7 Magic Methods That Will Turn You Into a Python Wizard
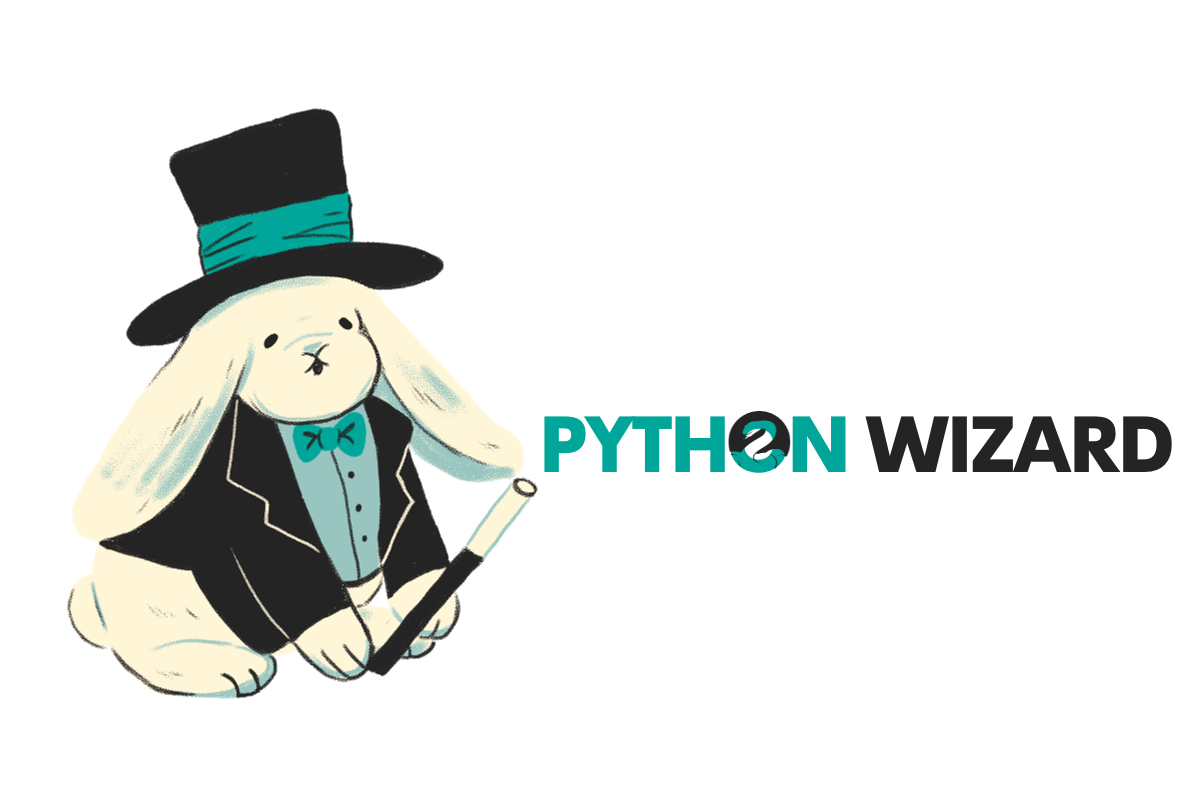
Categories
Unlock the power of Python magic methods to level up your programming skills. From the basic __init__to the advanced __getitem__.
Anyone familiar with Data Science will confirm that Python is the most popular and preferred programming language in data science. This is also supported by many researches, like this one.

Due to its vast features, expanding from web scraping to model building, mastering it might take time. As you gain experience, you might want to operate high-level operations, like defining classes and internal manipulation. This is where Python magic methods come into play.
In this article, you will explore some of these Python magic methods, starting with the __len__ method and extending to the __getitem__ method. You can detect magic methods, by looking at the double underscores at the beginning and end. “__”.
Magic Methods in Python
Before we go into the Python magic methods further, it's worth mentioning how many magic methods exist in Python. These methods, which might called Python "dunder" methods due to their double underscores, are special in that they allow us to define how our custom objects should behave in different situations, which we will cover later in this article.
To check how many magic methods are available in Python for a particular type, you can use the dir() function, which lists all attributes of an object, including its magic methods. Let’s see.
print(dir(int))
Here is the output, that contains Python magic methods.

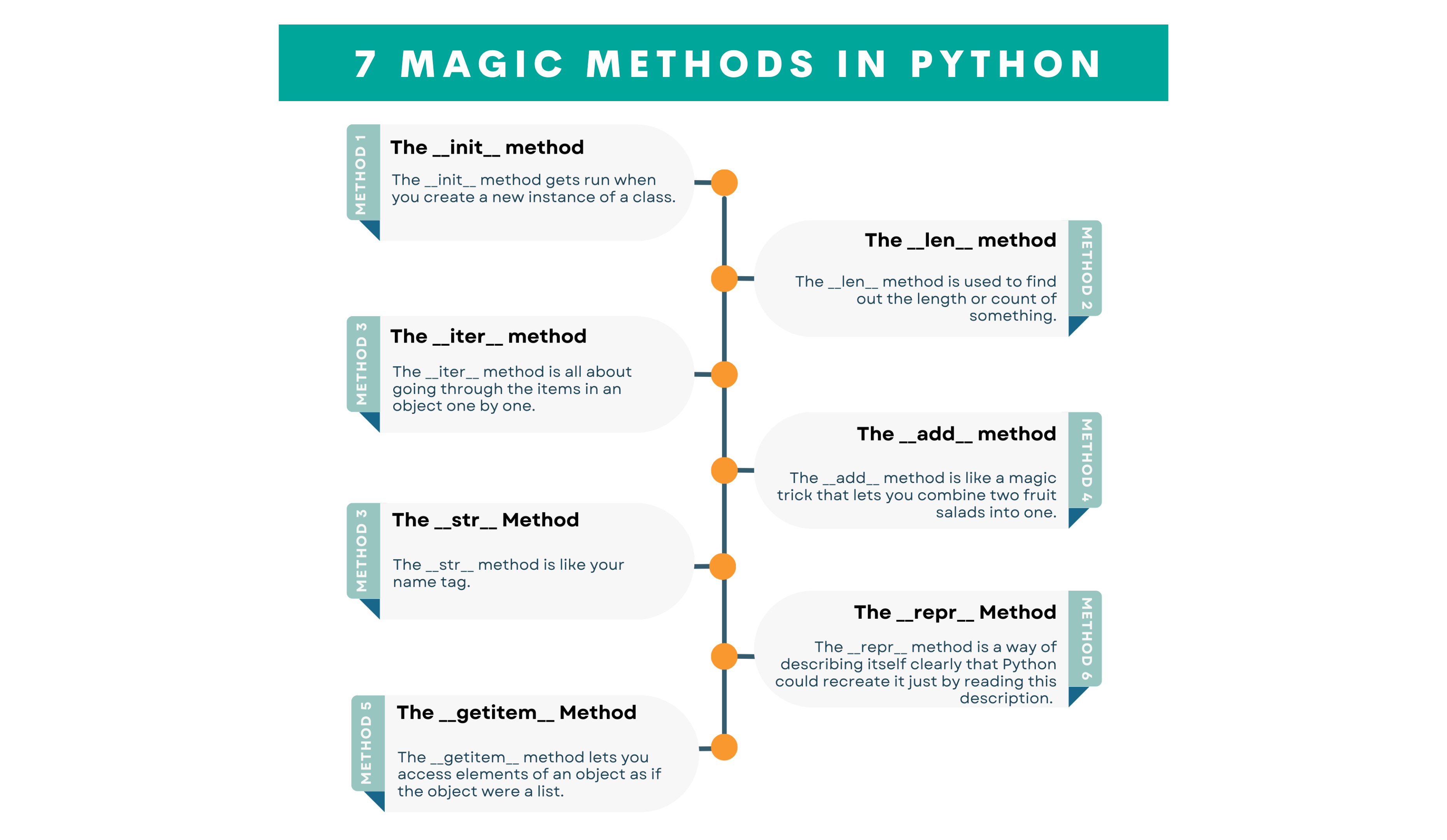
Great, now you know all the Python magic methods, let’s discover 7 of them, by starting with __init__ method.
Python Magic Method #1: The __init__ method
The __init__ method gets run when you create a new instance of a class.
Here we will define a class named FruitSalad. Think of a class as a blueprint for making objects, like a recipe. Objects are instances made using that blueprint.
Inside the class, there's a function called __init__. This function sets up each new object when it's created. It needs some initial info like size and fruits to set up the object.
When you do my_salad = FruitSalad('large', ['apple', 'banana', 'cherry']), you're making a new object using the FruitSalad blueprint. The __init__ function jumps in and sets the size to 'large' and fruits to ['apple', 'banana', 'cherry'].
Finally, print(my_salad.size) and print(my_salad.fruits) show what size and fruits got saved as when we made my_salad.
So, the __init__ function is like the starting point that sets up each new FruitSalad object with its own size and fruits.
Let’s see the code.
class FruitSalad:
# The __init__ method initializes the object
def __init__(self, size, fruits):
self.size = size
self.fruits = fruits
# Create a new FruitSalad instance
my_salad = FruitSalad('large', ['apple', 'banana', 'cherry'])
# Access the attributes
print(my_salad.size)
print(my_salad.fruits)
Here is the output.

Python Magic Method #2: The __len__ method
The __len__ method is used to find out the length or count of something. In Python, when you call len() on an object, Python looks for a __len__ method inside that object's class to get the answer.
When you have a FruitSalad object and you want to know how many fruits are in it, the __len__ method comes to the rescue. Just like __init__ sets up the object, __len__ gives us the count of fruits in the salad.
When you use len(my_salad), Python automatically runs the __len__ method inside the FruitSalad class. This Python magic method returns the count of items in self.fruits, which is the list of fruits you provided when creating the salad.
In this case, the print statement shows '3', which tells you that there are three fruits in my_salad.
So, the __len__ method helps you quickly find out how many fruits are in your FruitSalad.
Let’s see the code.
class FruitSalad:
def __init__(self, size, fruits):
self.size = size
self.fruits = fruits
def __len__(self):
return len(self.fruits)
# Let's create a salad
my_salad = FruitSalad('large', ['apple', 'banana', 'cherry'])
print(len(my_salad))
Let’s see the output.

Python Magic Method #3: The __iter__ method
The __iter__ method is all about going through the items in an object one by one. When you want to look at each fruit in your FruitSalad, you can use a for loop. But for that to work, Python needs to know how to move from one fruit to the next. That's where __iter__ comes in.
When you use for fruit in my_salad, Python starts the __iter__ method. It sets self.n to zero as a starting point. Then the __next__ method takes over. It looks at self.fruits and gives you the next fruit on the list. Once it reaches the end, it stops and says there's no more.
The output will show each fruit, one by one: 'apple', 'banana', 'cherry'.
So, __iter__ lets you loop through the fruits in your FruitSalad easily.
Let’s see the code.
class FruitSalad:
def __init__(self, size, fruits):
self.size = size
self.fruits = fruits
def __iter__(self):
self.n = 0
return self
def __next__(self):
if self.n < len(self.fruits):
result = self.fruits[self.n]
self.n += 1
return result
else:
raise StopIteration
# Create a fruit salad
my_salad = FruitSalad('large', ['apple', 'banana', 'cherry'])
# Iterate over it
for fruit in my_salad:
print(fruit)
Here is the output.

Python Magic Method #4: The __add__ method
The __add__ method is like a magic trick that lets you combine two fruit salads into one. When you have two separate FruitSalads and you want to make a bigger one, you use the + symbol. It's as if you're adding them up, but instead, you're mixing the fruits from both.
So when you do mixed_salad = salad1 + salad2, Python calls the __add__ method. It checks if you're adding another FruitSalad. If yes, it takes the fruits from both salads and puts them in a new FruitSalad. Finally, it shows you the new list of fruits: ['apple', 'banana', 'cherry', 'orange'].
That's how __add__ lets you mix two FruitSalads into one.
Simple, right? Let’s see the code.
class FruitSalad:
def __init__(self, size, fruits):
self.size = size
self.fruits = fruits
def __add__(self, other):
if not isinstance(other, FruitSalad):
raise TypeError("You can only add another FruitSalad!")
new_fruits = self.fruits + other.fruits
return FruitSalad(self.size, new_fruits)
# Create two fruit salads
salad1 = FruitSalad('large', ['apple', 'banana'])
salad2 = FruitSalad('large', ['cherry', 'orange'])
# "Add" them
mixed_salad = salad1 + salad2
print(mixed_salad.fruits)
Here is the output.

Python Magic Method #5: The __str__ Method
The __str__ method is like your salad's name tag. It tells Python what to show when you want to print a FruitSalad object. This way, instead of seeing something confusing, you get a neat sentence describing your fruit salad.
When you run the code below, Python checks for a __str__ method in your class. It finds one and uses it to print "A large fruit salad with apple, banana, cherry."
So, the __str__ method helps us see our FruitSalad in a friendly, readable way. It's like asking your salad, "Who are you?" and the salad answers back.
Let’s see the code:
class FruitSalad:
def __init__(self, size, fruits):
self.size = size
self.fruits = fruits
def __str__(self):
return f"A {self.size} fruit salad with {', '.join(self.fruits)}."
# Create a fruit salad
salad = FruitSalad('large', ['apple', 'banana', 'cherry'])
print(salad)
Here is the output.

Python Magic Method #6: The __repr__ Method
The __repr__ method is a way for your fruit salad to describe itself so clearly that Python could recreate it just by reading this description. It's useful for developers to understand the object better, it is often used for debugging or development purposes.
In the following code, When you do print(repr(salad)), Python uses the __repr__ method to print out "FruitSalad(size='large', fruits=['apple', 'banana', 'cherry'])". This is like the salad saying, "Hey, this is exactly how you can make another salad just like me!"
So, the __repr__ method is for giving a detailed, clear description that could be used to make a new, identical fruit salad.
Here is the code.
class FruitSalad:
def __init__(self, size, fruits):
self.size = size
self.fruits = fruits
def __repr__(self):
return f"FruitSalad(size='{self.size}', fruits={self.fruits})"
# Create a fruit salad
salad = FruitSalad('large', ['apple', 'banana', 'cherry'])
print(repr(salad))
Here is the output.

Python Magic Method #7: The __getitem__ Method
The __getitem__ method lets you access the fruits in your salad as if the salad were a list. This method makes your object behave like a Python list when you try to get items from it using square brackets.
So, when you do print(salad[0]), Python looks for the first fruit in your salad, which is 'apple'. Similarly, print(salad[1]) will give you 'banana'. It's like asking, "What's the fruit at this position in my salad?"
So, the __getitem__ method allows you to pick fruits out of your salad by their position.
Let’s see the code.
class FruitSalad:
def __init__(self, size, fruits):
self.size = size
self.fruits = fruits
def __getitem__(self, index):
return self.fruits[index]
# Create a fruit salad
salad = FruitSalad('large', ['apple', 'banana', 'cherry'])
# Access by index
print(salad[0])
print(salad[1])
Here is the output.

Conclusion
In this article, we've shed light on the magical world of Python's magic methods, from the fundamental __init__ method to the more specialized __getitem__ method. These tools not only elevate your Python game but also unlock endless customization possibilities.
It might be for data manipulation or creating seamless, user-friendly interfaces. You've seen how magic methods make your classes behave like Python's built-in types, saving you time and making your code more intuitive.
If you're looking to truly become a Python wizard, understanding magic methods is an invaluable step. To dive even deeper, visit our platform, and try to solve interview questions, like these Top 30 Python interview questions and answers, to gain experience, and prepare for interviews at the same time!
See you there!
FAQ’s
What are magic methods in Python?
Magic methods in Python are special methods with double underscores (__) at the beginning and end of their names. They let you define how your custom objects behave in various situations, like addition or length checks.
What is the magic call method in Python?
The __call__ magic method in Python allows an object to be called like a function. This makes your object's instances callable, enhancing their behavior.
What is an example of a magic method?
An example of a magic method is __init__, which initializes new instances of a class. When you create an object, Python automatically calls the __init__ method to set it up.
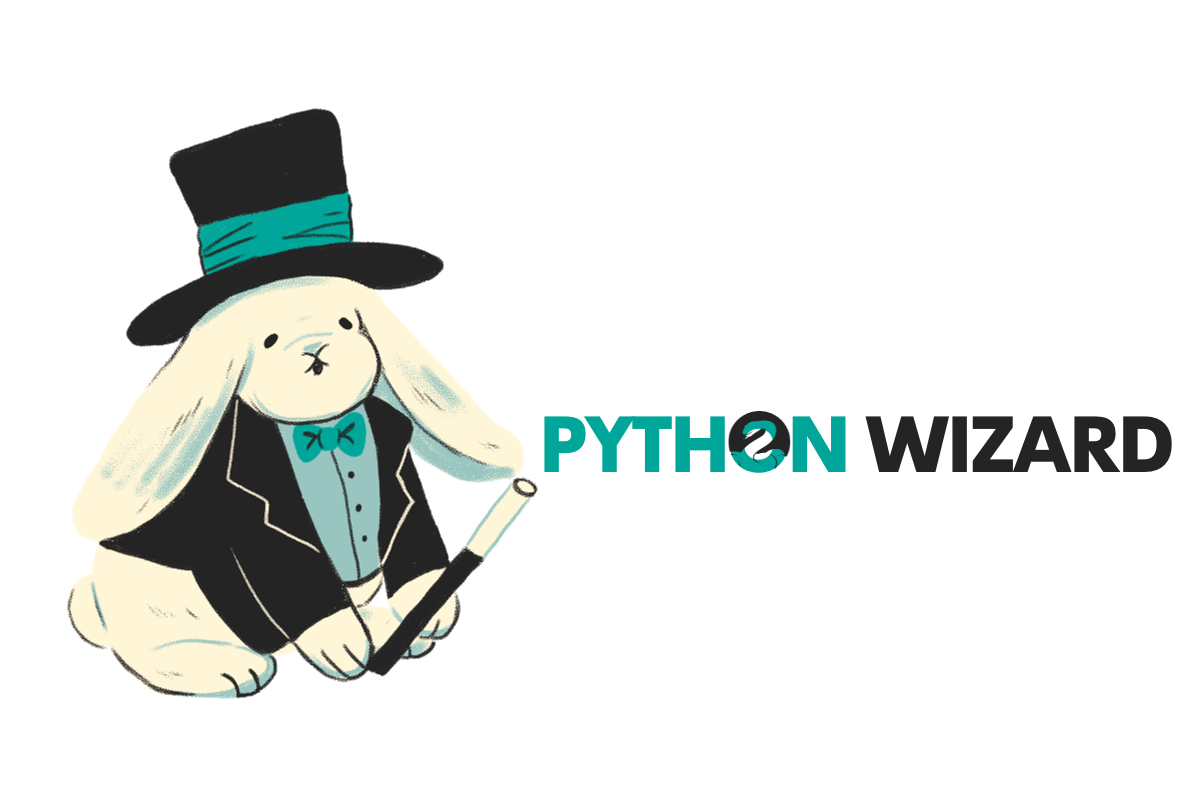