How to Use Python Property Decorator (With Examples)
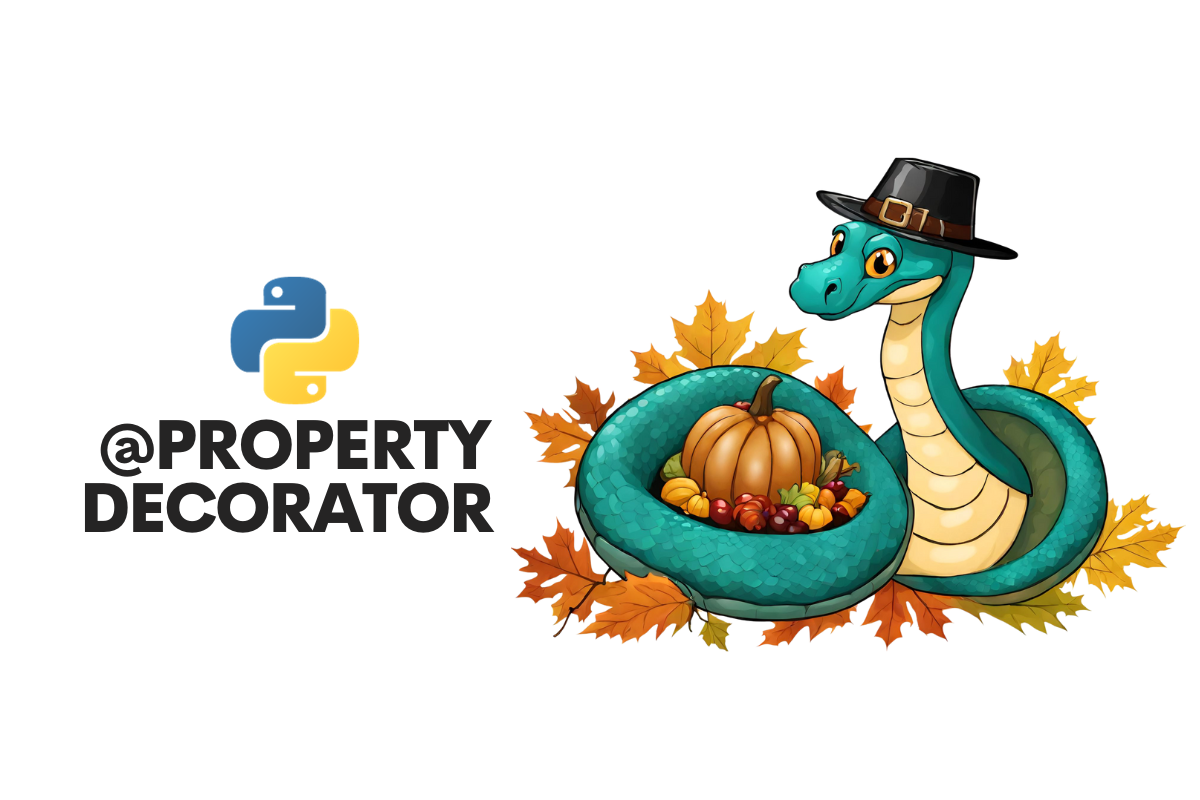
Categories
Master Python Property Decorators: From Basic Syntax to Advanced Techniques and Best Practices
You've probably noticed that the best Python code you've read seems clean, efficient, and easy to understand. Like a well-directed movie, every element has a purpose, and there are no extraneous details. One secret ingredient to go there is Python's property decorator!
So, what are this Python decorators? Well, I won't spill all the beans just yet. It's a tool that can help you write better, more maintainable code—making you look like a pro even if you're just starting out!
Buckle up, because we've got a great journey ahead! Our roadmap includes a deep dive into decorators, mastering the @property syntax, and uncovering its real-world applications. We'll also go into different practice, all while keeping our focus on how these concepts are invaluable for data science. So, ready to take your Python skills to the next level? Let's get started!
What are Decorators?
Decorators in Python are like special badges you give to functions to add extra powers without changing the function itself. Think of them as add-ons. In Data Science, you might use a decorator to measure how long a machine learning model takes to train.
So, how does this work? Let’s say you have a function, and you want to add some feature to it, like logging or timing. Instead of rewriting the function, you wrap it with a decorator.
Let's jump into an example. Say you're a data scientist and you want to know how long your data preprocessing takes.
import time
def timing_decorator(func):
def wrapper(*args, **kwargs):
start_time = time.time()
result = func(*args, **kwargs)
end_time = time.time()
print(f"{func.__name__} took {end_time - start_time} seconds to run.")
return result
return wrapper
@timing_decorator
def data_preprocessing(data):
# Some heavy computation here
processed = [x*2 for x in data]
return processed_data
# Using the decorated function
data = [1, 2, 3, 4]
processed = data_preprocessing(data)
Here is the output.

Here, the @timing_decorator tells Python to use timing_decorator to modify data_preprocessing. When you run data_preprocessing, it will also print out how long it took to run. This is super useful in Data Science to help you understand the efficiency of your code.
Understanding Properties in Python
In Python, properties allow you to change how you access an object's attributes. It is a gateway that controls both the input and output of data. In the world of Data Science, you could use properties to validate if the data being input into a machine learning model meets certain conditions.
Alright, let's get into it. Imagine you're building a data science model that requires the temperature to be in a certain range for optimal performance. You can use Python properties to do just that.
class TemperatureModel:
def __init__(self, initial_temperature):
self._temperature = initial_temperature
@property
def temperature(self):
return self._temperature
@temperature.setter
def temperature(self, value):
if 10 <= value <= 40:
self._temperature = value
else:
print("Temperature out of range. Please set between 10 and 40.")
# Create a model with initial temperature 20
model = TemperatureModel(20)
print(model.temperature)
# Try to set the temperature to 5
model.temperature = 5
Here is the output.

In this code, the Python property decorator makes it so that you can't just randomly set the temperature to any value. It has to be between 10 and 40 for the model to work properly. This is crucial in Data Science, where the quality of your input can seriously impact your results.
What is the Python @property Decorator?
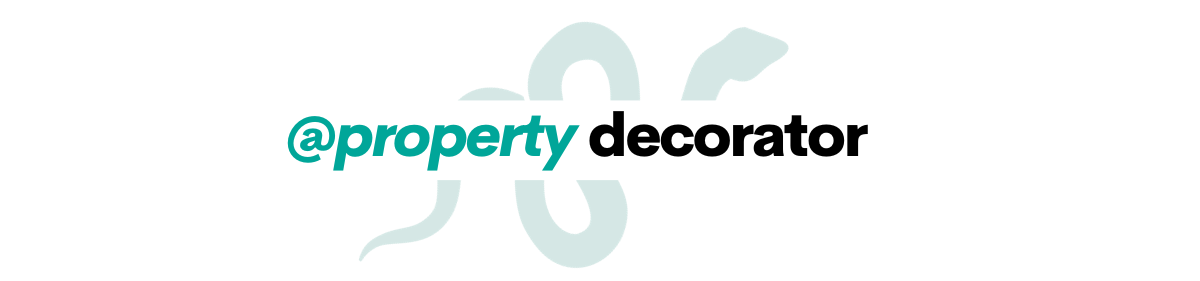
The Python property decorator makes attributes in a class act like read-only properties. Essentially, it lets you access methods as if they were attributes, without needing to write parentheses. In Data Science, you could use @property to create a read-only attribute for, say, the precision score of a classification model.
Let's get into it! Suppose you're working on a machine learning model for predicting stock prices. You have a Python class for the model, and you want to expose the model's accuracy without allowing it to be changed from outside the class.
class StockPredictor:
def __init__(self):
self._accuracy = 0.95
@property
def accuracy(self):
return self._accuracy
# Create a StockPredictor object
predictor = StockPredictor()
# Access the accuracy property
print(predictor.accuracy)
# Try to modify the accuracy property
# predictor.accuracy = 0.96
In this code, we used the Python property decorator on the accuracy method. This turns the method into a "property" that you can access like an attribute. You get the model's accuracy by just saying predictor.accuracy, and you can't change it, which is good for making sure your model's accuracy remains unaltered.
Here you can test if it can be changed.
predictor.accuracy = 0.96
Here is the output.

This is important in Data Science because sometimes attributes like accuracy are critical and should not be changed accidentally.
Implementing Setters with @property Decorator in Python
While the property decorator in Python is commonly used for read-only attributes, Python also allows you to define setters for those properties. A setter allows you to customize how a property is modified. Imagine you're working on a data science project where you're dealing with temperature data.
Using setters, you can automatically convert any temperature data to Fahrenheit when it's set.
Let's dive right in. For our data science project, suppose we want to store temperatures, but we want to ensure that all temperatures are stored in Fahrenheit.
class Temperature:
def __init__(self, temp_f):
self._temp_f = temp_f
@property
def temp_f(self):
return self._temp_f
@temp_f.setter
def temp_f(self, value):
if value < -459.67:
print("Temperature below absolute zero is not possible.")
else:
self._temp_f = value
Here's how it works:
- We define a Temperature class with an initial temperature in Fahrenheit.
- We create a @property called temp_f to get the temperature.
- We then use @temp_f.setter to create a setter for temp_f.
The setter allows us to apply a rule. In this case, the rule is that temperatures below -459.67 Fahrenheit (absolute zero) are not allowed. If you try to set a temperature below this value, the program will print a warning.
# Example
my_temp = Temperature(32)
print(my_temp.temp_f)
Here is the output.

But what if we try below -459.67? Let’s see.
my_temp.temp_f = -500
Here is the output.

So, what's the big deal in Data Science? Well, setters ensure data integrity. For example, in this case, we can be confident that any temperature data in the Temperature class will always be above absolute zero, ensuring more reliable results in any subsequent data analysis.
Handling Deletion with @property Decorator in Python
The Python @property decorator isn't just for getting and setting attributes. It also allows you to manage attribute deletion with a deleter. Let's say you're dealing with a data science project that involves patient medical records.
Deleting a record should only occur under certain conditions to maintain data integrity. Let's see it in action. In our example, we'll create a simple Patient class that only allows a record to be deleted if no treatment is ongoing.
class Patient:
def __init__(self, name, undergoing_treatment=False):
self.name = name
self._undergoing_treatment = undergoing_treatment
@property
def undergoing_treatment(self):
return self._undergoing_treatment
@undergoing_treatment.deleter
def undergoing_treatment(self):
if self._undergoing_treatment:
print("Cannot delete: Patient is undergoing treatment.")
else:
print(f"Patient {self.name}'s record deleted.")
del self._undergoing_treatment
Here's what we did:
- Initialized a Patient class with a name and a treatment status.
- Used the Python property decorator for undergoing_treatment to get the status.
- Employed @undergoing_treatment.deleter to specify the conditions under which the attribute can be deleted.
In this example, the deletion is conditioned on whether the patient is undergoing treatment.
# Example
john = Patient('John', False)
print(john.undergoing_treatment)
Here is the output.

Here is another code.
del john.undergoing_treatment
Here is the output.

We can delete John, because it looks like earlier, we defined that he wont go under treatment.
Why is this useful in Data Science? Controlling how data is deleted is crucial for maintaining the integrity of your datasets. With property deleters, you can set up conditions that must be met for a piece of data to be removed, reducing the risk of accidental or inappropriate data deletion.
Real-world Applications of Python Property Decorator
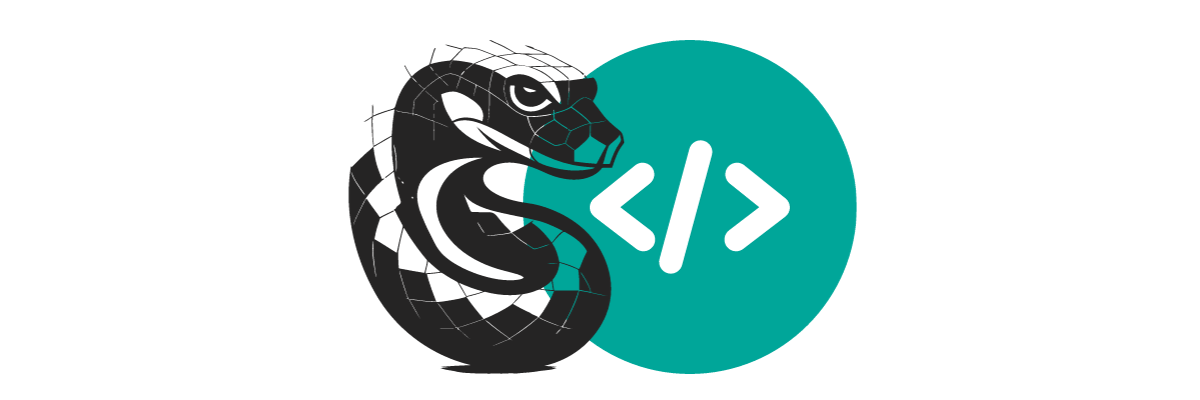
The Python @property decorator is not just a neat feature for academic exercises; it has numerous practical applications. Imagine you're a data scientist working with large datasets. How you manage, validate, and secure this data can be effectively handled by using the Python property decorator.
Managing Object States
Let's consider a machine learning model that trains on new data periodically. Here's how @property can be used to manage its training state:
class MLModel:
def __init__(self):
self._is_trained = False
@property
def is_trained(self):
return self._is_trained
def train(self, data):
# Simulate training
self._is_trained = True
print("Model trained.")
Let’s test it.
Here is the code.
model = MLModel()
print(model.is_trained)
Here is the output.

As you can see the model is not trained. Let’s train this model and test it again.
df = []
model.train(df)
print(model.is_trained)

This ensures that the training state of the model can be checked but not changed arbitrarily. This management is essential when handling dynamic datasets in data science.
Data Validation and Type Checking
When working with data, ensuring data type and validation is critical. Here's a Python example:
class DataFrame:
def __init__(self, data):
self._data = data
@property
def data(self):
return self._data
@data.setter
def data(self, value):
if not isinstance(value, list):
raise TypeError("Data should be a list.")
self._data = value
Let’s test it.
Here is the code, to create this dataframe.
df = DataFrame([1, 2, 3])
print(df.data)
Here is the output.

As you can see, it works. Let’s test it with different data types, if our initial code works or not.
df.data = "string"
Here is the error we got. It looks like our code works.
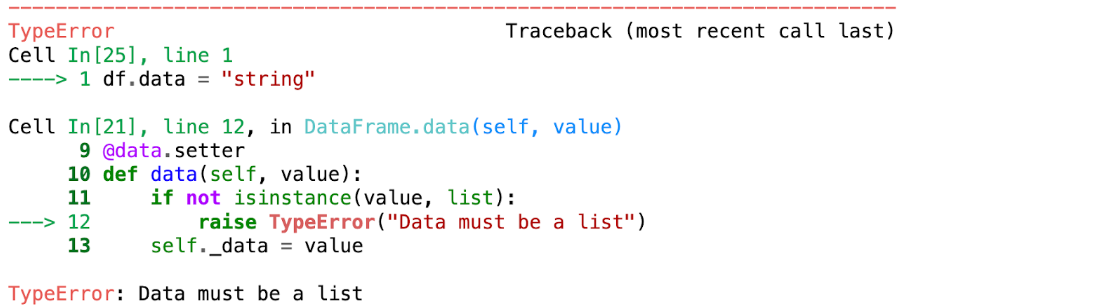
Creating Read-only Properties
Sometimes, you don't want certain attributes to be modified. The Python property decorator can make this happen.
class ImmutableData:
def __init__(self, data):
self._data = data
@property
def data(self):
return self._data
This makes data read-only, which is useful for ensuring the integrity of static datasets in data science.
Let’s test it, by creating data first and trying to change it afterwards.
im_data = ImmutableData([1, 2, 3])
print(im_data.data)
Here is the output.

Now, let’s try to change the data.
im_data = ImmutableData([3, 4, 5])
print(im_data.data)

Wonderful, our code makes this data immutable, so it can not be changed.
Enhancing Data Security in Applications
If you are working with sensitive data, controlling who can modify it is critical.
class SecureData:
def __init__(self, data, user):
self._data = data
self._user = user
@property
def data(self):
if self._user == 'admin':
return self._data
else:
return "Access Denied"
This approach enhances data security by restricting access to the data based on user roles.
Let’s test it.
# Test
secure_data = SecureData("Sensitive Info", 'user')
print(secure_data.data) # Output: "Access Denied"
Here is the output.

Let’s test it by roling our selves as an admin.
secure_data.user_role = 'admin'
print(secure_data.data)
Here is the output.

It works!
To sum up, the Python property decorator is a robust tool that offers various real-world applications, especially in managing, validating, and securing data in data science projects.
Common Pitfalls and Best Practices
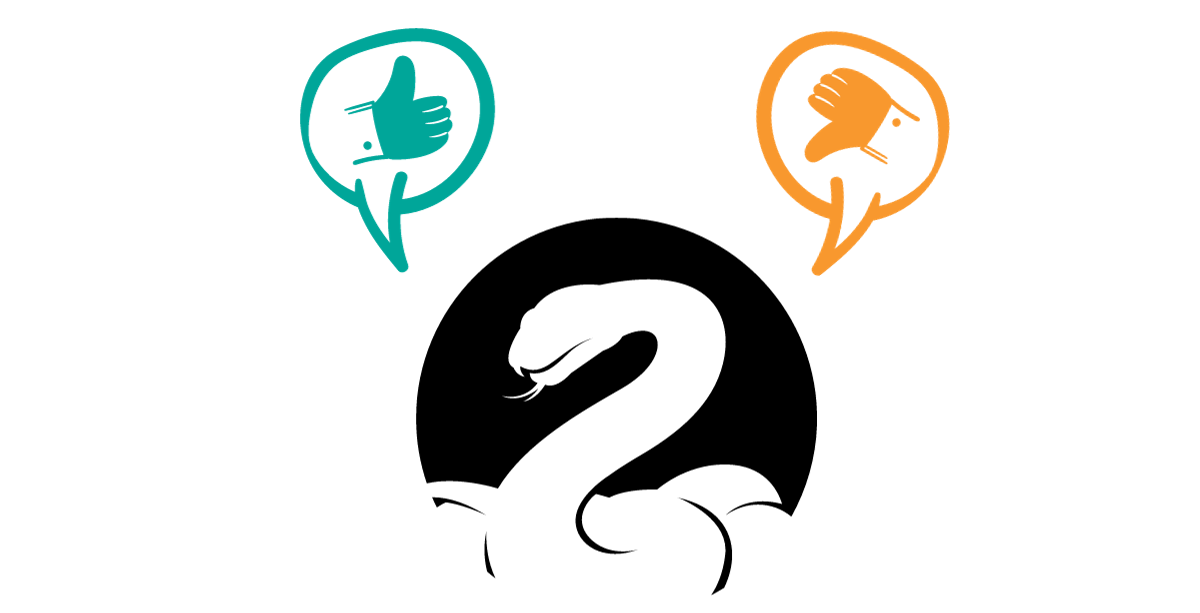
While Python's @property decorator can help manage data efficiently, especially in data science projects, it's crucial to be aware of potential pitfalls and follow best practices. For instance, a poorly implemented setter can disrupt the state of a machine learning model.
Potential Mistakes While Using @property
Sometimes, you might think you're validating data but actually aren't. Here's an example related to Data Science:
class BadModel:
def __init__(self):
self._is_trained = False
@property
def is_trained(self):
return self._is_trained
@is_trained.setter
def is_trained(self, value):
if type(value) is not bool:
self._is_trained = False # Wrongly setting to False
After running the code for the BadModel, you'll find that setting is_trained to a non-boolean value indeed sets it to False. This is a potential pitfall as it might unintentionally change the model's training state, leading to misleading results in your data science project.
Best Practices in Using Property Decorators
Always ensure to handle exceptions and errors gracefully. Here's how:
class GoodModel:
def __init__(self):
self._is_trained = False
@property
def is_trained(self):
return self._is_trained
@is_trained.setter
def is_trained(self, value):
if type(value) is not bool:
raise TypeError("Value must be a boolean")
By raising a TypeError, the code alerts you right away if you're doing something wrong, making your data science project more robust.
Testing and Documentation for Properties
It's crucial to add test cases for your properties. Take a look at this:
def test_good_model():
model = GoodModel()
assert model.is_trained == False
model.is_trained = True
assert model.is_trained == True
try:
model.is_trained = "yes" # Should raise a TypeError
except TypeError:
pass
After running the test_good_model() function, you should see that the test cases for the GoodModel pass as expected. This verifies that the property methods behave as intended, which is crucial for data validation and state management in data science.
Testing ensures that your property methods are doing exactly what you expect, which is especially important in complex data science tasks involving data validation and state management.
By being aware of these pitfalls and following best practices, you make your data science projects not only more efficient but also less prone to errors.
Conclusion
So, we disovered Python property decorators, from understanding the basics to diving into real-world applications. We even tackled common pitfalls and best practices to steer you clear of bumps in your data science journey.
Practice makes perfect, right? If you want to become a proficient data scientist, don't just read about Python property decorators—get your hands dirty with code. Run tests, build models, and see how these powerful Python features can streamline your workflow.
And here's some good news: you don't have to do it alone. Our platform is packed with data projects and Python interview questions tailored for data science. It's a sandbox for you to apply what you've learned and a ladder for you to climb higher in your data science career.
So what are you waiting for? See you there!
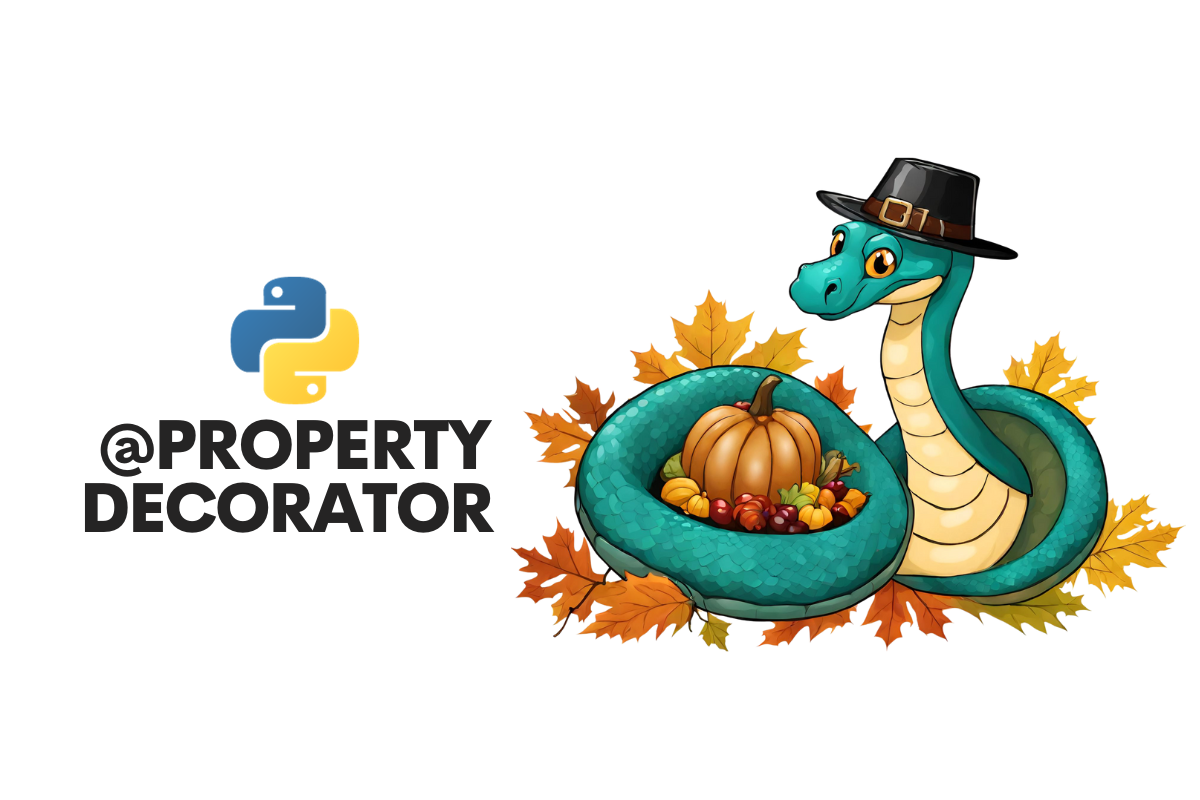