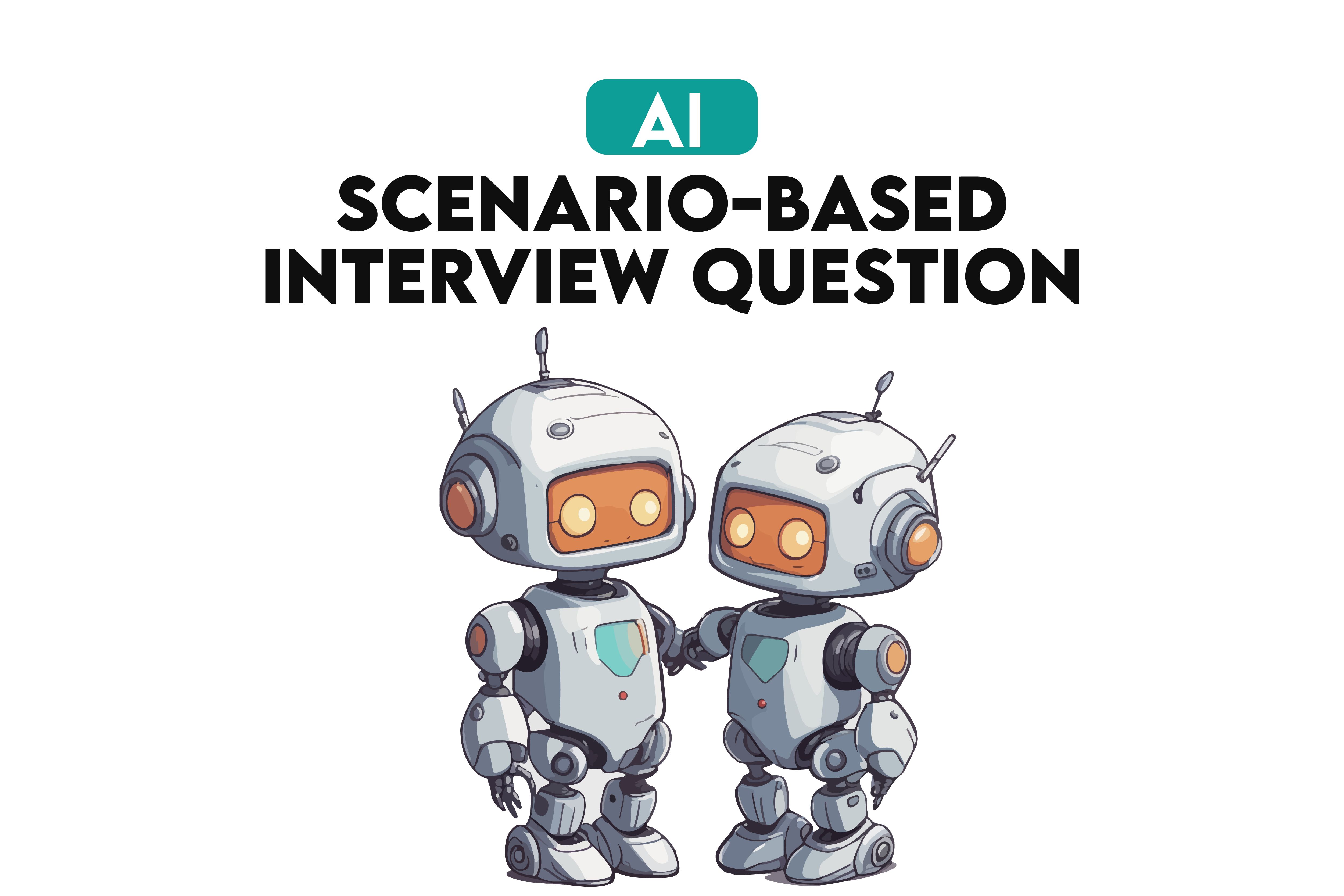
These AI interview questions place you in hypothetical, but plausible, AI scenarios. You may be asked to design an AI solution for a given problem, troubleshoot a failing model, or optimize an existing algorithm.
The goal here is to evaluate your problem-solving skills in real-world conditions and assess your practical understanding of AI technology. Each of these categories provides a unique angle on your AI skills and prepares you for the different kinds of challenges you'll face in your career.
Imbalanced Data Issue
This question was asked in the Machine Learning Engineer interview by MAANA.
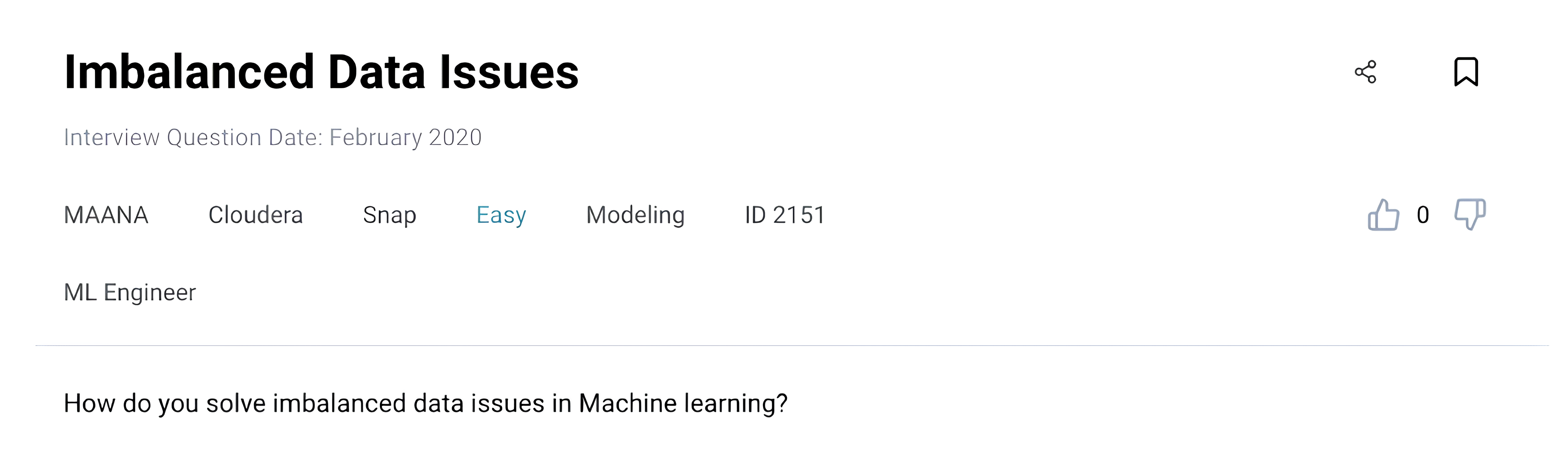
Link to this question: https://platform.stratascratch.com/technical/2151-imbalanced-data-issues
You can either collect more data for the minority class, use different metrics like precision or recall, or use techniques like SMOTE to balance the classes.
Predictive Model
This question was asked in the Machine Learning Engineer interview by Zillow.
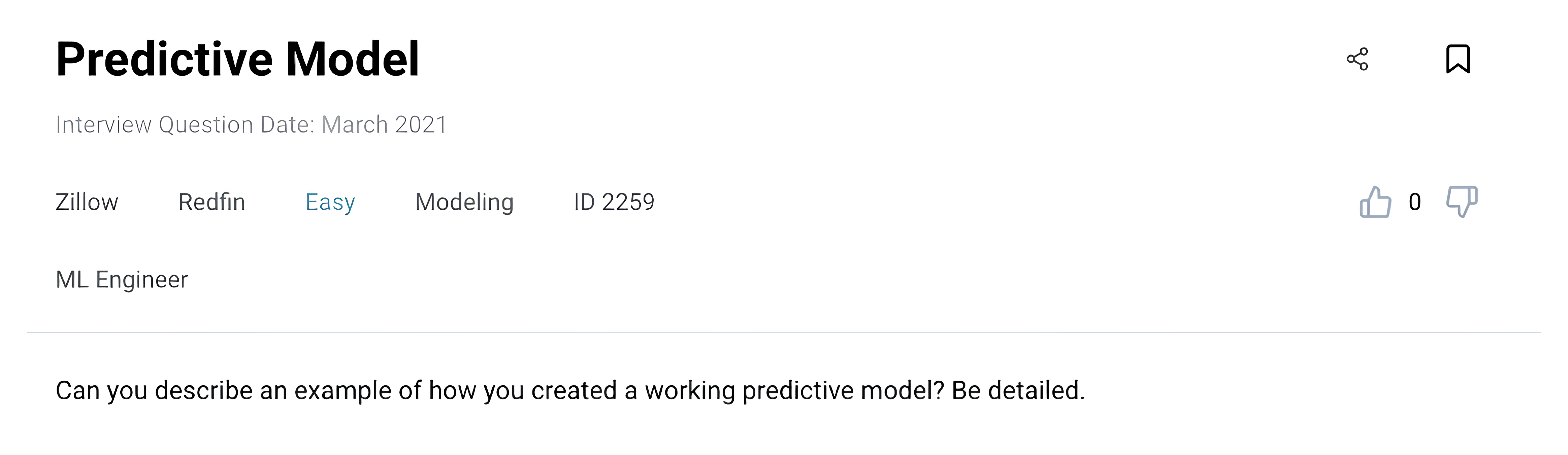
Link to this question: https://platform.stratascratch.com/technical/2259-predictive-model
- Step 1: Data Collection: Gathered historical sales data for an e-commerce platform.
- Step 2: Data Cleaning: Removed outliers and filled missing values.
- Step 3: Feature Selection: Choose variables like user activity, seasonality, and product ratings.
- Step 4: Model Selection: Opted for a linear regression model.
- Step 5: Training: Used 70% of the data to train the model.
- Step 6: Validation: Applied cross-validation to assess the model's performance.
- Step 7: Testing: Used the remaining 30% of data to test the model.
- Step 8: Evaluation: Achieved an R-squared value of 0.8, indicating a good fit.
- Step 9: Deployment: Integrated the model into the platform for real-time sales prediction.
Overfitting Problem
This AI interview question was asked in the Machine Learning Engineer by Capital One.
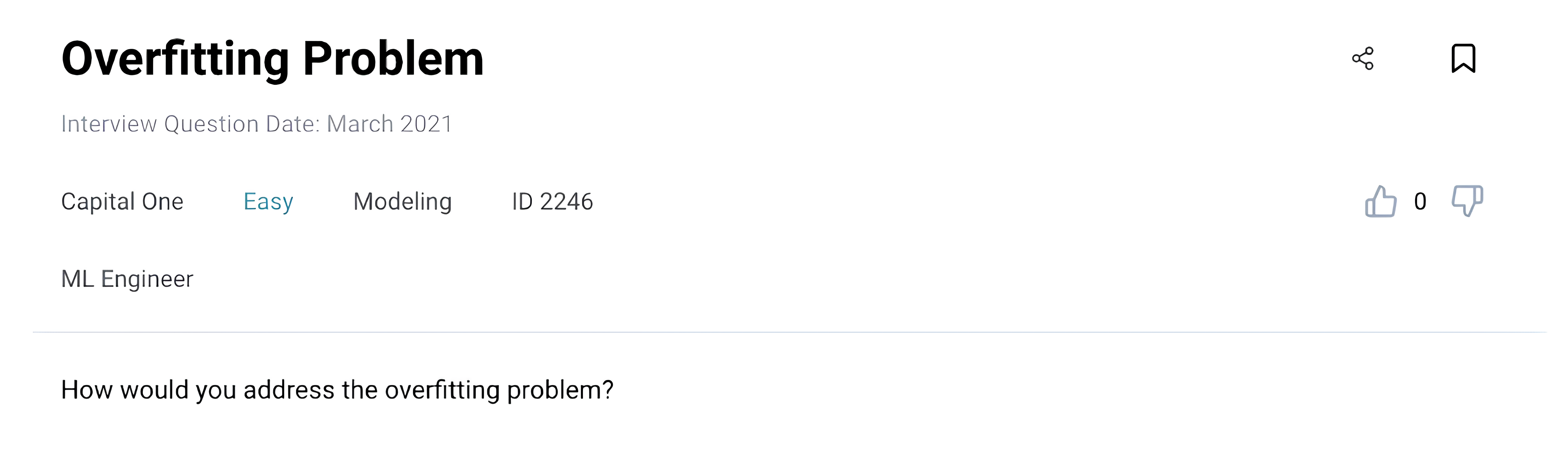
Link to this question: https://platform.stratascratch.com/technical/2246-overfitting-problem
To tackle overfitting, you can simplify the model, use more data, or apply techniques like cross-validation.
Machine Learning Model
This question was asked in a Machine Learning Engineer interview by the General Assembly.
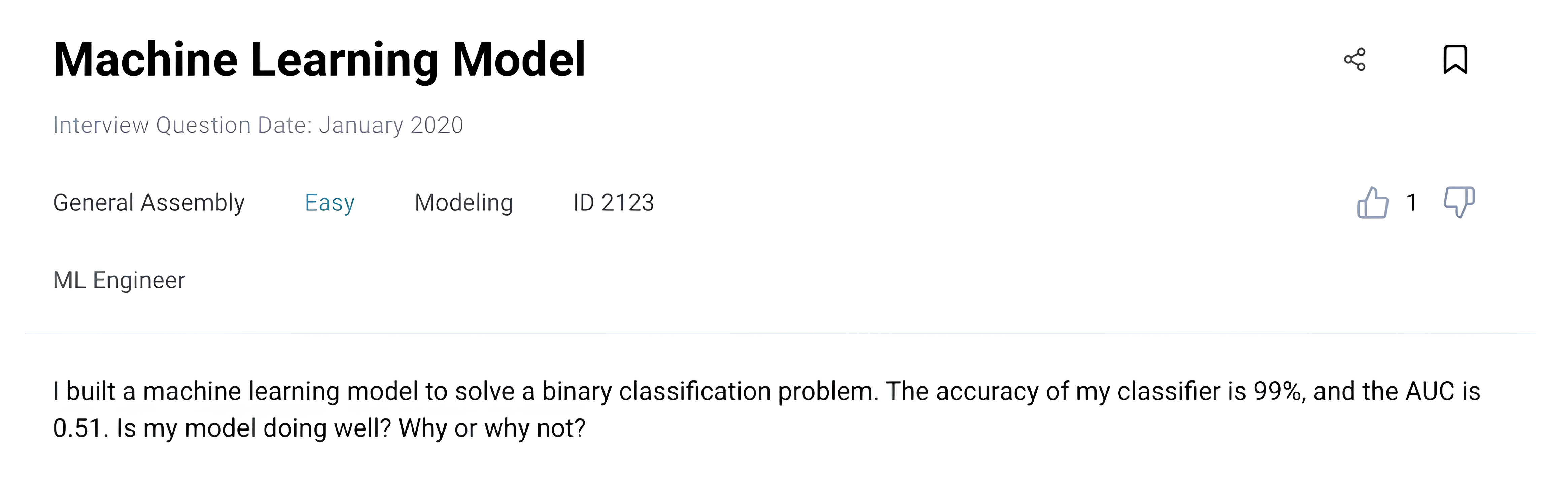
Link to this article: https://platform.stratascratch.com/technical/2123-machine-learning-model
Your model's high accuracy but low AUC suggests a problem. The likely reasons could be:
- Imbalanced Data: Your model might be biased towards the majority class.
- Wrong Metrics: Accuracy is not always the best metric, especially for imbalanced data.
- Overfitting: The model may have memorized the training data.
Solutions
- Use different metrics like F1-score or precision-recall curves.
- Balance the dataset.
- Simplify the model or use regularization.
Identify Peak Points
This question was asked in a Machine Learning Engineer interview by Square.
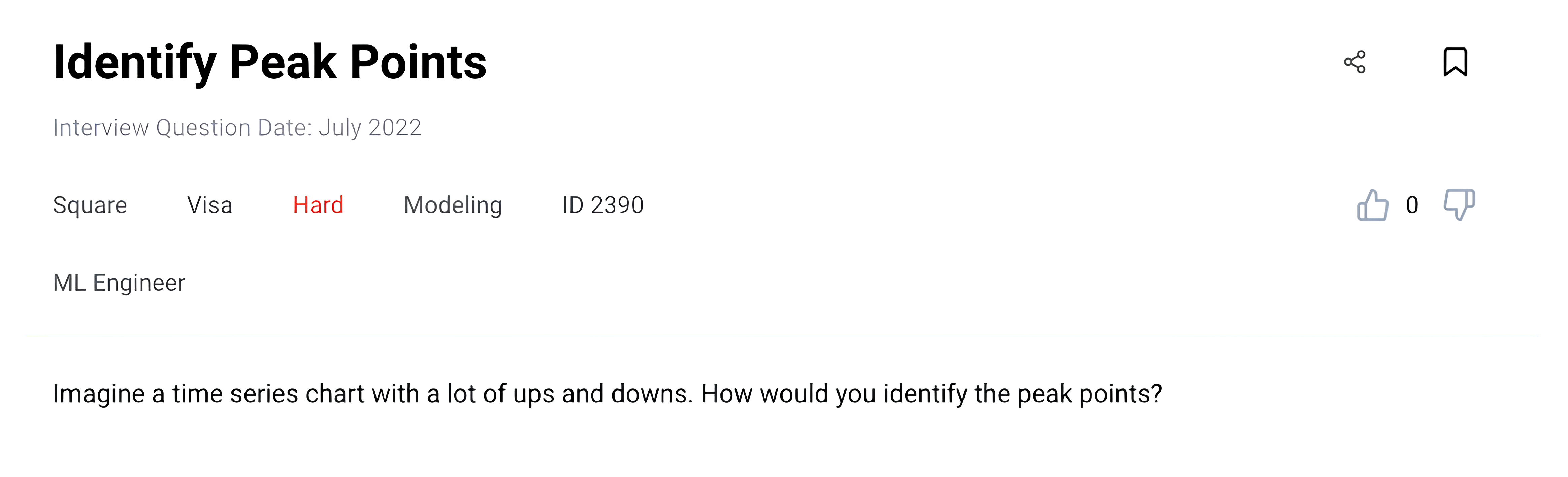
Link to this question: https://platform.stratascratch.com/technical/2390-identify-peak-points
You can use methods like moving averages to smooth the data and then identify points where the curve changes from rising to falling as peak points.
You might notice, there are a lot of Machine Learning engineer questions in our platform, if you want to see more of them, here is the article for you → Machine Learning Engineer Interview Questions.
Tips for Success
Cracking AI interviews requires more than just bookish knowledge; it demands practical experience and a bit of problem-solving. One of the best ways to prepare is through regular practice.
You will concrete your understanding, by doing practice. It helps you learn the nuances of code, and algorithms, and how to optimize them.
Another important factor of success is speed, because time is often limited in interviews. The more you practice, you will become faster.
Last but not least, you will gain confidence, once you crack a bunch of interview questions, because that will be living proof that you might actually be successful in an interview.
And also, if you get bored while working, here are the real-world ai projects article, that will not only help you,they are also interesting too.
Final Thoughts
In this article, you saw different kinds of AI Interview questions. But remember, to solidify your knowledge, one thing you must do is practice!
For a comprehensive practice regimen, consider using our platform. It offers a wide range of real-world AI interview questions across all categories that we mentioned, from FAANG companies and more.
Practicing on StrataScratch will not only prepare you for the interviews but also refine your skills and boost your confidence. See you there!
FAQ
How do I prepare for an AI interview?
Study core concepts like machine learning algorithms, neural networks, and data preprocessing. Practice coding challenges and review case studies related to AI applications.
What kind of questions do you ask AI?
Questions can range from technical aspects like "Explain backpropagation in neural networks" to ethical considerations like "How can AI be biased?".
What are the 4 basic concepts of AI?
The four basic concepts are machine learning, natural language processing, robotics, and computer vision.
Can AI help with interview questions?
Yes, AI can generate practice questions, provide answers for study, and even simulate interview scenarios to help you prepare.
Share